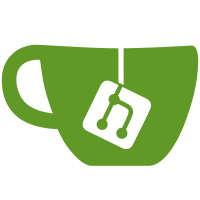
12 changed files with 28875 additions and 139 deletions
@ -1,114 +1,285 @@
|
||||
const jQueryBridget = require( 'jquery-bridget' ); |
||||
const Isotope = require( 'isotope-layout' ); |
||||
const jQueryBridget = require('jquery-bridget'); |
||||
const Isotope = require('isotope-layout'); |
||||
|
||||
export default { |
||||
init() { |
||||
// JavaScript to be fired on the catalog page
|
||||
jQuery( $ => { |
||||
jQueryBridget( 'isotope', Isotope, $ ); |
||||
let $grid = $( '.books' ); |
||||
$grid.isotope( { |
||||
(function() { |
||||
// Get all the <h2> headings
|
||||
const headings = document.querySelectorAll('fieldset h2'); |
||||
|
||||
Array.prototype.forEach.call(headings, heading => { |
||||
// Give each <h3> a toggle button child
|
||||
heading.innerHTML = ` |
||||
<button type="button" aria-expanded="false"> |
||||
${heading.textContent} |
||||
<svg aria-hidden="true" focusable="false" class="arrow" width="13" height="8" viewBox="0 0 13 8" xmlns="http://www.w3.org/2000/svg"><path d="M6.255 8L0 0h12.51z" fill="currentColor" fill-rule="evenodd"></path></svg> |
||||
</button> |
||||
`;
|
||||
|
||||
// Function to create a node list
|
||||
// of the content between this <h2> and the next
|
||||
const getContent = elem => { |
||||
let elems = []; |
||||
while ( |
||||
elem.nextElementSibling && |
||||
elem.nextElementSibling.tagName !== 'H2' |
||||
) { |
||||
elems.push(elem.nextElementSibling); |
||||
elem = elem.nextElementSibling; |
||||
} |
||||
|
||||
// Delete the old versions of the content nodes
|
||||
elems.forEach(node => { |
||||
node.parentNode.removeChild(node); |
||||
}); |
||||
|
||||
return elems; |
||||
}; |
||||
|
||||
// Assign the contents to be expanded/collapsed (array)
|
||||
let contents = getContent(heading); |
||||
|
||||
// Create a wrapper element for `contents` and hide it
|
||||
let wrapper = document.createElement('div'); |
||||
wrapper.hidden = true; |
||||
|
||||
// Add each element of `contents` to `wrapper`
|
||||
contents.forEach(node => { |
||||
wrapper.appendChild(node); |
||||
}); |
||||
|
||||
// Add the wrapped content back into the DOM
|
||||
// after the heading
|
||||
heading.parentNode.insertBefore(wrapper, heading.nextElementSibling); |
||||
|
||||
// Assign the button
|
||||
let btn = heading.querySelector('button'); |
||||
|
||||
btn.onclick = () => { |
||||
// Cast the state as a boolean
|
||||
let expanded = btn.getAttribute('aria-expanded') === 'true' || false; |
||||
|
||||
// Switch the state
|
||||
btn.setAttribute('aria-expanded', !expanded); |
||||
// Switch the content's visibility
|
||||
wrapper.hidden = expanded; |
||||
}; |
||||
}); |
||||
})(); |
||||
|
||||
(function() { |
||||
// Get all the <h3> headings
|
||||
const headings = document.querySelectorAll('fieldset h3'); |
||||
|
||||
Array.prototype.forEach.call(headings, heading => { |
||||
// Give each <h3> a toggle button child
|
||||
heading.innerHTML = ` |
||||
<button type="button" aria-expanded="false"> |
||||
${heading.textContent} |
||||
<svg class="arrow" width="13" height="8" viewBox="0 0 13 8" xmlns="http://www.w3.org/2000/svg"><path d="M6.255 8L0 0h12.51z" fill="currentColor" fill-rule="evenodd"></path></svg> |
||||
</button> |
||||
`;
|
||||
|
||||
// Function to create a node list
|
||||
// of the content between this <h2> and the next
|
||||
const getContent = elem => { |
||||
let elems = []; |
||||
while ( |
||||
elem.nextElementSibling && |
||||
elem.nextElementSibling.tagName !== 'H3' |
||||
) { |
||||
elems.push(elem.nextElementSibling); |
||||
elem = elem.nextElementSibling; |
||||
} |
||||
|
||||
// Delete the old versions of the content nodes
|
||||
elems.forEach(node => { |
||||
node.parentNode.removeChild(node); |
||||
}); |
||||
|
||||
return elems; |
||||
}; |
||||
|
||||
// Assign the contents to be expanded/collapsed (array)
|
||||
let contents = getContent(heading); |
||||
|
||||
// Create a wrapper element for `contents` and hide it
|
||||
let wrapper = document.createElement('div'); |
||||
wrapper.hidden = true; |
||||
|
||||
// Add each element of `contents` to `wrapper`
|
||||
contents.forEach(node => { |
||||
wrapper.appendChild(node); |
||||
}); |
||||
|
||||
// Add the wrapped content back into the DOM
|
||||
// after the heading
|
||||
heading.parentNode.insertBefore(wrapper, heading.nextElementSibling); |
||||
|
||||
// Assign the button
|
||||
let btn = heading.querySelector('button'); |
||||
|
||||
btn.onclick = () => { |
||||
// Cast the state as a boolean
|
||||
let expanded = btn.getAttribute('aria-expanded') === 'true' || false; |
||||
|
||||
// Switch the state
|
||||
btn.setAttribute('aria-expanded', !expanded); |
||||
// Switch the content's visibility
|
||||
wrapper.hidden = expanded; |
||||
}; |
||||
}); |
||||
})(); |
||||
|
||||
jQuery($ => { |
||||
jQueryBridget('isotope', Isotope, $); |
||||
let $grid = $('.books'); |
||||
$grid.isotope({ |
||||
itemSelector: '.book', |
||||
getSortData: { |
||||
title: '.title a', |
||||
title: '.book__title a', |
||||
subject: '[data-subject]', |
||||
latest: '[data-date-published]', |
||||
}, |
||||
sortAscending: { |
||||
title: true, |
||||
subject: true, |
||||
subject: false, |
||||
latest: false, |
||||
}, |
||||
} ); |
||||
$( '.filters > a' ).click( e => { |
||||
e.preventDefault(); |
||||
$( '.filters' ).toggleClass( 'is-active' ); |
||||
$( '.filter-groups > div' ).removeClass( 'is-active' ); |
||||
} ); |
||||
$( '.filter-groups .subjects > a' ).click( e => { |
||||
e.preventDefault(); |
||||
let id = $( e.currentTarget ).attr( 'href' ); |
||||
$( `.filter-groups .subjects:not(${id})` ).removeClass( 'is-active' ); |
||||
$( `.filter-groups ${id}` ).toggleClass( 'is-active' ); |
||||
} ); |
||||
$( '.licenses > a' ).click( e => { |
||||
e.preventDefault(); |
||||
let id = $( e.currentTarget ).attr( 'href' ); |
||||
$( id ).toggleClass( 'is-active' ); |
||||
} ); |
||||
$( '.subjects .filter-list a' ).click( e => { |
||||
e.preventDefault(); |
||||
if ( $( e.currentTarget ).hasClass( 'is-active' ) ) { |
||||
$( '.subjects .filter-list a' ).removeClass( 'is-active' ); |
||||
$( '.subjects' ).removeClass( 'has-active-child' ); |
||||
} else { |
||||
$( '.subjects .filter-list a' ).removeClass( 'is-active' ); |
||||
$( e.currentTarget ).addClass( 'is-active' ); |
||||
$( '.subjects' ).removeClass( 'has-active-child' ); |
||||
$( e.currentTarget ) |
||||
.parent() |
||||
.parent() |
||||
.parent( '.subjects' ) |
||||
.addClass( 'has-active-child' ); |
||||
} |
||||
let subjectValue = $( '.subjects .filter-list a.is-active' ).attr( |
||||
'data-filter' |
||||
); |
||||
let licenseValue = $( '.licenses .filter-list a.is-active' ).attr( |
||||
'data-filter' |
||||
); |
||||
if ( typeof licenseValue === 'undefined' ) { |
||||
licenseValue = ''; |
||||
} else { |
||||
licenseValue = `[data-license="${licenseValue}"]`; |
||||
}); |
||||
let licenses = document.querySelector('.license-filters'); |
||||
let subjects = document.querySelector('.subject-filters'); |
||||
let sorts = document.querySelector('.sorts'); |
||||
licenses.addEventListener('click', function(event) { |
||||
if (event.target.type !== 'radio') { |
||||
return; |
||||
} |
||||
if ( typeof subjectValue === 'undefined' ) { |
||||
subjectValue = ''; |
||||
} else { |
||||
subjectValue = `[data-subject="${subjectValue}"]`; |
||||
let license = ''; |
||||
let subject = ''; |
||||
if (subjects.querySelector('input[type="radio"]:checked').value) { |
||||
subject = `[data-subject="${ |
||||
subjects.querySelector('input[type="radio"]:checked').value |
||||
}"]`;
|
||||
} |
||||
$grid.isotope( { filter: `${subjectValue}${licenseValue}` } ); |
||||
} ); |
||||
$( '.licenses .filter-list a' ).click( e => { |
||||
e.preventDefault(); |
||||
if ( $( e.currentTarget ).hasClass( 'is-active' ) ) { |
||||
$( '.licenses .filter-list a' ).removeClass( 'is-active' ); |
||||
$( '.licenses' ).removeClass( 'has-active-child' ); |
||||
} else { |
||||
$( '.licenses .filter-list a' ).removeClass( 'is-active' ); |
||||
$( e.currentTarget ).addClass( 'is-active' ); |
||||
$( '.licenses' ).addClass( 'has-active-child' ); |
||||
license = `[data-license="${event.target.value}"]`; |
||||
alert(`[data-license="${event.target.value}"]${subject}`); |
||||
$grid.isotope({ |
||||
filter: `[data-license="${event.target.value}"]${subject}`, |
||||
}); |
||||
}); |
||||
subjects.addEventListener('click', function(event) { |
||||
if (event.target.type !== 'radio') { |
||||
return; |
||||
} |
||||
let subjectValue = $( '.subjects .filter-list a.is-active' ).attr( |
||||
'data-filter' |
||||
); |
||||
let licenseValue = $( '.licenses .filter-list a.is-active' ).attr( |
||||
'data-filter' |
||||
); |
||||
if ( typeof licenseValue === 'undefined' ) { |
||||
licenseValue = ''; |
||||
} else { |
||||
licenseValue = `[data-license="${licenseValue}"]`; |
||||
let license = ''; |
||||
let subject = ''; |
||||
if (licenses.querySelector('input[type="radio"]:checked').value) { |
||||
license = `[data-license="${ |
||||
licenses.querySelector('input[type="radio"]:checked').value |
||||
}"]`;
|
||||
} |
||||
if ( typeof subjectValue === 'undefined' ) { |
||||
subjectValue = ''; |
||||
} else { |
||||
subjectValue = `[data-subject="${subjectValue}"]`; |
||||
subject = `[data-subject="${event.target.value}"]`; |
||||
alert(`${license}[data-subject="${event.target.value}"]`); |
||||
$grid.isotope({ |
||||
filter: `${license}${subject}`, |
||||
}); |
||||
}); |
||||
sorts.addEventListener('click', function(event) { |
||||
if (event.target.type !== 'radio') { |
||||
return; |
||||
} |
||||
$grid.isotope( { filter: `${subjectValue}${licenseValue}` } ); |
||||
} ); |
||||
$( '.sort > a' ).click( e => { |
||||
e.preventDefault(); |
||||
$( '.sort' ).toggleClass( 'is-active' ); |
||||
} ); |
||||
$( '.sorts a' ).click( e => { |
||||
e.preventDefault(); |
||||
let sortBy = $( e.currentTarget ).attr( 'data-sort' ); |
||||
$( '.sorts a' ).removeClass( 'is-active' ); |
||||
$( e.currentTarget ).addClass( 'is-active' ); |
||||
$grid.isotope( { sortBy: sortBy } ); |
||||
} ); |
||||
} ); |
||||
$grid.isotope({ sortBy: event.target.value }); |
||||
}); |
||||
// $('.filters > a').click(e => {
|
||||
// e.preventDefault();
|
||||
// $('.filters').toggleClass('is-active');
|
||||
// $('.filter-groups > div').removeClass('is-active');
|
||||
// });
|
||||
// $('.filter-groups .subjects > a').click(e => {
|
||||
// e.preventDefault();
|
||||
// let id = $(e.currentTarget).attr('href');
|
||||
// $(`.filter-groups .subjects:not(${id})`).removeClass('is-active');
|
||||
// $(`.filter-groups ${id}`).toggleClass('is-active');
|
||||
// });
|
||||
// $('.licenses > a').click(e => {
|
||||
// e.preventDefault();
|
||||
// let id = $(e.currentTarget).attr('href');
|
||||
// $(id).toggleClass('is-active');
|
||||
// });
|
||||
// $('.subjects .filter-list a').click(e => {
|
||||
// e.preventDefault();
|
||||
// if ($(e.currentTarget).hasClass('is-active')) {
|
||||
// $('.subjects .filter-list a').removeClass('is-active');
|
||||
// $('.subjects').removeClass('has-active-child');
|
||||
// } else {
|
||||
// $('.subjects .filter-list a').removeClass('is-active');
|
||||
// $(e.currentTarget).addClass('is-active');
|
||||
// $('.subjects').removeClass('has-active-child');
|
||||
// $(e.currentTarget)
|
||||
// .parent()
|
||||
// .parent()
|
||||
// .parent('.subjects')
|
||||
// .addClass('has-active-child');
|
||||
// }
|
||||
// let subjectValue = $('.subjects .filter-list a.is-active').attr(
|
||||
// 'data-filter'
|
||||
// );
|
||||
// let licenseValue = $('.licenses .filter-list a.is-active').attr(
|
||||
// 'data-filter'
|
||||
// );
|
||||
// if (typeof licenseValue === 'undefined') {
|
||||
// licenseValue = '';
|
||||
// } else {
|
||||
// licenseValue = `[data-license="${licenseValue}"]`;
|
||||
// }
|
||||
// if (typeof subjectValue === 'undefined') {
|
||||
// subjectValue = '';
|
||||
// } else {
|
||||
// subjectValue = `[data-subject="${subjectValue}"]`;
|
||||
// }
|
||||
// $grid.isotope({ filter: `${subjectValue}${licenseValue}` });
|
||||
// });
|
||||
// $('.licenses .filter-list a').click(e => {
|
||||
// e.preventDefault();
|
||||
// if ($(e.currentTarget).hasClass('is-active')) {
|
||||
// $('.licenses .filter-list a').removeClass('is-active');
|
||||
// $('.licenses').removeClass('has-active-child');
|
||||
// } else {
|
||||
// $('.licenses .filter-list a').removeClass('is-active');
|
||||
// $(e.currentTarget).addClass('is-active');
|
||||
// $('.licenses').addClass('has-active-child');
|
||||
// }
|
||||
// let subjectValue = $('.subjects .filter-list a.is-active').attr(
|
||||
// 'data-filter'
|
||||
// );
|
||||
// let licenseValue = $('.licenses .filter-list a.is-active').attr(
|
||||
// 'data-filter'
|
||||
// );
|
||||
// if (typeof licenseValue === 'undefined') {
|
||||
// licenseValue = '';
|
||||
// } else {
|
||||
// licenseValue = `[data-license="${licenseValue}"]`;
|
||||
// }
|
||||
// if (typeof subjectValue === 'undefined') {
|
||||
// subjectValue = '';
|
||||
// } else {
|
||||
// subjectValue = `[data-subject="${subjectValue}"]`;
|
||||
// }
|
||||
// $grid.isotope({ filter: `${subjectValue}${licenseValue}` });
|
||||
// });
|
||||
// $('.sort > a').click(e => {
|
||||
// e.preventDefault();
|
||||
// $('.sort').toggleClass('is-active');
|
||||
// });
|
||||
// $('.sorts a').click(e => {
|
||||
// e.preventDefault();
|
||||
// let sortBy = $(e.currentTarget).attr('data-sort');
|
||||
// $('.sorts a').removeClass('is-active');
|
||||
// $(e.currentTarget).addClass('is-active');
|
||||
// $grid.isotope({ sortBy: sortBy });
|
||||
// });
|
||||
}); |
||||
}, |
||||
finalize() {}, |
||||
}; |
||||
|
@ -0,0 +1,106 @@
|
||||
fieldset { |
||||
border-top: solid 2px var(--accent); |
||||
font-family: $font-family-sans-serif; |
||||
|
||||
h2, |
||||
h3 { |
||||
margin-bottom: 0; |
||||
font-size: 1rem; |
||||
font-weight: bold; |
||||
text-align: left; |
||||
text-transform: none; |
||||
|
||||
&:before { |
||||
display: none; |
||||
} |
||||
|
||||
button { |
||||
display: flex; |
||||
flex-direction: row; |
||||
justify-content: space-between; |
||||
all: inherit; |
||||
width: 100%; |
||||
padding: 1rem 1.1875rem; |
||||
margin: 0; |
||||
border-top: 0; |
||||
|
||||
svg { |
||||
display: block; |
||||
float: right; |
||||
margin-top: 0.5rem; |
||||
} |
||||
|
||||
&:hover, |
||||
&:focus { |
||||
color: var(--brand); |
||||
background: var(--bg-body); |
||||
} |
||||
|
||||
&:active { |
||||
box-shadow: none; |
||||
} |
||||
|
||||
&[aria-expanded="true"] { |
||||
color: var(--brand); |
||||
|
||||
svg { |
||||
transform: rotate(180deg); |
||||
} |
||||
} |
||||
} |
||||
} |
||||
|
||||
h2 { |
||||
button[aria-expanded="true"] { |
||||
border-bottom: solid 2px var(--accent); |
||||
background: #fafdff; |
||||
} |
||||
} |
||||
|
||||
[type="radio"] { |
||||
position: absolute !important; |
||||
width: 1px !important; |
||||
height: 1px !important; |
||||
padding: 0 !important; |
||||
border: 0 !important; |
||||
overflow: hidden !important; |
||||
clip: rect(1px, 1px, 1px, 1px); |
||||
} |
||||
|
||||
[type="radio"] + label { |
||||
cursor: pointer; |
||||
display: block; |
||||
padding: 1rem 1.1875rem; |
||||
margin-bottom: 0; |
||||
|
||||
svg { |
||||
display: none; |
||||
} |
||||
} |
||||
|
||||
[type="radio"]:focus { |
||||
label { |
||||
cursor: pointer; |
||||
display: block; |
||||
} |
||||
} |
||||
|
||||
[type="radio"]:checked + label { |
||||
color: var(--brand); |
||||
font-weight: bold; |
||||
|
||||
svg { |
||||
display: block; |
||||
float: right; |
||||
margin-top: 0.5rem; |
||||
width: 1rem; |
||||
height: 1rem; |
||||
fill: transparent; |
||||
} |
||||
} |
||||
|
||||
&:last-of-type { |
||||
border-bottom: solid 2px var(--accent); |
||||
margin-bottom: 1rem; |
||||
} |
||||
} |
@ -1,6 +1,6 @@
|
||||
{ |
||||
"/scripts/aldine.js": "/scripts/aldine.js?id=c8641d03dfb38dc75e0d", |
||||
"/styles/aldine.css": "/styles/aldine.css?id=7fca925f82ab0be23d54", |
||||
"/styles/editor.css": "/styles/editor.css?id=10e3f4b144847aa8d75e", |
||||
"/scripts/customizer.js": "/scripts/customizer.js?id=f1f1f4225cba4c1b35f2" |
||||
"/scripts/aldine.js": "/scripts/aldine.js", |
||||
"/styles/aldine.css": "/styles/aldine.css", |
||||
"/styles/editor.css": "/styles/editor.css", |
||||
"/scripts/customizer.js": "/scripts/customizer.js" |
||||
} |
File diff suppressed because one or more lines are too long
File diff suppressed because one or more lines are too long
File diff suppressed because one or more lines are too long
File diff suppressed because one or more lines are too long
Loading…
Reference in new issue