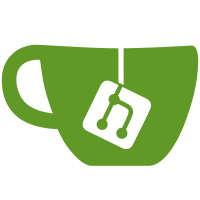
13 changed files with 222 additions and 69 deletions
@ -0,0 +1,8 @@
|
||||
<?php |
||||
|
||||
namespace App; |
||||
|
||||
add_action('widgets_init', function () { |
||||
register_widget('Aldine\LinkButton'); |
||||
register_widget('Aldine\PageButton'); |
||||
}); |
@ -0,0 +1,72 @@
|
||||
<?php |
||||
|
||||
namespace Aldine; |
||||
|
||||
class LinkButton extends \WP_Widget |
||||
{ |
||||
/** |
||||
* Constructor. |
||||
* |
||||
* @see WP_Widget::__construct() |
||||
* |
||||
*/ |
||||
public function __construct() |
||||
{ |
||||
parent::__construct('linkbutton', __('Link Button', 'pressbooks-aldine'), [ |
||||
'description' => esc_html__('Add a styled link button.', 'pressbooks-aldine') |
||||
]); |
||||
} |
||||
|
||||
/** |
||||
* Front-end display of widget. |
||||
* |
||||
* @see WP_Widget::widget() |
||||
* |
||||
* @param array $args Widget arguments. |
||||
* @param array $instance Saved values from database. |
||||
*/ |
||||
public function widget($args, $instance) |
||||
{ |
||||
echo $args['before_widget']; |
||||
if (! empty($instance['url']) && ! empty($instance['title'])) { |
||||
printf('<a class="button" href="%1$s">%2$s</a>', $instance['url'], apply_filters('widget_title', $instance['title'])); |
||||
} |
||||
echo $args['after_widget']; |
||||
} |
||||
|
||||
/** |
||||
* Back-end widget form. |
||||
* |
||||
* @see WP_Widget::form() |
||||
* |
||||
* @param array $instance Previously saved values from database. |
||||
*/ |
||||
public function form($instance) |
||||
{ |
||||
$title = ! empty($instance['title']) ? $instance['title'] : ''; |
||||
$url = ! empty($instance['url']) ? $instance['url'] : ''; ?> |
||||
<p><label for="<?php echo esc_attr($this->get_field_id('title')); ?>"><?php esc_attr_e('Title:', 'pressbooks-aldine'); ?></label>
|
||||
<input class="widefat" id="<?php echo esc_attr($this->get_field_id('title')); ?>" name="<?php echo esc_attr($this->get_field_name('title')); ?>" type="text" value="<?php echo esc_attr($title); ?>"></p>
|
||||
<p><label for="<?php echo esc_attr($this->get_field_id('url')); ?>"><?php esc_attr_e('URL:', 'pressbooks-aldine'); ?></label>
|
||||
<input class="widefat code" id="<?php echo esc_attr($this->get_field_id('url')); ?>" name="<?php echo esc_attr($this->get_field_name('url')); ?>" type="text" value="<?php echo esc_attr($url); ?>"></p>
|
||||
<?php |
||||
} |
||||
|
||||
/** |
||||
* Sanitize widget form values as they are saved. |
||||
* |
||||
* @see WP_Widget::update() |
||||
* |
||||
* @param array $new_instance Values just sent to be saved. |
||||
* @param array $old_instance Previously saved values from database. |
||||
* |
||||
* @return array Updated safe values to be saved. |
||||
*/ |
||||
public function update($new_instance, $old_instance) |
||||
{ |
||||
$instance = []; |
||||
$instance['title'] = ( ! empty($new_instance['title']) ) ? strip_tags($new_instance['title']) : ''; |
||||
$instance['url'] = ( ! empty($new_instance['url']) ) ? esc_url($new_instance['url']) : ''; |
||||
return $instance; |
||||
} |
||||
} |
@ -0,0 +1,81 @@
|
||||
<?php |
||||
|
||||
namespace Aldine; |
||||
|
||||
class PageButton extends \WP_Widget |
||||
{ |
||||
/** |
||||
* Constructor. |
||||
* |
||||
* @see WP_Widget::__construct() |
||||
* |
||||
*/ |
||||
public function __construct() |
||||
{ |
||||
parent::__construct('pagebutton', __('Page Button', 'pressbooks-aldine'), [ |
||||
'description' => esc_html__('Add a styled button which links to a page.', 'pressbooks-aldine') |
||||
]); |
||||
} |
||||
|
||||
/** |
||||
* Front-end display of widget. |
||||
* |
||||
* @see WP_Widget::widget() |
||||
* |
||||
* @param array $args Widget arguments. |
||||
* @param array $instance Saved values from database. |
||||
*/ |
||||
public function widget($args, $instance) |
||||
{ |
||||
echo $args['before_widget']; |
||||
if (! empty($instance['page_id'])) { |
||||
if (empty($instance['title'])) { |
||||
$instance['title'] = get_the_title($instance['page_id']); |
||||
} |
||||
printf('<a class="button" href="%1$s">%2$s</a>', get_permalink($instance['page_id']), apply_filters('widget_title', $instance['title'])); |
||||
} |
||||
echo $args['after_widget']; |
||||
} |
||||
|
||||
/** |
||||
* Back-end widget form. |
||||
* |
||||
* @see WP_Widget::form() |
||||
* |
||||
* @param array $instance Previously saved values from database. |
||||
*/ |
||||
public function form($instance) |
||||
{ |
||||
$title = ! empty($instance['title']) ? $instance['title'] : ''; |
||||
$url = ! empty($instance['page_id']) ? $instance['page_id'] : ''; ?> |
||||
<p><label for="<?php echo esc_attr($this->get_field_id('title')); ?>"><?php esc_attr_e('Title:', 'pressbooks-aldine'); ?></label>
|
||||
<input class="widefat" id="<?php echo esc_attr($this->get_field_id('title')); ?>" name="<?php echo esc_attr($this->get_field_name('title')); ?>" type="text" value="<?php echo esc_attr($title); ?>"></p>
|
||||
<p><label for="<?php echo esc_attr($this->get_field_id('page_id')); ?>"><?php esc_attr_e('Page:', 'pressbooks-aldine'); ?></label>
|
||||
<select id="<?php echo esc_attr($this->get_field_id('page_id')); ?>" name="<?php echo esc_attr($this->get_field_name('page_id')); ?>">
|
||||
<option value="" <?php selected($instance['page_id'], ''); ?>> -- </a>
|
||||
<?php $pages = get_pages(); |
||||
foreach ($pages as $page) { ?> |
||||
<option value="<?= $page->ID; ?>" <?php selected(@$instance['page_id'], $page->ID); ?>><?= $page->post_title; ?></option>
|
||||
<?php } ?> |
||||
</select></p> |
||||
<?php |
||||
} |
||||
|
||||
/** |
||||
* Sanitize widget form values as they are saved. |
||||
* |
||||
* @see WP_Widget::update() |
||||
* |
||||
* @param array $new_instance Values just sent to be saved. |
||||
* @param array $old_instance Previously saved values from database. |
||||
* |
||||
* @return array Updated safe values to be saved. |
||||
*/ |
||||
public function update($new_instance, $old_instance) |
||||
{ |
||||
$instance = []; |
||||
$instance['title'] = ( ! empty($new_instance['title']) ) ? strip_tags($new_instance['title']) : ''; |
||||
$instance['page_id'] = ( ! empty($new_instance['page_id']) ) ? absint($new_instance['page_id']) : ''; |
||||
return $instance; |
||||
} |
||||
} |
File diff suppressed because one or more lines are too long
@ -0,0 +1,25 @@
|
||||
.button { |
||||
display: inline-block; |
||||
width: 250px; |
||||
height: 60px; |
||||
margin-top: 2em; |
||||
padding: 1.25em; |
||||
border: 2px solid $brand-primary; |
||||
border-radius: 3px; |
||||
background: $brand-primary; |
||||
font-family: Karla, sans-serif; |
||||
font-size: 16px; |
||||
font-weight: 600; |
||||
color: $white; |
||||
letter-spacing: 2px; |
||||
text-align: center; |
||||
text-decoration: none; |
||||
text-transform: uppercase; |
||||
transition: all 0.5s; |
||||
|
||||
&:hover, |
||||
&:focus { |
||||
background: $white; |
||||
color: $brand-primary; |
||||
} |
||||
} |
Loading…
Reference in new issue