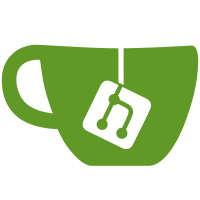
52 changed files with 242 additions and 299 deletions
@ -0,0 +1,9 @@ |
|||||||
|
name: Roblib Search EDS |
||||||
|
type: module |
||||||
|
configure: roblib_search_eds.settings |
||||||
|
description: Implements the Roblib Search EDS book and article results |
||||||
|
package: Roblib Search |
||||||
|
core_version_requirement: ^8.9 || ^9 |
||||||
|
dependancies: |
||||||
|
- roblib_search |
||||||
|
- ebsco |
@ -0,0 +1,8 @@ |
|||||||
|
roblib_search_eds.settings: |
||||||
|
path: '/admin/config/system/roblib_search_eds_settings' |
||||||
|
defaults: |
||||||
|
_form: '\Drupal\roblib_search_eds\Form\EdsSettingsForm' |
||||||
|
_title: 'Roblib Search EDS Settings Form' |
||||||
|
requirements: |
||||||
|
_permission: 'administer site configuration' |
||||||
|
|
@ -0,0 +1,137 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Drupal\roblib_search_eds\Form; |
||||||
|
|
||||||
|
use Drupal\Core\Form\FormBase; |
||||||
|
use Drupal\Core\Form\FormStateInterface; |
||||||
|
|
||||||
|
/** |
||||||
|
* Settings form for module |
||||||
|
* |
||||||
|
* @author ppound |
||||||
|
*/ |
||||||
|
class EdsSettingsForm extends FormBase { |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function getFormId() { |
||||||
|
return 'roblib_search_eds_settings_form'; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function buildForm(array $form, FormStateInterface $form_state) { |
||||||
|
$config = \Drupal::config('roblib_search_eds.settings'); |
||||||
|
$form['eds_rest_url'] = array( |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => t('EDS Rest endpoint'), |
||||||
|
'#default_value' => $config->get('eds_rest_url'), |
||||||
|
'#description' => t('The base EDS URL, for example https://eds-api.ebscohost.com/edsapi/rest'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
$form['eds_auth_url'] = array( |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => t('EDS Auth endpoint'), |
||||||
|
'#default_value' => $config->get('eds_auth_url'), |
||||||
|
'#description' => t('The EDS Auth endpoint, for example https://eds-api.ebscohost.com/Authservice/rest'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
|
||||||
|
$form['eds_user'] = array( |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => t('EDS user'), |
||||||
|
'#default_value' => $config->get('eds_user'), |
||||||
|
'#description' => t('EDS user, for example username'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
|
||||||
|
$form['eds_pass'] = array( |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => t('EDS password'), |
||||||
|
'#default_value' => $config->get('eds_pass'), |
||||||
|
'#description' => t('EDS password, for example password'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
|
||||||
|
$form['eds_guest'] = array( |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => t('EDS Guest access'), |
||||||
|
'#default_value' => $config->get('eds_guest'), |
||||||
|
'#description' => t('EDS Guest access, y = guest access, n = not a guest'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
|
||||||
|
$form['eds_article_profile'] = array( |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => t('EDS Article profile'), |
||||||
|
'#default_value' => $config->get('eds_article_profile'), |
||||||
|
'#description' => t('EDS profile, for example edsapi'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
|
||||||
|
$form['eds_book_profile'] = array( |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => t('EDS Book profile'), |
||||||
|
'#default_value' => $config->get('eds_book_profile'), |
||||||
|
'#description' => t('EDS Book profile, for example apilite'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
|
||||||
|
$form['eds_num_results'] = array( |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => t('Number of results to return'), |
||||||
|
'#default_value' => $config->get('eds_num_results'), |
||||||
|
'#description' => t('The number of results to display in the Bento box, usurally 5'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
$form['eds_article_limiters'] = array( |
||||||
|
'#type' => 'textarea', |
||||||
|
'#title' => t('The limiters for Article searches'), |
||||||
|
'#default_value' => $config->get('eds_article_limiters'), |
||||||
|
'#description' => t('Specify the Publication Types to use as limiters for Article searches. Formatted similar to |
||||||
|
AND (PT Article OR PT Magazines OR PT News OR PT Academic Journals OR PT Conference Materials)'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
$form['eds_book_limiters'] = array( |
||||||
|
'#type' => 'textarea', |
||||||
|
'#title' => t('The limiters for Books and Media searches'), |
||||||
|
'#default_value' => $config->get('eds_book_limiters'), |
||||||
|
'#description' => t('Specify the Publication Types to use as limiters for Article searches. Formatted similar to |
||||||
|
AND (PT Book OR PT Video OR PT Audio'), |
||||||
|
'#required' => TRUE, |
||||||
|
); |
||||||
|
$form['actions']['submit'] = [ |
||||||
|
'#type' => 'submit', |
||||||
|
'#value' => t('Save'), |
||||||
|
'#button_type' => 'primary', |
||||||
|
]; |
||||||
|
return $form; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function validateForm(array &$form, FormStateInterface $form_state) { |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function submitForm(array &$form, FormStateInterface $form_state) { |
||||||
|
$config = \Drupal::configFactory()->getEditable('roblib_search_eds.settings'); |
||||||
|
$config->set('eds_rest_url', $form_state->getValue('eds_rest_url'))->save(); |
||||||
|
$config->set('eds_auth_url', $form_state->getValue('eds_auth_url'))->save(); |
||||||
|
$config->set('eds_user', $form_state->getValue('eds_user'))->save(); |
||||||
|
$config->set('eds_pass', $form_state->getValue('eds_pass'))->save(); |
||||||
|
$config->set('eds_guest', $form_state->getValue('eds_guest'))->save(); |
||||||
|
$config->set('eds_article_profile', $form_state->getValue('eds_article_profile'))->save(); |
||||||
|
$config->set('eds_book_profile', $form_state->getValue('eds_book_profile'))->save(); |
||||||
|
$config->set('eds_num_results', $form_state->getValue('eds_num_results'))->save(); |
||||||
|
$config->set('eds_article_limiters', $form_state->getValue('eds_article_limiters'))->save(); |
||||||
|
$config->set('eds_book_limiters', $form_state->getValue('eds_book_limiters'))->save(); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,27 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Drupal\roblib_search_eds\Plugin\Block; |
||||||
|
|
||||||
|
use Drupal\Core\Block\BlockBase; |
||||||
|
|
||||||
|
/** |
||||||
|
* Provides EDS Article search results. |
||||||
|
* |
||||||
|
* @Block( |
||||||
|
* id = "roblib_search_eds_articles_block", |
||||||
|
* admin_label = @Translation("Roblib Search EDS Articles Block"), |
||||||
|
* category = @Translation("Roblib Search"), |
||||||
|
* ) |
||||||
|
*/ |
||||||
|
class RoblibSearchEdsArticles extends BlockBase { |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function build() { |
||||||
|
return [ |
||||||
|
'#theme' => 'roblib_search_eds_articles', |
||||||
|
'#query' => $query, |
||||||
|
]; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,27 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Drupal\roblib_search_eds\Plugin\Block; |
||||||
|
|
||||||
|
use Drupal\Core\Block\BlockBase; |
||||||
|
|
||||||
|
/** |
||||||
|
* Provides EDS book search results. |
||||||
|
* |
||||||
|
* @Block( |
||||||
|
* id = "roblib_search_eds_books_block", |
||||||
|
* admin_label = @Translation("Roblib Search EDS Books Block"), |
||||||
|
* category = @Translation("Roblib Search"), |
||||||
|
* ) |
||||||
|
*/ |
||||||
|
class RoblibSearchEdsBooks extends BlockBase { |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function build() { |
||||||
|
return [ |
||||||
|
'#theme' => 'roblib_search_eds_books', |
||||||
|
'#query' => $query, |
||||||
|
]; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,12 @@ |
|||||||
|
|
||||||
|
<div class ="roblib-search-more" id="roblib-search-eds-article-more"></div> |
||||||
|
<div class ="roblib-search-content eds-article" id="roblib-search-content-eds-articles"> |
||||||
|
|
||||||
|
|
||||||
|
</div> |
||||||
|
|
||||||
|
<div id="roblib-eds-articles-more-results" class="roblib-eds-more-button button"></div> |
||||||
|
<div id="roblib-eds-articles-toc" class="roblib-bento-toc moveme-please"></div> |
||||||
|
|
||||||
|
|
||||||
|
|
@ -1,13 +1,6 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
/** |
|
||||||
* @file roblib-search-eds.tpl.php |
|
||||||
* |
|
||||||
*/ |
|
||||||
?> |
|
||||||
<div class ="roblib-search-more" id="roblib-search-eds-more"></div> |
<div class ="roblib-search-more" id="roblib-search-eds-more"></div> |
||||||
<div class ="roblib-search-content eds" id="roblib-search-content-eds"> |
<div class ="roblib-search-content eds" id="roblib-search-content-eds"> |
||||||
<img src="<?php print (empty($spinner_path) ? ' ' : $spinner_path); ?>"/> |
|
||||||
|
|
||||||
</div> |
</div> |
||||||
<div id="roblib-eds-books-more-results" class="roblib-eds-more-button button"></div> |
<div id="roblib-eds-books-more-results" class="roblib-eds-more-button button"></div> |
@ -1,133 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace Drupal\roblib_search\Form; |
|
||||||
|
|
||||||
use Drupal\Core\Form\FormBase; |
|
||||||
use Drupal\Core\Form\FormStateInterface; |
|
||||||
|
|
||||||
/** |
|
||||||
* Settings form for module |
|
||||||
* |
|
||||||
* @author ppound |
|
||||||
*/ |
|
||||||
class RoblibSearchEdsSettingsForm extends FormBase { |
|
||||||
|
|
||||||
/** |
|
||||||
* {@inheritdoc} |
|
||||||
*/ |
|
||||||
public function getFormId() { |
|
||||||
return 'roblib_search_eds_settings_form'; |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* {@inheritdoc} |
|
||||||
*/ |
|
||||||
public function buildForm(array $form, FormStateInterface $form_state) { |
|
||||||
$config = \Drupal::config('roblib_search_eds.settings'); |
|
||||||
$form['eds_rest_url'] = array( |
|
||||||
'#type' => 'textfield', |
|
||||||
'#title' => t('EDS Rest endpoint'), |
|
||||||
'#default_value' => $config->get('eds_rest_url'), |
|
||||||
'#description' => t('The base EDS URL, for example https://eds-api.ebscohost.com/edsapi/rest'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
$form['eds_auth_url'] = array( |
|
||||||
'#type' => 'textfield', |
|
||||||
'#title' => t('EDS Auth endpoint'), |
|
||||||
'#default_value' => $config->get('eds_auth_url'), |
|
||||||
'#description' => t('The EDS Auth endpoint, for example https://eds-api.ebscohost.com/Authservice/rest'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
|
|
||||||
$form['eds_user'] = array( |
|
||||||
'#type' => 'textfield', |
|
||||||
'#title' => t('EDS user'), |
|
||||||
'#default_value' => $config->get('eds_user'), |
|
||||||
'#description' => t('EDS user, for example username'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
|
|
||||||
$form['eds_pass'] = array( |
|
||||||
'#type' => 'textfield', |
|
||||||
'#title' => t('EDS password'), |
|
||||||
'#default_value' => $config->get('eds_pass'), |
|
||||||
'#description' => t('EDS password, for example password'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
|
|
||||||
$form['eds_guest'] = array( |
|
||||||
'#type' => 'textfield', |
|
||||||
'#title' => t('EDS Guest access'), |
|
||||||
'#default_value' => $config->get('eds_guest'), |
|
||||||
'#description' => t('EDS Guest access, y = guest access, n = not a guest'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
|
|
||||||
$form['eds_article_profile'] = array( |
|
||||||
'#type' => 'textfield', |
|
||||||
'#title' => t('EDS Article profile'), |
|
||||||
'#default_value' => $config->get('eds_article_profile'), |
|
||||||
'#description' => t('EDS profile, for example edsapi'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
|
|
||||||
$form['eds_book_profile'] = array( |
|
||||||
'#type' => 'textfield', |
|
||||||
'#title' => t('EDS Book profile'), |
|
||||||
'#default_value' => $config->get('eds_book_profile'), |
|
||||||
'#description' => t('EDS Book profile, for example apilite'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
|
|
||||||
$form['eds_num_results'] = array( |
|
||||||
'#type' => 'textfield', |
|
||||||
'#title' => t('Number of results to return'), |
|
||||||
'#default_value' => $config->get('eds_num_results'), |
|
||||||
'#description' => t('The number of results to display in the Bento box, usurally 5'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
$form['eds_article_limiters'] = array( |
|
||||||
'#type' => 'textarea', |
|
||||||
'#title' => t('The limiters for Article searches'), |
|
||||||
'#default_value' => $config->get('eds_article_limiters'), |
|
||||||
'#description' => t('Specify the Publication Types to use as limiters for Article searches. Formatted similar to |
|
||||||
AND (PT Article OR PT Magazines OR PT News OR PT Academic Journals OR PT Conference Materials)'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
$form['eds_book_limiters'] = array( |
|
||||||
'#type' => 'textarea', |
|
||||||
'#title' => t('The limiters for Books and Media searches'), |
|
||||||
'#default_value' => $config->get('eds_book_limiters'), |
|
||||||
'#description' => t('Specify the Publication Types to use as limiters for Article searches. Formatted similar to |
|
||||||
AND (PT Book OR PT Video OR PT Audio'), |
|
||||||
'#required' => TRUE, |
|
||||||
); |
|
||||||
return $form; |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* {@inheritdoc} |
|
||||||
*/ |
|
||||||
public function validateForm(array &$form, FormStateInterface $form_state) { |
|
||||||
|
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* {@inheritdoc} |
|
||||||
*/ |
|
||||||
public function submitForm(array &$form, FormStateInterface $form_state) { |
|
||||||
$config = \Drupal::configFactory()->getEditable('roblib_search_eds.settings'); |
|
||||||
$config->set('eds_rest_url', $form_state->getValue('eds_rest_url'))->save(); |
|
||||||
$config->set('eds_auth_url', $form_state->getValue('eds_auth_url'))->save(); |
|
||||||
$config->set('eds_user', $form_state->getValue('eds_user'))->save(); |
|
||||||
$config->set('eds_pass', $form_state->getValue('eds_pass'))->save(); |
|
||||||
$config->set('eds_guest', $form_state->getValue('eds_guest'))->save(); |
|
||||||
$config->set('eds_article_profile', $form_state->getValue('eds_article_profile'))->save(); |
|
||||||
$config->set('eds_book_profile', $form_state->getValue('eds_book_profile'))->save(); |
|
||||||
$config->set('eds_num_results', $form_state->getValue('eds_num_results'))->save(); |
|
||||||
$config->set('eds_article_limiters', $form_state->getValue('eds_article_limiters'))->save(); |
|
||||||
$config->set('eds_book_limiters', $form_state->getValue('eds_book_limiters'))->save(); |
|
||||||
|
|
||||||
} |
|
||||||
|
|
||||||
} |
|
@ -1,8 +0,0 @@ |
|||||||
name: Roblib Search EDS |
|
||||||
configure: admin/roblib_search/eds_search |
|
||||||
description: Implements the Roblib Search modules _roblib_search hook |
|
||||||
package: Roblib Search |
|
||||||
core_version_requirement: ^8.9 || ^9 |
|
||||||
dependancies: |
|
||||||
- roblib_search |
|
||||||
- ebsco |
|
@ -1,25 +0,0 @@ |
|||||||
roblib_search_eds.settings: |
|
||||||
path: '/admin/config/system/roblib_search' |
|
||||||
defaults: |
|
||||||
_form: '\Drupal\roblib_search_eds\Form\RoblibSearchEdsSettingsForm' |
|
||||||
_title: 'Roblib Search EDS Settings Form' |
|
||||||
requirements: |
|
||||||
_permission: 'administer site configuration' |
|
||||||
|
|
||||||
roblib_search_eds.article_search: |
|
||||||
path: '/roblib_search/eds/articles/{query}' |
|
||||||
defaults: |
|
||||||
_controller: '\Drupal\roblib_search_eds\Controller\RoblibSearchEdsController::articleResults' |
|
||||||
_title: 'Roblib Search EDS Articles' |
|
||||||
query: '[a-zA-Z\s]+' |
|
||||||
requirements: |
|
||||||
_permission: 'access content' |
|
||||||
|
|
||||||
roblib_search_eds.book_search: |
|
||||||
path: '/roblib_search/eds/book/{query}' |
|
||||||
defaults: |
|
||||||
_controller: '\Drupal\roblib_search_eds\Controller\RoblibSearchEdsController::bookResults' |
|
||||||
_title: 'Roblib Search EDS Articles' |
|
||||||
query: '[a-zA-Z\s]+' |
|
||||||
requirements: |
|
||||||
_permission: 'access content' |
|
@ -1,35 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
/** |
|
||||||
* @file roblib-search-eds-article.tpl.php |
|
||||||
* Search ebscohost for articles |
|
||||||
* |
|
||||||
* Variables available: |
|
||||||
* - $variables: all array elements of $variables can be used as a variable. e.g. $base_url equals $variables['base_url'] |
|
||||||
* |
|
||||||
* |
|
||||||
*/ |
|
||||||
?> |
|
||||||
<div class ="roblib-search-more" id="roblib-search-eds-article-more"></div> |
|
||||||
<div class ="roblib-search-content eds-article" id="roblib-search-content-eds-articles"> |
|
||||||
<img src="<?php print (empty($spinner_path) ? ' ' : $spinner_path); ?>"/>
|
|
||||||
|
|
||||||
</div> |
|
||||||
|
|
||||||
<div id="roblib-eds-articles-more-results" class="roblib-eds-more-button button"></div> |
|
||||||
<div id="roblib-eds-articles-toc" class="roblib-bento-toc moveme-please"></div> |
|
||||||
<?php |
|
||||||
/** |
|
||||||
* Alternate way to create link back to ebscohost. |
|
||||||
<form action="" id = 'roblib-search-eds-articles' method="post" style="width: 375px; overflow: auto;" onsubmit="return ebscoHostSearchGo(this);"> |
|
||||||
<input id="ebscohosturl" name="ebscohosturl" type="hidden" value="https://search.ebscohost.com/login.aspx?direct=true&site=ehost-live&scope=site&type=0&custid=uprince&profid=eds&groupid=main&mode=and&cli0=RV&clv0=N&lang=en" /> |
|
||||||
<input id="ebscohostsearchsrc" name="ebscohostsearchsrc" type="hidden" value="url" /> <input id="ebscohostsearchmode" name="ebscohostsearchmode" type="hidden" value="+" /> |
|
||||||
<input id="ebscohostkeywords" name="ebscohostkeywords" type="hidden" value="" /> |
|
||||||
<div><input id="ebscohostsearchtext" name="ebscohostsearchtext" value="history" type="hidden" size="23" style="font-size: 9pt; padding-left: 5px; margin-left: 0px;" /> <input type="submit" value="Search" style="font-size: 9pt; padding-left: 5px;" /></div> |
|
||||||
</div> |
|
||||||
|
|
||||||
</form> |
|
||||||
*/ |
|
||||||
?> |
|
||||||
|
|
||||||
|
|
Loading…
Reference in new issue