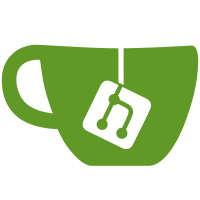
5 changed files with 266 additions and 0 deletions
@ -0,0 +1,62 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\upei_roblib_ill\Controller; |
||||
|
||||
use Drupal\Core\Controller\ControllerBase; |
||||
use Drupal\Core\Url; |
||||
use Drupal\Core\Link; |
||||
use Symfony\Component\HttpFoundation\Response; |
||||
|
||||
/** |
||||
* Controller for d3 graphs. |
||||
*/ |
||||
class RoblibIllController extends ControllerBase { |
||||
|
||||
/** |
||||
* Show the user contact info etc. after ILL Request. |
||||
* @return string |
||||
* Rendered HTML |
||||
*/ |
||||
function finished() { |
||||
$config = \Drupal::config('upei_roblib_ill.settings'); |
||||
$contact_email = $config->get('ill_contact_email'); |
||||
$contact_phone_number = $config->get('ill_contact_phone'); |
||||
$librarian_link = Link::fromTextAndUrl($this->t('subject-specific'), Url::fromUri('http://library.upei.ca/librarians'))->toString(); |
||||
$help_message = $this->t("We can help! Please contact your !librarian_link librarian if you'd like help finding more resources relating to your topic.", |
||||
['!librarian_link' => $librarian_link]); |
||||
$standard_message = t("To contact the department about this request, you can send a message to @email or |
||||
call @phone.", ['@phone' => $contact_phone_number, '@email' => $contact_email]); |
||||
if (isset($_GET['error']) && $_GET['error'] === 'FALSE') { |
||||
$standard_message = t("A message including the Request ID has been sent to @email.", [ |
||||
'@email' => $_GET['email'], |
||||
]) . $standard_message; |
||||
} |
||||
|
||||
$output = [ |
||||
'#prefix' => '<div upei-roblib-ill-content>', |
||||
'#suffix' => '</div>', |
||||
//'#type' => 'page', |
||||
'dynamic_message' => [ |
||||
'#type' => 'markup', |
||||
'#markup' => $_GET['message'], |
||||
'#prefix' => '<div class="upei-roblib-ill-message">', |
||||
'#suffix' => '</div>', |
||||
], |
||||
'standard_message' => [ |
||||
'#type' => 'markup', |
||||
'#markup' => $standard_message, |
||||
'#prefix' => '<div class="upei-roblib-ill-email">', |
||||
'#suffix' => '</div>', |
||||
], |
||||
'help_message' => [ |
||||
'#type' => 'markup', |
||||
'#markup' => $help_message, |
||||
'#allowed_tags' => ['a', 'source'], |
||||
'#prefix' => '<div class="upei-roblib-ill-help">', |
||||
'#suffix' => '</div>', |
||||
], |
||||
]; |
||||
return $output; |
||||
} |
||||
|
||||
} |
@ -0,0 +1,47 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\upei_roblib_ill\Form; |
||||
|
||||
use Drupal\Core\Form\FormBase; |
||||
use Drupal\Core\Form\FormStateInterface; |
||||
|
||||
/** |
||||
* Settings form for module |
||||
* |
||||
* @author ppound |
||||
*/ |
||||
class RoblibIllLoanForm extends FormBase { |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function getFormId() { |
||||
return 'roblib_ill_loan_form'; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function buildForm(array $form, FormStateInterface $form_state) { |
||||
|
||||
|
||||
$form = []; |
||||
|
||||
return $form; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function validateForm(array &$form, FormStateInterface $form_state) { |
||||
|
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function submitForm(array &$form, FormStateInterface $form_state) { |
||||
|
||||
} |
||||
|
||||
} |
@ -0,0 +1,126 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\upei_roblib_ill\Form; |
||||
|
||||
use Drupal\Core\Form\FormBase; |
||||
use Drupal\Core\Form\FormStateInterface; |
||||
|
||||
/** |
||||
* Settings form for module |
||||
* |
||||
* @author ppound |
||||
*/ |
||||
class RoblibIllSettingsForm extends FormBase { |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function getFormId() { |
||||
return 'roblib_ill_settings_form'; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function buildForm(array $form, FormStateInterface $form_state) { |
||||
$config = \Drupal::config('upei_roblib_ill.settings'); |
||||
|
||||
$form = []; |
||||
$form['ill_add_url'] = [ |
||||
'#required' => TRUE, |
||||
'#type' => 'textfield', |
||||
'#size' => 200, |
||||
'#title' => t('The Relais AddRequest URL'), |
||||
'#description' => t('The URL for the Relais ILL server AddRequest service, the default is |
||||
https://caul-cbua.relais-host.com/portal-service/request/add'), |
||||
'#default_value' => $config->get('ill_add_url'), |
||||
]; |
||||
$form['ill_auth_url'] = [ |
||||
'#required' => TRUE, |
||||
'#type' => 'textfield', |
||||
'#size' => 200, |
||||
'#title' => t('The Relais Auth URL'), |
||||
'#description' => t('The URL for the Relais ILL server Auth service (retrieve the aid), the default is |
||||
https://caul-cbua.relais-host.com/portal-service/user/authentication'), |
||||
'#default_value' => $config->get('ill_auth_url'), |
||||
]; |
||||
$form['ill_library_symbol'] = [ |
||||
'#required' => TRUE, |
||||
'#type' => 'textfield', |
||||
'#size' => 200, |
||||
'#title' => t('Your Relais Library Symbol'), |
||||
'#description' => t('Your Relais Library Symbol'), |
||||
'#default_value' => $config->get('ill_library_symbol'), |
||||
]; |
||||
|
||||
$form['ill_relais_key'] = [ |
||||
'#required' => TRUE, |
||||
'#type' => 'textfield', |
||||
'#size' => 200, |
||||
'#title' => t('The Relais API key'), |
||||
'#description' => t('The API key used to communicate with the Relais API, Contact Relais International to get your key'), |
||||
'#default_value' => $config->get('ill_relais_key'), |
||||
]; |
||||
$form['ill_doi_openurl_pid'] = [ |
||||
'#required' => TRUE, |
||||
'#type' => 'textfield', |
||||
'#size' => 200, |
||||
'#title' => t('OpenURL PID'), |
||||
'#description' => t('An identifier to call yourself, for the OpenURL endpoint. To use this service you first need to register for an account here: http://www.crossref.org/requestaccount/'), |
||||
'#default_value' => $config->get('ill_doi_openurl_pid'), |
||||
]; |
||||
$form['ill_contact_email'] = [ |
||||
'#required' => TRUE, |
||||
'#type' => 'textfield', |
||||
'#size' => 200, |
||||
'#title' => t('Contact Email'), |
||||
'#description' => t('The email address we want to show after a user has submitted an ILL request, Roblib uses ill@upei.ca'), |
||||
'#default_value' => $config->get('ill_contact_email'), |
||||
]; |
||||
$form['ill_contact_phone'] = [ |
||||
'#required' => TRUE, |
||||
'#type' => 'textfield', |
||||
'#size' => 200, |
||||
'#title' => t('Contact Phone Number'), |
||||
'#description' => t('The phone number we want to show to the user after a user has submitted an ILL request, Roblib uses 902-566-0445'), |
||||
'#default_value' => $config->get('ill_contact_phone'), |
||||
]; |
||||
$form['ill_header_message'] = [ |
||||
'#required' => TRUE, |
||||
'#type' => 'textarea', |
||||
'#title' => t('ILL Header Message'), |
||||
'#description' => t('The message that appears at the top of the ILL form, recently used for Covid messages. Leave this blank for no message to appear at the top of the form.'), |
||||
'#default_value' => $config->get('ill_header_message'), |
||||
]; |
||||
$form['actions']['submit'] = [ |
||||
'#type' => 'submit', |
||||
'#value' => t('Save'), |
||||
'#button_type' => 'primary', |
||||
]; |
||||
|
||||
return $form; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function validateForm(array &$form, FormStateInterface $form_state) { |
||||
|
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function submitForm(array &$form, FormStateInterface $form_state) { |
||||
$config = \Drupal::configFactory()->getEditable('upei_roblib_ill.settings'); |
||||
$config->set('ill_add_url', $form_state->getValue('ill_add_url'))->save(); |
||||
$config->set('ill_auth_url', $form_state->getValue('ill_auth_url'))->save(); |
||||
$config->set('ill_library_symbol', $form_state->getValue('ill_library_symbol'))->save(); |
||||
$config->set('ill_relais_key', $form_state->getValue('ill_relais_key'))->save(); |
||||
$config->set('ill_doi_openurl_pid', $form_state->getValue('ill_doi_openurl_pid'))->save(); |
||||
$config->set('ill_contact_email', $form_state->getValue('ill_contact_email'))->save(); |
||||
$config->set('ill_contact_phone', $form_state->getValue('ill_contact_phone'))->save(); |
||||
$config->set('ill_header_message', $form_state->getValue('ill_header_message'))->save(); |
||||
} |
||||
|
||||
} |
@ -0,0 +1,7 @@
|
||||
name: Roblib ILL Loan Forms |
||||
type: module |
||||
configure: upei_roblib_ill.settings |
||||
description: Provides a front end for our Inter Library Loan users. |
||||
package: Roblib ILL |
||||
core_version_requirement: ^9 |
||||
dependancies: |
@ -0,0 +1,24 @@
|
||||
roblib_ill.settings: |
||||
path: 'admin/settings/roblibill' |
||||
defaults: |
||||
_form: '\Drupal\upei_roblib_ill\Form\RoblibIllSettingsForm' |
||||
_title: 'Roblib ILL Settings Form' |
||||
requirements: |
||||
_permission: 'administer site configuration' |
||||
|
||||
roblib_ill.loan_form: |
||||
path: 'upei/roblib/ill/{parameters}' |
||||
defaults: |
||||
_form: '\Drupal\upei_roblib_ill\Form\RoblibIllLoanForm' |
||||
_title: 'Roblib ILL Loan Form' |
||||
parameters: '[a-zA-Z\s]+' |
||||
requirements: |
||||
_permission: 'access content' |
||||
|
||||
roblib_ill.loan_form_finished: |
||||
path: 'upei/roblib/ill/finished' |
||||
defaults: |
||||
_controller: '\Drupal\upei_roblib_ill\Controller\RoblibIllController::finished' |
||||
_title: 'Inter Library Loan Completed' |
||||
requirements: |
||||
_permission: 'access content' |
Loading…
Reference in new issue