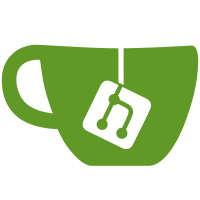
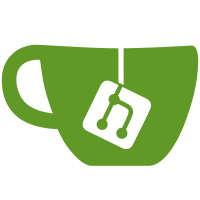
10 changed files with 739 additions and 389 deletions
@ -0,0 +1,163 @@
|
||||
#!/usr/bin/env bash |
||||
|
||||
if [ $# -lt 3 ]; then |
||||
echo "usage: $0 <db-name> <db-user> <db-pass> [db-host] [wp-version] [skip-database-creation]" |
||||
exit 1 |
||||
fi |
||||
|
||||
DB_NAME=$1 |
||||
DB_USER=$2 |
||||
DB_PASS=$3 |
||||
DB_HOST=${4-localhost} |
||||
WP_VERSION=${5-latest} |
||||
SKIP_DB_CREATE=${6-false} |
||||
|
||||
TMPDIR=${TMPDIR-/tmp} |
||||
TMPDIR=$(echo $TMPDIR | sed -e "s/\/$//") |
||||
WP_TESTS_DIR=${WP_TESTS_DIR-$TMPDIR/wordpress-tests-lib} |
||||
WP_CORE_DIR=${WP_CORE_DIR-$TMPDIR/wordpress/} |
||||
|
||||
download() { |
||||
if [ `which curl` ]; then |
||||
curl -s "$1" > "$2"; |
||||
elif [ `which wget` ]; then |
||||
wget -nv -O "$2" "$1" |
||||
fi |
||||
} |
||||
|
||||
if [[ $WP_VERSION =~ ^[0-9]+\.[0-9]+$ ]]; then |
||||
WP_TESTS_TAG="branches/$WP_VERSION" |
||||
elif [[ $WP_VERSION =~ [0-9]+\.[0-9]+\.[0-9]+ ]]; then |
||||
if [[ $WP_VERSION =~ [0-9]+\.[0-9]+\.[0] ]]; then |
||||
# version x.x.0 means the first release of the major version, so strip off the .0 and download version x.x |
||||
WP_TESTS_TAG="tags/${WP_VERSION%??}" |
||||
else |
||||
WP_TESTS_TAG="tags/$WP_VERSION" |
||||
fi |
||||
elif [[ $WP_VERSION == 'nightly' || $WP_VERSION == 'trunk' ]]; then |
||||
WP_TESTS_TAG="trunk" |
||||
else |
||||
# http serves a single offer, whereas https serves multiple. we only want one |
||||
download http://api.wordpress.org/core/version-check/1.7/ /tmp/wp-latest.json |
||||
grep '[0-9]+\.[0-9]+(\.[0-9]+)?' /tmp/wp-latest.json |
||||
LATEST_VERSION=$(grep -o '"version":"[^"]*' /tmp/wp-latest.json | sed 's/"version":"//') |
||||
if [[ -z "$LATEST_VERSION" ]]; then |
||||
echo "Latest WordPress version could not be found" |
||||
exit 1 |
||||
fi |
||||
WP_TESTS_TAG="tags/$LATEST_VERSION" |
||||
fi |
||||
|
||||
set -ex |
||||
|
||||
install_wp() { |
||||
|
||||
if [ -d $WP_CORE_DIR ]; then |
||||
return; |
||||
fi |
||||
|
||||
mkdir -p $WP_CORE_DIR |
||||
|
||||
if [[ $WP_VERSION == 'nightly' || $WP_VERSION == 'trunk' ]]; then |
||||
mkdir -p $TMPDIR/wordpress-nightly |
||||
download https://wordpress.org/nightly-builds/wordpress-latest.zip $TMPDIR/wordpress-nightly/wordpress-nightly.zip |
||||
unzip -q $TMPDIR/wordpress-nightly/wordpress-nightly.zip -d $TMPDIR/wordpress-nightly/ |
||||
mv $TMPDIR/wordpress-nightly/wordpress/* $WP_CORE_DIR |
||||
else |
||||
if [ $WP_VERSION == 'latest' ]; then |
||||
local ARCHIVE_NAME='latest' |
||||
elif [[ $WP_VERSION =~ [0-9]+\.[0-9]+ ]]; then |
||||
# https serves multiple offers, whereas http serves single. |
||||
download https://api.wordpress.org/core/version-check/1.7/ $TMPDIR/wp-latest.json |
||||
if [[ $WP_VERSION =~ [0-9]+\.[0-9]+\.[0] ]]; then |
||||
# version x.x.0 means the first release of the major version, so strip off the .0 and download version x.x |
||||
LATEST_VERSION=${WP_VERSION%??} |
||||
else |
||||
# otherwise, scan the releases and get the most up to date minor version of the major release |
||||
local VERSION_ESCAPED=`echo $WP_VERSION | sed 's/\./\\\\./g'` |
||||
LATEST_VERSION=$(grep -o '"version":"'$VERSION_ESCAPED'[^"]*' $TMPDIR/wp-latest.json | sed 's/"version":"//' | head -1) |
||||
fi |
||||
if [[ -z "$LATEST_VERSION" ]]; then |
||||
local ARCHIVE_NAME="wordpress-$WP_VERSION" |
||||
else |
||||
local ARCHIVE_NAME="wordpress-$LATEST_VERSION" |
||||
fi |
||||
else |
||||
local ARCHIVE_NAME="wordpress-$WP_VERSION" |
||||
fi |
||||
download https://wordpress.org/${ARCHIVE_NAME}.tar.gz $TMPDIR/wordpress.tar.gz |
||||
tar --strip-components=1 -zxmf $TMPDIR/wordpress.tar.gz -C $WP_CORE_DIR |
||||
fi |
||||
|
||||
download https://raw.github.com/markoheijnen/wp-mysqli/master/db.php $WP_CORE_DIR/wp-content/db.php |
||||
} |
||||
|
||||
install_pressbooks() { |
||||
if [ -d $WP_CORE_DIR/wp-content/plugins/pressbooks ]; then |
||||
cd $WP_CORE_DIR/wp-content/plugins/pressbooks && git pull |
||||
return; |
||||
fi |
||||
|
||||
git clone --depth=1 https://github.com/pressbooks/pressbooks.git $WP_CORE_DIR/wp-content/plugins/pressbooks |
||||
cd $WP_CORE_DIR/wp-content/plugins/pressbooks && composer install --no-dev --optimize-autoloader |
||||
} |
||||
|
||||
install_test_suite() { |
||||
# portable in-place argument for both GNU sed and Mac OSX sed |
||||
if [[ $(uname -s) == 'Darwin' ]]; then |
||||
local ioption='-i .bak' |
||||
else |
||||
local ioption='-i' |
||||
fi |
||||
|
||||
# set up testing suite if it doesn't yet exist |
||||
if [ ! -d $WP_TESTS_DIR ]; then |
||||
# set up testing suite |
||||
mkdir -p $WP_TESTS_DIR |
||||
svn co --quiet https://develop.svn.wordpress.org/${WP_TESTS_TAG}/tests/phpunit/includes/ $WP_TESTS_DIR/includes |
||||
svn co --quiet https://develop.svn.wordpress.org/${WP_TESTS_TAG}/tests/phpunit/data/ $WP_TESTS_DIR/data |
||||
fi |
||||
|
||||
if [ ! -f wp-tests-config.php ]; then |
||||
download https://develop.svn.wordpress.org/${WP_TESTS_TAG}/wp-tests-config-sample.php "$WP_TESTS_DIR"/wp-tests-config.php |
||||
# remove all forward slashes in the end |
||||
WP_CORE_DIR=$(echo $WP_CORE_DIR | sed "s:/\+$::") |
||||
sed $ioption "s:dirname( __FILE__ ) . '/src/':'$WP_CORE_DIR/':" "$WP_TESTS_DIR"/wp-tests-config.php |
||||
sed $ioption "s/youremptytestdbnamehere/$DB_NAME/" "$WP_TESTS_DIR"/wp-tests-config.php |
||||
sed $ioption "s/yourusernamehere/$DB_USER/" "$WP_TESTS_DIR"/wp-tests-config.php |
||||
sed $ioption "s/yourpasswordhere/$DB_PASS/" "$WP_TESTS_DIR"/wp-tests-config.php |
||||
sed $ioption "s|localhost|${DB_HOST}|" "$WP_TESTS_DIR"/wp-tests-config.php |
||||
fi |
||||
|
||||
} |
||||
|
||||
install_db() { |
||||
|
||||
if [ ${SKIP_DB_CREATE} = "true" ]; then |
||||
return 0 |
||||
fi |
||||
|
||||
# parse DB_HOST for port or socket references |
||||
local PARTS=(${DB_HOST//\:/ }) |
||||
local DB_HOSTNAME=${PARTS[0]}; |
||||
local DB_SOCK_OR_PORT=${PARTS[1]}; |
||||
local EXTRA="" |
||||
|
||||
if ! [ -z $DB_HOSTNAME ] ; then |
||||
if [ $(echo $DB_SOCK_OR_PORT | grep -e '^[0-9]\{1,\}$') ]; then |
||||
EXTRA=" --host=$DB_HOSTNAME --port=$DB_SOCK_OR_PORT --protocol=tcp" |
||||
elif ! [ -z $DB_SOCK_OR_PORT ] ; then |
||||
EXTRA=" --socket=$DB_SOCK_OR_PORT" |
||||
elif ! [ -z $DB_HOSTNAME ] ; then |
||||
EXTRA=" --host=$DB_HOSTNAME --protocol=tcp" |
||||
fi |
||||
fi |
||||
|
||||
# create database |
||||
mysqladmin create $DB_NAME --user="$DB_USER" --password="$DB_PASS"$EXTRA |
||||
} |
||||
|
||||
install_wp |
||||
install_pressbooks |
||||
install_test_suite |
||||
install_db |
@ -0,0 +1,26 @@
|
||||
codecov: |
||||
notify: |
||||
require_ci_to_pass: yes |
||||
|
||||
coverage: |
||||
precision: 2 |
||||
round: down |
||||
range: "70...100" |
||||
|
||||
status: |
||||
project: yes |
||||
patch: yes |
||||
changes: no |
||||
|
||||
parsers: |
||||
gcov: |
||||
branch_detection: |
||||
conditional: yes |
||||
loop: yes |
||||
method: no |
||||
macro: no |
||||
|
||||
comment: |
||||
layout: "header, diff" |
||||
behavior: default |
||||
require_changes: no |
@ -1,60 +0,0 @@
|
||||
<?xml version="1.0"?> |
||||
<ruleset name="WordPress Theme Coding Standards"> |
||||
<!-- See https://github.com/squizlabs/PHP_CodeSniffer/wiki/Annotated-ruleset.xml --> |
||||
<!-- See https://github.com/WordPress-Coding-Standards/WordPress-Coding-Standards --> |
||||
<!-- See https://github.com/WordPress-Coding-Standards/WordPress-Coding-Standards/wiki --> |
||||
<!-- See https://github.com/wimg/PHPCompatibility --> |
||||
|
||||
<!-- Set a description for this ruleset. --> |
||||
<description>A custom set of code standard rules to check for WordPress themes.</description> |
||||
|
||||
<!-- Pass some flags to PHPCS: |
||||
p flag: Show progress of the run. |
||||
s flag: Show sniff codes in all reports. |
||||
v flag: Print verbose output. |
||||
n flag: Do not print warnings. |
||||
--> |
||||
<arg value="psvn"/> |
||||
|
||||
<!-- Only check the PHP, CSS and SCSS files. JS files are checked separately with JSCS and JSHint. --> |
||||
<arg name="extensions" value="php,css,scss/css"/> |
||||
|
||||
<!-- Check all files in this directory and the directories below it. --> |
||||
<file>.</file> |
||||
|
||||
<!-- Include the WordPress ruleset, with exclusions. --> |
||||
<rule ref="WordPress"> |
||||
<exclude name="Generic.WhiteSpace.ScopeIndent.IncorrectExact" /> |
||||
<exclude name="Generic.WhiteSpace.ScopeIndent.Incorrect" /> |
||||
<exclude name="PEAR.Functions.FunctionCallSignature.Indent" /> |
||||
</rule> |
||||
|
||||
<!-- Verify that the text_domain is set to the desired text-domain. |
||||
Multiple valid text domains can be provided as a comma-delimited list. --> |
||||
<rule ref="WordPress.WP.I18n"> |
||||
<properties> |
||||
<property name="text_domain" type="array" value="_s" /> |
||||
</properties> |
||||
</rule> |
||||
|
||||
<!-- Allow for theme specific exceptions to the file name rules based |
||||
on the theme hierarchy. --> |
||||
<rule ref="WordPress.Files.FileName"> |
||||
<properties> |
||||
<property name="is_theme" value="true" /> |
||||
</properties> |
||||
</rule> |
||||
|
||||
<!-- Verify that no WP functions are used which are deprecated or have been removed. |
||||
The minimum version set here should be in line with the minimum WP version |
||||
as set in the "Requires at least" tag in the readme.txt file. --> |
||||
<rule ref="WordPress.WP.DeprecatedFunctions"> |
||||
<properties> |
||||
<property name="minimum_supported_version" value="4.0" /> |
||||
</properties> |
||||
</rule> |
||||
|
||||
<!-- Include sniffs for PHP cross-version compatibility. --> |
||||
<config name="testVersion" value="5.2-99.0"/> |
||||
<rule ref="PHPCompatibility"/> |
||||
</ruleset> |
@ -0,0 +1,23 @@
|
||||
<?xml version="1.0"?> |
||||
<phpunit |
||||
bootstrap="tests/bootstrap.php" |
||||
backupGlobals="false" |
||||
colors="true" |
||||
convertErrorsToExceptions="true" |
||||
convertNoticesToExceptions="true" |
||||
convertWarningsToExceptions="true" |
||||
> |
||||
<php> |
||||
<const name="WP_TESTS_MULTISITE" value="1" /> |
||||
</php> |
||||
<testsuites> |
||||
<testsuite name="Pressbooks Aldine"> |
||||
<directory prefix="test-" suffix=".php">./tests/</directory> |
||||
</testsuite> |
||||
</testsuites> |
||||
<filter> |
||||
<whitelist> |
||||
<directory suffix=".php">./inc</directory> |
||||
</whitelist> |
||||
</filter> |
||||
</phpunit> |
@ -0,0 +1,56 @@
|
||||
<?php |
||||
/** |
||||
* PHPUnit bootstrap file |
||||
* |
||||
* @package Pressbooks_Aldine |
||||
*/ |
||||
|
||||
$_tests_dir = getenv( 'WP_TESTS_DIR' ); |
||||
if ( ! $_tests_dir ) { |
||||
$_tests_dir = rtrim( sys_get_temp_dir(), '/\\' ) . '/wordpress-tests-lib'; |
||||
} |
||||
|
||||
if ( ! file_exists( $_tests_dir . '/includes/functions.php' ) ) { |
||||
throw new Exception( "Could not find $_tests_dir/includes/functions.php, have you run bin/install-wp-tests.sh ?" ); |
||||
} |
||||
|
||||
// Give access to tests_add_filter() function. |
||||
require_once $_tests_dir . '/includes/functions.php'; |
||||
|
||||
function _manually_load_plugin() { |
||||
$_pressbooks = '/tmp/wordpress/wp-content/plugins/pressbooks/pressbooks.php'; |
||||
if ( file_exists( $_pressbooks ) ) { |
||||
require_once( $_pressbooks ); |
||||
} else { |
||||
require_once( __DIR__ . '/../../../plugins/pressbooks/pressbooks.php' ); |
||||
} |
||||
} |
||||
|
||||
/** |
||||
* Registers theme |
||||
*/ |
||||
function _register_theme() { |
||||
|
||||
$theme_dir = dirname( __DIR__ ); |
||||
$current_theme = basename( $theme_dir ); |
||||
$theme_root = dirname( $theme_dir ); |
||||
|
||||
add_filter( 'theme_root', function() use ( $theme_root ) { |
||||
return $theme_root; |
||||
} ); |
||||
|
||||
register_theme_directory( $theme_root ); |
||||
|
||||
add_filter( 'pre_option_template', function() use ( $current_theme ) { |
||||
return $current_theme; |
||||
}); |
||||
add_filter( 'pre_option_stylesheet', function() use ( $current_theme ) { |
||||
return $current_theme; |
||||
}); |
||||
} |
||||
tests_add_filter( 'muplugins_loaded', '_register_theme' ); |
||||
tests_add_filter( 'muplugins_loaded', '_manually_load_plugin' ); |
||||
|
||||
|
||||
// Start up the WP testing environment. |
||||
require $_tests_dir . '/includes/bootstrap.php'; |
@ -0,0 +1,19 @@
|
||||
<?php |
||||
/** |
||||
* Class Filters |
||||
* |
||||
* @package Pressbooks_Aldine |
||||
*/ |
||||
|
||||
use function \Aldine\Filters\register_query_vars; |
||||
|
||||
/** |
||||
* Filters test case. |
||||
*/ |
||||
class FiltersTest extends WP_UnitTestCase { |
||||
public function test_register_query_vars() { |
||||
$vars = register_query_vars([]); |
||||
$this->assertContains('license', $vars); |
||||
$this->assertContains('subject', $vars); |
||||
} |
||||
} |
Loading…
Reference in new issue