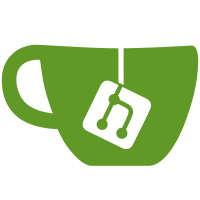
5 changed files with 123 additions and 5 deletions
@ -0,0 +1,42 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Provides the callback implementations for the service. |
||||
*/ |
||||
|
||||
/** |
||||
* The POST callback for the deletion by Fedora URI service. |
||||
* |
||||
* @param array $args |
||||
* JSON encoded arguments to delete function. |
||||
* |
||||
* @return array |
||||
* JSON encoded response message. |
||||
*/ |
||||
function islandora_delete_by_fedora_uri_service_delete(array $args) { |
||||
// Hack the Fedora path out of the POST data. |
||||
$fedora_uri = $args['fedoraUri']; |
||||
|
||||
// Get entities identified by Fedora path. |
||||
$query = new EntityFieldQuery(); |
||||
$results = $query->fieldCondition('field_fedora_path', 'value', $fedora_uri) |
||||
->execute(); |
||||
|
||||
// Delete 'em all. |
||||
foreach ($results as $entity_type => $entities) { |
||||
$ids = array_keys($entities); |
||||
if (entity_delete_multiple($entity_type, $ids) === FALSE) { |
||||
$response[] = "Could not delete any $entity_type identified by $fedora_uri."; |
||||
} |
||||
else { |
||||
$response[] = "Deleted entities of type $entity_type with ids " . implode(', ', $ids) . "."; |
||||
} |
||||
} |
||||
|
||||
if (!isset($response)) { |
||||
$response[] = "Could not find any entities identified by $fedora_uri."; |
||||
} |
||||
|
||||
return $response; |
||||
} |
@ -0,0 +1,6 @@
|
||||
name = Islandora Delete By Fedora URI Service |
||||
description = "Deletes Drupal nodes by Fedora URI. Needed for Sync to handle delete events that don't originate from Drupal." |
||||
package = Islandora |
||||
version = 7.x-dev |
||||
core = 7.x |
||||
dependencies[] = rest_server |
@ -0,0 +1,48 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Provides a service for deleting Drupal nodes by Fedora path. |
||||
*/ |
||||
|
||||
/** |
||||
* Implements hook_permission(). |
||||
*/ |
||||
function islandora_delete_by_fedora_uri_service_permission() { |
||||
return array( |
||||
'islandora delete by fedora uri' => array( |
||||
'title' => t('Delete by Fedora URI.'), |
||||
'description' => t('Allows external sources to delete Drupal nodes by Fedora URI.'), |
||||
), |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Implements hook_services_resources(). |
||||
*/ |
||||
function islandora_delete_by_fedora_uri_service_services_resources() { |
||||
return array( |
||||
'delete_by_fedora_uri' => array( |
||||
'create' => array( |
||||
'help' => t('Deletes a Drupal node by the Fedora URI supplied in the POST data.'), |
||||
'file' => array( |
||||
'type' => 'inc', |
||||
'module' => 'islandora_delete_by_fedora_uri_service', |
||||
'name' => 'include/islandora_delete_by_fedora_uri_service', |
||||
), |
||||
'callback' => 'islandora_delete_by_fedora_uri_service_delete', |
||||
'access callback' => 'user_access', |
||||
'access arguments' => array('islandora delete by fedora uri'), |
||||
'args' => array( |
||||
array( |
||||
'name' => 'args', |
||||
'type' => 'array', |
||||
'description' => t("JSON data containing arguments to the delete function (e.g. the Fedora URI)"), |
||||
'source' => 'data', |
||||
'optional' => FALSE, |
||||
), |
||||
), |
||||
), |
||||
), |
||||
); |
||||
} |
Loading…
Reference in new issue