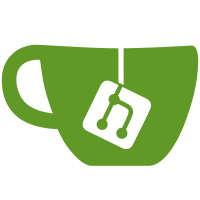
8 changed files with 708 additions and 0 deletions
@ -0,0 +1,76 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* Hashtable cache ( nothing special ) |
||||
* Should be replaced with something like |
||||
*/ |
||||
class Cache |
||||
{ |
||||
private $objectList = array(); |
||||
|
||||
/** |
||||
* Add an object to the cache |
||||
* @param ObjectModel $object |
||||
*/ |
||||
public function addObject(ObjectModel &$object) |
||||
{ |
||||
if ( !isset($this->objectList[$object->getId()])) |
||||
{ |
||||
$this->objectList[$object->getId()] = array(); |
||||
} |
||||
|
||||
$this->objectList[$object->getId()]['checksum'] = sha1(serialize($object)); |
||||
$this->objectList[$object->getId()]['object'] = $object; |
||||
} |
||||
|
||||
/** |
||||
* Check to see if the object has been updated |
||||
* @param ObjectModel $object |
||||
*/ |
||||
public function hasChanged(ObjectModel &$object) |
||||
{ |
||||
// Is the object even cached |
||||
if ( !isset($this->objectList[$object->getId()])) |
||||
{ |
||||
return false; |
||||
} |
||||
|
||||
// Does it have the same checksum |
||||
if ($this->objectList[$object->getId()]['checksum'] == sha1(serialize($object)) ) |
||||
{ |
||||
return true; |
||||
} |
||||
|
||||
// If they were the same then it would have already exited |
||||
return false; |
||||
} |
||||
|
||||
/** |
||||
* Get object from the cache. Returns null if not found. |
||||
* @param type $id |
||||
*/ |
||||
public function getObject($id) |
||||
{ |
||||
// Is the object cached |
||||
if ( isset($this->objectList[$id]['object'] ) ) { |
||||
|
||||
// Return the object from the cache |
||||
return $this->objectList[$id]['object']; |
||||
} |
||||
|
||||
// Object wasn't found so return null |
||||
return null; |
||||
} |
||||
|
||||
/** |
||||
* Remove the object from the cache |
||||
* @param type $id |
||||
*/ |
||||
public function deleteObject($id) |
||||
{ |
||||
// Unset it from the array |
||||
unset( $this->objectList[$id]); |
||||
} |
||||
} |
||||
|
||||
?> |
@ -0,0 +1,58 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* Basic config should be replaced with a specific implementation |
||||
*/ |
||||
class Configuration |
||||
{ |
||||
private $baseUrl; |
||||
private $port; |
||||
|
||||
/** |
||||
* Default constructor |
||||
* @param type $base_url |
||||
* @param type $port |
||||
*/ |
||||
public function __construct($base_url, $port) { |
||||
$this->$baseUrl = $base_url; |
||||
$this->$port = $port; |
||||
} |
||||
|
||||
/** |
||||
* Get the base url |
||||
* @return type |
||||
*/ |
||||
public function getBaseURL() |
||||
{ |
||||
return $this->$baseUrl; |
||||
} |
||||
|
||||
/** |
||||
* Set the base url |
||||
* @param type $url |
||||
*/ |
||||
public function setBaseURL($url) |
||||
{ |
||||
$this->$baseUrl = $url; |
||||
} |
||||
|
||||
/** |
||||
* Get the port |
||||
* @return type |
||||
*/ |
||||
public function getPort() |
||||
{ |
||||
return $this->$port; |
||||
} |
||||
|
||||
/** |
||||
* Set the port |
||||
* @param type $port |
||||
*/ |
||||
public function setPort($port) |
||||
{ |
||||
$this->$port = $port; |
||||
} |
||||
} |
||||
|
||||
?> |
@ -0,0 +1,37 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* Islandora wrapper class to make everything easy to access |
||||
*/ |
||||
class IslandoraModule |
||||
{ |
||||
private static $instance; |
||||
private $repository; |
||||
|
||||
/** |
||||
* Block people from creating the class |
||||
*/ |
||||
private function __construct() |
||||
{ |
||||
$this->repository = new Repository(new Configuration("127.0.0.1", 8080), new Search, new Cache()); |
||||
} |
||||
|
||||
/** |
||||
* Get the repository singleton |
||||
* @return type |
||||
*/ |
||||
public static function instance() |
||||
{ |
||||
if ( self::instance == null ) |
||||
{ |
||||
$className = __CLASS__; |
||||
self::$instance = new $className; |
||||
// get_called_class only works in 5.3 |
||||
} |
||||
|
||||
// Return the link to the repository |
||||
return self::$instance->repository; |
||||
} |
||||
} |
||||
|
||||
?> |
@ -0,0 +1,264 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* Fedora repository |
||||
*/ |
||||
class Repository |
||||
{ |
||||
private $config; |
||||
private $search; |
||||
private $cache; |
||||
|
||||
public function __construct(Configuration &$config, Search &$search, Cache &$cache) |
||||
{ |
||||
// Store all the dependencies |
||||
$this->setConfig($config); |
||||
$this->setSearch($search); |
||||
$this->setCache($cache); |
||||
} |
||||
|
||||
/** |
||||
* Get the configuration implementation |
||||
* @return type |
||||
*/ |
||||
public function getConfig() |
||||
{ |
||||
return $this->config; |
||||
} |
||||
|
||||
/** |
||||
* Get the search implementation |
||||
* @return type |
||||
*/ |
||||
public function getSearch() |
||||
{ |
||||
return $this->search; |
||||
} |
||||
|
||||
/** |
||||
* Get the cache implementation |
||||
* @return type |
||||
*/ |
||||
public function getCache() |
||||
{ |
||||
return $this->cache; |
||||
} |
||||
|
||||
/** |
||||
* Set the configuration implementation |
||||
* @param Configuration $config |
||||
*/ |
||||
public function setConfig(Configuration &$config) |
||||
{ |
||||
if ( $config == null ) |
||||
{ |
||||
throw new Exception("Config implementation can't be null"); |
||||
} |
||||
$this->config = $config; |
||||
} |
||||
|
||||
/** |
||||
* Set the search implementation |
||||
* @param Search $search |
||||
*/ |
||||
public function setSearch(Search &$search) |
||||
{ |
||||
if ( $search == null ) |
||||
{ |
||||
throw new Exception("Search implementation can't be null"); |
||||
} |
||||
$this->search = $search; |
||||
} |
||||
|
||||
/** |
||||
* Set the cache implementation |
||||
* @param Cache $cache |
||||
*/ |
||||
public function setCache(Cache &$cache) |
||||
{ |
||||
if ( $cache == null ) |
||||
{ |
||||
throw new Exception("Cache implementation can't be null"); |
||||
} |
||||
$this->cache = $cache; |
||||
} |
||||
|
||||
/** |
||||
* Get the object from the repo |
||||
* @param type $id |
||||
*/ |
||||
public function loadObject($id, $cache=true) |
||||
{ |
||||
// Check to see if its already cached |
||||
if ( $this->getCache()->getObject( $id ) && $cache = true) |
||||
{ |
||||
// Return the cached object |
||||
return $this->getCache()->getObject( $id ); |
||||
} |
||||
|
||||
// Create the request |
||||
$results = $this->makeRequest( '/objects/' .$id ); |
||||
|
||||
// Return the object model |
||||
return unserialize($results); |
||||
} |
||||
|
||||
/** |
||||
* Save an object to the repository |
||||
* @param ObjectModel $model |
||||
* @param type $force |
||||
*/ |
||||
public function saveObject(ObjectModel $model, $force=false) |
||||
{ |
||||
// Has the object been created at all |
||||
if ( $model->getId() == null ) { |
||||
|
||||
// Get the next free persistent id |
||||
$id = $this->makeRequest( '/objects/nextPID' ); |
||||
|
||||
// Set the id on the model |
||||
$model->setId($id); |
||||
|
||||
} |
||||
|
||||
// If it hasn't changed then done't save unless it's forced |
||||
if ( ! $force && ! $this->getCache()->hasChanged($model) ) |
||||
{ |
||||
return; |
||||
} |
||||
|
||||
// Add the object to the cache so everybody has the new copy |
||||
$this->getCache()->addObject($model); |
||||
|
||||
// Post the serialized model to the object endpoint |
||||
$this->makeRequest('/objects', $this->serialize($model)); |
||||
} |
||||
|
||||
/** |
||||
* Find an object using a search term |
||||
* @param type $term |
||||
* @return type |
||||
*/ |
||||
public function findObjectByTerm($term) |
||||
{ |
||||
// Create results |
||||
$results = $this->makeRequest('/objects?terms=' . $term); |
||||
|
||||
// Do something with results |
||||
return $results; |
||||
} |
||||
|
||||
/** |
||||
* Find an object with a query |
||||
* @param type $query |
||||
* @return type |
||||
*/ |
||||
public function findObjectWithQuery($query) |
||||
{ |
||||
// Create results |
||||
$results = $this->makeRequest('/objects?query=' . $term); |
||||
|
||||
// Do something with results |
||||
return $results; |
||||
} |
||||
|
||||
/** |
||||
* Search the repository using either a SPO or an array of SPOs |
||||
* @param type $query |
||||
*/ |
||||
public function SearchSPO($SPO) |
||||
{ |
||||
// Search |
||||
$results = $this->getSearch()->SearchSPO($SPO); |
||||
|
||||
// Do something with the results |
||||
return $results; |
||||
} |
||||
|
||||
/** |
||||
* Unserialize the object from foxml |
||||
* @param type $xml |
||||
* @return \ObjectModel |
||||
*/ |
||||
protected function unserialize($xml) |
||||
{ |
||||
// Create the object model |
||||
$model = new ObjectModel(); |
||||
|
||||
// Do something with the xml |
||||
$xml = $xml; |
||||
|
||||
// Return the model; |
||||
return $model; |
||||
} |
||||
|
||||
/** |
||||
* Serialize the object to foxml |
||||
* @param ObjectModel $model |
||||
* @return string |
||||
*/ |
||||
protected function serialize(ObjectModel $model) |
||||
{ |
||||
// Do something with the model |
||||
serialize($model); |
||||
|
||||
// return a string |
||||
return ""; |
||||
} |
||||
|
||||
/** |
||||
* Make a request |
||||
* @param type $request |
||||
* @return type |
||||
*/ |
||||
private function makeRequest($request, array $postData = null, $responseCode=200, $format="xml") |
||||
{ |
||||
// Check to see if we already have parameters |
||||
$pos = strpos($request, "?"); |
||||
if ($pos === false) { |
||||
$request.="?format=".$format; |
||||
} else { |
||||
$request.="&format=".$format; |
||||
} |
||||
|
||||
// Initialize Curl |
||||
$curl = curl_init(); |
||||
|
||||
// Set all the options |
||||
curl_setopt($curl, CURLOPT_URL, $this->getConfig()->getBaseURL() . $request); |
||||
curl_setopt($curl, CURLOPT_PORT , $this->getConfig()->getPort() ); |
||||
curl_setopt($curl, CURLOPT_VERBOSE, 1); |
||||
// If we have post data then append that |
||||
if ( $postData ) { |
||||
curl_setopt($curl, CURLOPT_POSTFIELDS, $postData); |
||||
} |
||||
|
||||
// Execute the curl call |
||||
$results = curl_exec($curl); |
||||
|
||||
// Check for an error |
||||
if( ! curl_errno($curl) ) { |
||||
|
||||
// Get information regarding the curl connection |
||||
$info = curl_getinfo($curl); |
||||
|
||||
// Check the response code |
||||
if ( $info['http_code'] != $responseCode ) |
||||
{ |
||||
var_dump($results); |
||||
var_dump($info); |
||||
throw new Exception("Curl request failed"); |
||||
} else { |
||||
return $results; |
||||
} |
||||
} |
||||
|
||||
// Close connection |
||||
curl_close($curl); |
||||
|
||||
} // Close makeRequest |
||||
|
||||
} |
||||
|
||||
|
||||
?> |
@ -0,0 +1,37 @@
|
||||
<?php |
||||
|
||||
|
||||
/** |
||||
* Basic search that uses RI but could be replaced with solr |
||||
*/ |
||||
class Search |
||||
{ |
||||
/** |
||||
* Search the repository using either a SPO or an array of SPOs |
||||
* @param type $query |
||||
*/ |
||||
public function SearchSPO($SPO) |
||||
{ |
||||
$queryString = ""; |
||||
if (is_array($SPO)) |
||||
{ |
||||
foreach($SPO as $spo) |
||||
{ |
||||
$queryString .= $spo->getSubject() . " " . $spo->getPredicate() . " " . $spo->getObject() . ", "; |
||||
} |
||||
// Strip off the extra comma |
||||
$queryString = substr($queryString, '', -2); |
||||
} |
||||
else { |
||||
$queryString .= $SPO->getSubject() . " " . $SPO->getPredicate() . " " . $SPO->getObject(); |
||||
} |
||||
|
||||
// Do a search |
||||
$results = ""; |
||||
|
||||
// Return results; |
||||
return $results; |
||||
} |
||||
} |
||||
|
||||
?> |
@ -0,0 +1,42 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* Repository Object Data |
||||
*/ |
||||
class DataObject |
||||
{ |
||||
private $id; |
||||
|
||||
/** |
||||
* Get the object id |
||||
* @return type |
||||
*/ |
||||
public function getId() |
||||
{ |
||||
return $this->id; |
||||
} |
||||
|
||||
/** |
||||
* Set the idea if not already set |
||||
* @param type $id |
||||
* @throws Exception |
||||
*/ |
||||
public function setId($id) |
||||
{ |
||||
if ( $pid != null ) |
||||
{ |
||||
throw new Exception("PID can't be changed"); |
||||
} |
||||
$this->id = $pid; |
||||
} |
||||
|
||||
/** |
||||
* Add the data from a file ( example ) |
||||
*/ |
||||
public function addDataFromFile() |
||||
{ |
||||
|
||||
} |
||||
} |
||||
|
||||
?> |
@ -0,0 +1,119 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* Repository Object |
||||
* |
||||
* @todo Create an iterator for the datas |
||||
*/ |
||||
class ObjectModel |
||||
{ |
||||
private $id; |
||||
private $label; |
||||
private $status; |
||||
private $dataObjects = array(); |
||||
|
||||
/** |
||||
* Get the object id |
||||
* @return type |
||||
*/ |
||||
public function getId() |
||||
{ |
||||
return $this->id; |
||||
} |
||||
|
||||
/** |
||||
* Set the idea if not already set |
||||
* @param type $id |
||||
* @throws Exception |
||||
*/ |
||||
public function setId($id) |
||||
{ |
||||
if ( $id != null ) |
||||
{ |
||||
throw new Exception("ID can't be changed"); |
||||
} |
||||
$this->id = $id; |
||||
} |
||||
|
||||
/** |
||||
* Get the object label |
||||
* @return type |
||||
*/ |
||||
public function getLabel() |
||||
{ |
||||
return $this->label; |
||||
} |
||||
|
||||
/** |
||||
* Set the label |
||||
* @param type $label |
||||
*/ |
||||
public function setLabel($label) |
||||
{ |
||||
$this->label = $label; |
||||
} |
||||
|
||||
/** |
||||
* Get the object status |
||||
* @return type |
||||
*/ |
||||
public function getStatus() |
||||
{ |
||||
return $this->status; |
||||
} |
||||
|
||||
/** |
||||
* Set the status |
||||
* @param type $status |
||||
*/ |
||||
public function setStatus($status) |
||||
{ |
||||
$this->label = $status; |
||||
} |
||||
|
||||
/** |
||||
* Get a data using the id |
||||
* @param type $id |
||||
* @return null |
||||
*/ |
||||
public function getData($id) |
||||
{ |
||||
foreach($this->getAllDataObjects() as $data) |
||||
{ |
||||
if ($data->getId() == $id) |
||||
{ |
||||
return $data; |
||||
} |
||||
} |
||||
return null; |
||||
} |
||||
|
||||
/** |
||||
* Get all the data object |
||||
* @return type |
||||
*/ |
||||
public function getAllDataObjects() |
||||
{ |
||||
return $this->dataObjects; |
||||
} |
||||
|
||||
/** |
||||
* Add a data object |
||||
* @param type $data |
||||
*/ |
||||
public function addData(DataObject &$data) |
||||
{ |
||||
$this->dataObjects[$data->getId()] = $data; |
||||
} |
||||
|
||||
/** |
||||
* Delete a data object |
||||
* @param type $data |
||||
*/ |
||||
public function deleteData(DataObject &$data) |
||||
{ |
||||
unset( $this->datas[$data->getId()] ); |
||||
} |
||||
} |
||||
|
||||
?> |
@ -0,0 +1,75 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* Subject Predicate Object |
||||
* I.E. This, has, that |
||||
*/ |
||||
class SPO |
||||
{ |
||||
private $subject; |
||||
private $predicate; |
||||
private $object; |
||||
|
||||
public function __construct($subject, $predicate, $object) |
||||
{ |
||||
$this->subject = $subject; |
||||
$this->predicate = $predicate; |
||||
$this->object = $object; |
||||
} |
||||
|
||||
/** |
||||
* Get the subject |
||||
* @return type |
||||
*/ |
||||
public function getSubject() |
||||
{ |
||||
return $this->subject; |
||||
} |
||||
|
||||
/** |
||||
* Get the predicate |
||||
* @return type |
||||
*/ |
||||
public function getPredicate() |
||||
{ |
||||
return $this->predicate; |
||||
} |
||||
|
||||
/** |
||||
* Get the object |
||||
* @return type |
||||
*/ |
||||
public function getObject() |
||||
{ |
||||
return $this->object; |
||||
} |
||||
|
||||
/** |
||||
* Set the subject |
||||
* @param type $value |
||||
*/ |
||||
public function setSubject($value) |
||||
{ |
||||
$this->subject = $value; |
||||
} |
||||
|
||||
/** |
||||
* Set the predicate |
||||
* @param type $value |
||||
*/ |
||||
public function setPredicate($value) |
||||
{ |
||||
$this->predicate = $value; |
||||
} |
||||
|
||||
/** |
||||
* Set the object |
||||
* @param type $value |
||||
*/ |
||||
public function setObject($value) |
||||
{ |
||||
$this->object = $value; |
||||
} |
||||
} |
||||
|
||||
?> |
Loading…
Reference in new issue