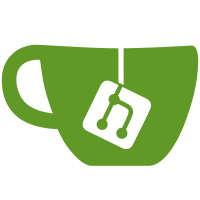
7 changed files with 344 additions and 11 deletions
@ -1,4 +1,4 @@
|
||||
You can define your own configurations specific to your enviroment by copying |
||||
default.test_config.ini to test_config.ini, making your changes in the copied |
||||
file. These test need write access to the system's $FEDORA_HOME/server/config |
||||
file. These test need write access to the system's $FEDORA_HOME/server/config |
||||
directory as well as the filter-drupal.xml file. |
@ -0,0 +1,118 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Tests to see if the hooks get called when appropriate. |
||||
* |
||||
* In the test module 'islandora_hooks_test' there are implementations |
||||
* of hooks being tested. These implementations modifies the session, and |
||||
* that's how we test if the hook gets called. |
||||
* |
||||
* To make sense of these tests reference islandora_hooks_test.module. |
||||
*/ |
||||
|
||||
class IslandoraIngestsTestCase extends IslandoraWebTestCase { |
||||
|
||||
/** |
||||
* Gets info to display to describe this test. |
||||
* |
||||
* @see IslandoraWebTestCase::getInfo() |
||||
*/ |
||||
public static function getInfo() { |
||||
return array( |
||||
'name' => 'Islandora Ingestion', |
||||
'description' => 'Ensure that the ingest forms function correctly.', |
||||
'group' => 'Islandora', |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Creates an admin user and a connection to a fedora repository. |
||||
* |
||||
* @see IslandoraWebTestCase::setUp() |
||||
*/ |
||||
public function setUp() { |
||||
parent::setUp('islandora_ingest_test'); |
||||
$this->repository = $this->admin->repository; |
||||
$this->purgeTestObjects(); |
||||
|
||||
} |
||||
|
||||
/** |
||||
* Free any objects/resources created for this test. |
||||
* |
||||
* @see IslandoraWebTestCase::tearDown() |
||||
*/ |
||||
public function tearDown() { |
||||
$this->purgeTestObjects(); |
||||
unset($this->repository); |
||||
parent::tearDown(); |
||||
} |
||||
|
||||
/** |
||||
* Purge any objects created by the test's in this class. |
||||
*/ |
||||
public function purgeTestObjects() { |
||||
$objects = array( |
||||
'test:test', |
||||
); |
||||
foreach ($objects as $object) { |
||||
try { |
||||
$object = $this->repository->getObject($object); |
||||
$this->repository->purgeObject($object->id); |
||||
} |
||||
catch (Exception $e) { |
||||
// Meh... Either it didn't exist or the purge failed. |
||||
} |
||||
} |
||||
} |
||||
|
||||
/** |
||||
* Test Ingest Steps. |
||||
*/ |
||||
public function testIngest() { |
||||
// Login the Admin user. |
||||
$this->drupalLogin($this->admin); |
||||
// First step in form. |
||||
$this->drupalGet('test/ingest'); |
||||
// Default model selected, has no additional steps. |
||||
$this->assertFieldByName('ingest', 'Ingest'); |
||||
// Select a model with additional steps. |
||||
$edit = array( |
||||
'model' => 'test:testcmodel', |
||||
); |
||||
$this->drupalPostAJAX(NULL, $edit, 'model'); |
||||
// Form now reflexts multiple steps. |
||||
$this->assertFieldByName('label', ''); |
||||
$this->assertFieldByName('next', 'Next'); |
||||
// Move to next step. |
||||
$edit = array( |
||||
'label' => 'foobar', |
||||
'model' => 'test:testcmodel', |
||||
); |
||||
$this->drupalPost(NULL, $edit, t('Next')); |
||||
$this->assertFieldByName('form_step_id', 'islandora_ingest_test_testcmodel'); |
||||
$this->assertFieldByName('ingest', 'Ingest'); |
||||
// Move back to first step. |
||||
$this->drupalPost(NULL, array(), t('Previous')); |
||||
// Try a different model that has an additional step. |
||||
$edit = array( |
||||
'model' => 'test:testcmodel2', |
||||
); |
||||
$this->drupalPostAJAX(NULL, $edit, 'model'); |
||||
$edit = array( |
||||
'label' => 'foobar', |
||||
'model' => 'test:testcmodel2', |
||||
); |
||||
$this->drupalPost(NULL, $edit, t('Next')); |
||||
$this->assertFieldByName('form_step_id', 'islandora_ingest_test_testcmodel2'); |
||||
$this->assertFieldByName('ingest', 'Ingest'); |
||||
// Ingest the thing. |
||||
$this->drupalPost(NULL, array(), t('Ingest')); |
||||
// Test that the thing got made. |
||||
$object = islandora_object_load('test:test'); |
||||
$this->assertEqual($object->label, 'foobar', 'Ingest Object matches submitted form values.'); |
||||
$this->assertEqual($object->models, array('test:testcmodel2', 'fedora-system:FedoraObject-3.0'), 'Ingest Object matches submitted form values.'); |
||||
} |
||||
|
||||
} |
@ -0,0 +1,7 @@
|
||||
name = Islandora Testing |
||||
description = Required for testing islandora web-test case. |
||||
core = 7.x |
||||
package = Testing |
||||
hidden = TRUE |
||||
dependencies[] = islandora |
||||
files[] = islandora_test.module |
@ -0,0 +1,166 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Implements hooks that get tested by islandora_hooks.test |
||||
*/ |
||||
|
||||
/** |
||||
* Implements hook menu. |
||||
*/ |
||||
function islandora_ingest_test_menu() { |
||||
return array( |
||||
'test/ingest' => array( |
||||
'title' => 'Ingest Form', |
||||
'page callback' => 'islandora_ingest_test_ingest', |
||||
'type' => MENU_LOCAL_ACTION, |
||||
'access callback' => TRUE, |
||||
), |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Render the ingest form. |
||||
*/ |
||||
function islandora_ingest_test_ingest() { |
||||
$connection = islandora_get_tuque_connection(); |
||||
$object = $connection->repository->constructObject('test:test'); |
||||
$object->label = 'New Object'; |
||||
$configuration = array( |
||||
'objects' => array($object), |
||||
); |
||||
module_load_include('inc', 'islandora', 'includes/ingest.form'); |
||||
return drupal_get_form('islandora_ingest_form', $configuration); |
||||
} |
||||
|
||||
/** |
||||
* Implements hook_islandora_ingest_steps(). |
||||
*/ |
||||
function islandora_ingest_test_islandora_ingest_steps(array &$form_state) { |
||||
return array( |
||||
'islandora_ingest_test' => array( |
||||
'type' => 'form', |
||||
'form_id' => 'islandora_ingest_test_set_label_form', |
||||
'weight' => -50, |
||||
'module' => 'islandora_ingest_test', |
||||
), |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Implements hook_MODEL_PID_islandora_ingest_steps(). |
||||
*/ |
||||
function islandora_ingest_test_test_testcmodel_islandora_ingest_steps(array &$form_state) { |
||||
return array( |
||||
'islandora_ingest_test_testcmodel' => array( |
||||
'type' => 'form', |
||||
'form_id' => 'islandora_ingest_test_testcmodel_form', |
||||
'weight' => -30, |
||||
'module' => 'islandora_ingest_test', |
||||
), |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Implements hook_MODEL_PID_islandora_ingest_steps(). |
||||
*/ |
||||
function islandora_ingest_test_test_testcmodel2_islandora_ingest_steps(array &$form_state) { |
||||
return array( |
||||
'islandora_ingest_test_testcmodel2' => array( |
||||
'type' => 'form', |
||||
'form_id' => 'islandora_ingest_test_testcmodel2_form', |
||||
'weight' => -40, |
||||
'module' => 'islandora_ingest_test', |
||||
), |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Sets the label of the ingestable object. |
||||
* |
||||
* @param array $form |
||||
* The Drupal form. |
||||
* @param array $form_state |
||||
* The Drupal form state. |
||||
* |
||||
* @return array |
||||
* The Drupal form definition. |
||||
*/ |
||||
function islandora_ingest_test_set_label_form(array $form, array &$form_state) { |
||||
$models = array('test:nomorestepscmodel', 'test:testcmodel', 'test:testcmodel2'); |
||||
$model = isset($form_state['values']['model']) ? $form_state['values']['model'] : reset($models); |
||||
$shared_storage = &islandora_ingest_form_get_shared_storage($form_state); |
||||
$shared_storage['models'] = array($model); |
||||
return array( |
||||
'#prefix' => '<div id="islandora-select-content-model-wrapper">', |
||||
'#suffix' => '</div>', |
||||
'label' => array( |
||||
'#type' => 'textfield', |
||||
'#title' => t('Label'), |
||||
), |
||||
'model' => array( |
||||
'#type' => 'select', |
||||
'#title' => t('Select a Content Model to Ingest'), |
||||
'#options' => array_combine($models, $models), |
||||
'#default' => $model, |
||||
'#ajax' => array( |
||||
'callback' => 'islandora_ingest_test_model_ajax_callback', |
||||
'wrapper' => 'islandora-select-content-model-wrapper', |
||||
'method' => 'replace', |
||||
'effect' => 'fade', |
||||
), |
||||
), |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Updates the model field. |
||||
*/ |
||||
function islandora_ingest_test_model_ajax_callback(array $form, array &$form_state) { |
||||
return $form; |
||||
} |
||||
|
||||
/** |
||||
* Sets the label of the ingestable object. |
||||
* |
||||
* @param array $form |
||||
* The Drupal form. |
||||
* @param array $form_state |
||||
* The Drupal form state. |
||||
*/ |
||||
function islandora_ingest_test_set_label_form_submit(array $form, array &$form_state) { |
||||
$object = islandora_ingest_form_get_object($form_state); |
||||
$object->label = $form_state['values']['label']; |
||||
unset($object->models); |
||||
$object->models = array($form_state['values']['model']); |
||||
} |
||||
|
||||
/** |
||||
* Test the First content model. |
||||
*/ |
||||
function islandora_ingest_test_testcmodel_form(array $form, array &$form_state) { |
||||
return array( |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Test the CModel submit. |
||||
*/ |
||||
function islandora_ingest_test_testcmodel_form_submit(array $form, array &$form_state) { |
||||
|
||||
} |
||||
|
||||
/** |
||||
* Test the second content model. |
||||
*/ |
||||
function islandora_ingest_test_testcmodel2_form(array $form, array &$form_state) { |
||||
return array( |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Test the CModel submit. |
||||
*/ |
||||
function islandora_ingest_test_testcmodel2_form_submit(array $form, array &$form_state) { |
||||
|
||||
} |
Loading…
Reference in new issue