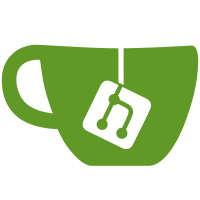
2 changed files with 100 additions and 0 deletions
@ -0,0 +1,88 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Drupal\islandora\Plugin\views\filter; |
||||||
|
|
||||||
|
use Drupal\Core\Form\FormStateInterface; |
||||||
|
use Drupal\views\Plugin\views\filter\FilterPluginBase; |
||||||
|
|
||||||
|
/** |
||||||
|
* Views Filter on Having Media of a Type. |
||||||
|
* |
||||||
|
* @ingroup views_field_handlers |
||||||
|
* |
||||||
|
* @ViewsFilter("islandora_node_has_media_use") |
||||||
|
*/ |
||||||
|
class NodeHasMediaUse extends FilterPluginBase { |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
protected function defineOptions() { |
||||||
|
$options = parent::defineOptions(); |
||||||
|
|
||||||
|
$options['use_uri'] = ['default' => NULL]; |
||||||
|
$options['negated'] = ['default' => FALSE]; |
||||||
|
|
||||||
|
return $options; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function validateOptionsForm(&$form, FormStateInterface $form_state) { |
||||||
|
parent::validateOptionsForm($form, $form_state); |
||||||
|
|
||||||
|
$uri = $form_state->getValues()['options']['use_uri']; |
||||||
|
$term = \Drupal::service('islandora.utils')->getTermForUri($uri); |
||||||
|
if (empty($term)) { |
||||||
|
$form_state->setError($form['use_uri'], $this->t('Could not find term with URI: "%uri"', ['%uri' => $uri])); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
protected function valueForm(&$form, FormStateInterface $form_state) { |
||||||
|
$form['use_uri'] = [ |
||||||
|
'#type' => 'textfield', |
||||||
|
'#title' => "Media Use URI", |
||||||
|
'#default_value' => $this->options['use_uri'], |
||||||
|
'#required' => TRUE, |
||||||
|
]; |
||||||
|
$form['negated'] = [ |
||||||
|
'#type' => 'checkbox', |
||||||
|
'#title' => 'Negated', |
||||||
|
'#description' => $this->t("Return nodes that <em>don't</em> have this use URI"), |
||||||
|
'#default_value' => $this->options['negated'], |
||||||
|
]; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function adminSummary() { |
||||||
|
$operator = ($this->options['negated']) ? "does not have" : "has"; |
||||||
|
return "Node {$operator} media with use: '{$this->options['use_uri']}'"; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function query() { |
||||||
|
$condition = ($this->options['negated']) ? 'NOT IN' : 'IN'; |
||||||
|
$utils = \Drupal::service('islandora.utils'); |
||||||
|
$term = $utils->getTermForUri($this->options['use_uri']); |
||||||
|
if (empty($term)) { |
||||||
|
\Drupal::logger('islandora')->warning('Node Has Media Filter could not find term with URI: "%uri"', ['%uri' => $this->options['use_uri']]); |
||||||
|
return; |
||||||
|
} |
||||||
|
$sub_query = \Drupal::database()->select('media', 'm'); |
||||||
|
$sub_query->join('media__field_media_use', 'use', 'm.mid = use.entity_id'); |
||||||
|
$sub_query->join('media__field_media_of', 'of', 'm.mid = of.entity_id'); |
||||||
|
$sub_query->fields('of', ['field_media_of_target_id']) |
||||||
|
->condition('use.field_media_use_target_id', $term->id()); |
||||||
|
$this->query->addWhere(0, 'nid', $sub_query, $condition); |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
} |
Loading…
Reference in new issue