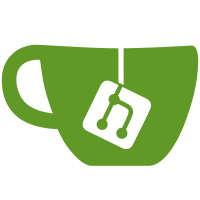
8 changed files with 213 additions and 240 deletions
@ -0,0 +1,97 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\islandora_advanced_search\Plugin\Block; |
||||
|
||||
use Drupal\Component\Plugin\PluginBase; |
||||
use Drupal\Core\Plugin\Discovery\ContainerDeriverInterface; |
||||
use Drupal\Core\StringTranslation\StringTranslationTrait; |
||||
use Symfony\Component\DependencyInjection\ContainerInterface; |
||||
|
||||
/** |
||||
* This deriver creates a block for every search_api.display. |
||||
*/ |
||||
abstract class SearchApiDisplayBlockDeriver implements ContainerDeriverInterface { |
||||
|
||||
use StringTranslationTrait; |
||||
|
||||
/** |
||||
* List of derivative definitions. |
||||
* |
||||
* @var array |
||||
*/ |
||||
protected $derivatives = []; |
||||
|
||||
/** |
||||
* The entity storage for the view. |
||||
* |
||||
* @var \Drupal\Core\Entity\EntityStorageInterface |
||||
*/ |
||||
protected $storage; |
||||
|
||||
/** |
||||
* The display manager for the search_api. |
||||
* |
||||
* @var \Drupal\search_api\Display\DisplayPluginManager |
||||
*/ |
||||
protected $displayPluginManager; |
||||
|
||||
/** |
||||
* Label for the SearchApiDisplayBlockDriver. |
||||
*/ |
||||
abstract protected function label(); |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public static function create(ContainerInterface $container, $base_plugin_id) { |
||||
$deriver = new static($container, $base_plugin_id); |
||||
$deriver->storage = $container->get('entity_type.manager')->getStorage('view'); |
||||
$deriver->displayPluginManager = $container->get('plugin.manager.search_api.display'); |
||||
return $deriver; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function getDerivativeDefinition($derivative_id, $base_plugin_definition) { |
||||
$derivatives = $this->getDerivativeDefinitions($base_plugin_definition); |
||||
return isset($derivatives[$derivative_id]) ? $derivatives[$derivative_id] : NULL; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function getDerivativeDefinitions($base_plugin_definition) { |
||||
$base_plugin_id = $base_plugin_definition['id']; |
||||
|
||||
if (!isset($this->derivatives[$base_plugin_id])) { |
||||
$plugin_derivatives = []; |
||||
|
||||
foreach ($this->displayPluginManager->getDefinitions() as $display_definition) { |
||||
$view_id = $display_definition['view_id']; |
||||
$view_display = $display_definition['view_display']; |
||||
// The derived block needs both the view / display identifiers to |
||||
// construct the pager. |
||||
$machine_name = "${view_id}__${view_display}"; |
||||
|
||||
/** @var \Drupal\views\ViewEntityInterface $view */ |
||||
$view = $this->storage->load($view_id); |
||||
$display = $view->getDisplay($view_display); |
||||
|
||||
$plugin_derivatives[$machine_name] = [ |
||||
'id' => $base_plugin_id . PluginBase::DERIVATIVE_SEPARATOR . $machine_name, |
||||
'label' => $this->label(), |
||||
'admin_label' => $this->t(':view: :label for :display', [ |
||||
':view' => $view->label(), |
||||
':label' => $this->label(), |
||||
':display' => $display['display_title'], |
||||
]), |
||||
] + $base_plugin_definition; |
||||
} |
||||
|
||||
$this->derivatives[$base_plugin_id] = $plugin_derivatives; |
||||
} |
||||
return $this->derivatives[$base_plugin_id]; |
||||
} |
||||
|
||||
} |
@ -0,0 +1,67 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\islandora_advanced_search\Plugin\facets_summary\processor; |
||||
|
||||
use Drupal\Core\Link; |
||||
use Drupal\Core\Url; |
||||
use Drupal\facets\FacetInterface; |
||||
|
||||
/** |
||||
* Common logic to toggle the display of facets given a condition. |
||||
*/ |
||||
trait ShowFacetsTrait { |
||||
|
||||
/** |
||||
* Checks if the facet should be shown or not. |
||||
*/ |
||||
abstract protected function condition(FacetInterface $facet); |
||||
|
||||
/** |
||||
* Classes to include on the shown facet. |
||||
*/ |
||||
abstract protected function classes(); |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
protected function buildHelper(array $build, array $facets) { |
||||
$request = \Drupal::request(); |
||||
$query_params = $request->query->all(); |
||||
foreach ($facets as $facet) { |
||||
if ($this->condition($facet)) { |
||||
$url_alias = $facet->getUrlAlias(); |
||||
$filter_key = $facet->getFacetSourceConfig()->getFilterKey() ?: 'f'; |
||||
$active_items = $facet->getActiveItems(); |
||||
foreach ($active_items as $active_item) { |
||||
$url = Url::createFromRequest($request); |
||||
$modified_query_params = $query_params; |
||||
$modified_query_params[$filter_key] = array_filter($query_params[$filter_key], function ($query_param) use ($url_alias, $active_item) { |
||||
$pos = strpos($query_param, ':'); |
||||
$alias = substr($query_param, 0, $pos); |
||||
$value = substr($query_param, $pos + 1); |
||||
return !($alias == $url_alias && $value == $active_item); |
||||
}); |
||||
$url->setOption('query', $modified_query_params); |
||||
$item = [ |
||||
'#theme' => 'facets_result_item__summary', |
||||
'#value' => $active_item, |
||||
// We do not have counts for excluded/missing facets... |
||||
'#show_count' => FALSE, |
||||
// Do not know the count. |
||||
'#count' => 0, |
||||
'#is_active' => TRUE, |
||||
'#facet' => $facet, |
||||
'#raw_value' => $active_item, |
||||
]; |
||||
$item = (new Link($item, $url))->toRenderable(); |
||||
$item['#wrapper_attributes'] = [ |
||||
'class' => $this->classes(), |
||||
]; |
||||
$build['#items'][] = $item; |
||||
} |
||||
} |
||||
} |
||||
return $build; |
||||
} |
||||
|
||||
} |
Loading…
Reference in new issue