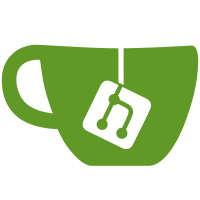
5 changed files with 200 additions and 54 deletions
@ -0,0 +1,71 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
/** |
||||||
|
* @file |
||||||
|
* Utility functions for defining collection bundles. |
||||||
|
*/ |
||||||
|
|
||||||
|
/** |
||||||
|
* Defines bundles (content types) for this module. |
||||||
|
*/ |
||||||
|
function islandora_collection_define_bundles() { |
||||||
|
module_load_include('inc', 'islandora_dcterms', 'include/fields'); |
||||||
|
|
||||||
|
$t = get_t(); |
||||||
|
|
||||||
|
// Create basic_image bundle. |
||||||
|
$collection_content_type = array( |
||||||
|
'type' => ISLANDORA_COLLECTION_BUNDLE, |
||||||
|
'name' => $t('Collection'), |
||||||
|
'base' => 'node_content', |
||||||
|
'description' => $t('A collection in Fedora'), |
||||||
|
'custom' => 1, |
||||||
|
'modified' => 1, |
||||||
|
'locked' => 0, |
||||||
|
'disabled' => 0, |
||||||
|
); |
||||||
|
|
||||||
|
$collection_content_type = node_type_set_defaults($collection_content_type); |
||||||
|
|
||||||
|
$status = node_type_save($collection_content_type); |
||||||
|
|
||||||
|
$t_args = array('%name' => $collection_content_type->name); |
||||||
|
|
||||||
|
if ($status == SAVED_UPDATED) { |
||||||
|
drupal_set_message($t('Updated %name content type.', $t_args)); |
||||||
|
} |
||||||
|
elseif ($status == SAVED_NEW) { |
||||||
|
drupal_set_message($t('Created %name content type.', $t_args)); |
||||||
|
} |
||||||
|
|
||||||
|
// Add thumbnail field. |
||||||
|
if (field_info_field(ISLANDORA_TN_FIELD)) { |
||||||
|
$tn_field_instance = array( |
||||||
|
'field_name' => ISLANDORA_TN_FIELD, |
||||||
|
'entity_type' => 'node', |
||||||
|
'bundle' => ISLANDORA_COLLECTION_BUNDLE, |
||||||
|
'label' => $t("Thumbnail"), |
||||||
|
'description' => $t("A thumbnail for the Fedora resource"), |
||||||
|
'required' => FALSE, |
||||||
|
); |
||||||
|
field_create_instance($tn_field_instance); |
||||||
|
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_TN_FIELD))); |
||||||
|
} |
||||||
|
|
||||||
|
// Add MODS field. |
||||||
|
if (field_info_field(ISLANDORA_MODS_FIELD)) { |
||||||
|
$mods_field_instance = array( |
||||||
|
'field_name' => ISLANDORA_MODS_FIELD, |
||||||
|
'entity_type' => 'node', |
||||||
|
'bundle' => ISLANDORA_COLLECTION_BUNDLE, |
||||||
|
'label' => $t("MODS"), |
||||||
|
'description' => $t("A MODS record for the Fedora resource"), |
||||||
|
'required' => FALSE, |
||||||
|
); |
||||||
|
field_create_instance($mods_field_instance); |
||||||
|
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_MODS_FIELD))); |
||||||
|
} |
||||||
|
|
||||||
|
// Add dcterms fields. |
||||||
|
islandora_dcterms_attach_fields_to_bundle(ISLANDORA_COLLECTION_BUNDLE); |
||||||
|
} |
@ -0,0 +1,78 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
/** |
||||||
|
* @file |
||||||
|
* Exported views code for this module. |
||||||
|
*/ |
||||||
|
|
||||||
|
/** |
||||||
|
* Implements hook_views_default_views(). |
||||||
|
*/ |
||||||
|
function islandora_collection_views_default_views() { |
||||||
|
$view = new view(); |
||||||
|
$view->name = 'collection_view'; |
||||||
|
$view->description = 'Shows all members of a collection'; |
||||||
|
$view->tag = 'default'; |
||||||
|
$view->base_table = 'node'; |
||||||
|
$view->human_name = 'Collection View'; |
||||||
|
$view->core = 7; |
||||||
|
$view->api_version = '3.0'; |
||||||
|
$view->disabled = FALSE; /* Edit this to true to make a default view disabled initially */ |
||||||
|
|
||||||
|
/* Display: Master */ |
||||||
|
$handler = $view->new_display('default', 'Master', 'default'); |
||||||
|
$handler->display->display_options['title'] = 'Members'; |
||||||
|
$handler->display->display_options['use_more_always'] = FALSE; |
||||||
|
$handler->display->display_options['access']['type'] = 'perm'; |
||||||
|
$handler->display->display_options['cache']['type'] = 'none'; |
||||||
|
$handler->display->display_options['query']['type'] = 'views_query'; |
||||||
|
$handler->display->display_options['exposed_form']['type'] = 'basic'; |
||||||
|
$handler->display->display_options['pager']['type'] = 'full'; |
||||||
|
$handler->display->display_options['pager']['options']['items_per_page'] = '10'; |
||||||
|
$handler->display->display_options['style_plugin'] = 'default'; |
||||||
|
$handler->display->display_options['row_plugin'] = 'node'; |
||||||
|
/* Relationship: Content: Relation: pcdm:hasMember (node → node) */ |
||||||
|
$handler->display->display_options['relationships']['relation_pcdm_hasmember_node']['id'] = 'relation_pcdm_hasmember_node'; |
||||||
|
$handler->display->display_options['relationships']['relation_pcdm_hasmember_node']['table'] = 'node'; |
||||||
|
$handler->display->display_options['relationships']['relation_pcdm_hasmember_node']['field'] = 'relation_pcdm_hasmember_node'; |
||||||
|
$handler->display->display_options['relationships']['relation_pcdm_hasmember_node']['required'] = TRUE; |
||||||
|
$handler->display->display_options['relationships']['relation_pcdm_hasmember_node']['r_index'] = '1'; |
||||||
|
$handler->display->display_options['relationships']['relation_pcdm_hasmember_node']['entity_deduplication_left'] = 0; |
||||||
|
$handler->display->display_options['relationships']['relation_pcdm_hasmember_node']['entity_deduplication_right'] = 0; |
||||||
|
/* Field: Content: Title */ |
||||||
|
$handler->display->display_options['fields']['title']['id'] = 'title'; |
||||||
|
$handler->display->display_options['fields']['title']['table'] = 'node'; |
||||||
|
$handler->display->display_options['fields']['title']['field'] = 'title'; |
||||||
|
$handler->display->display_options['fields']['title']['label'] = ''; |
||||||
|
$handler->display->display_options['fields']['title']['alter']['word_boundary'] = FALSE; |
||||||
|
$handler->display->display_options['fields']['title']['alter']['ellipsis'] = FALSE; |
||||||
|
/* Sort criterion: Content: Post date */ |
||||||
|
$handler->display->display_options['sorts']['created']['id'] = 'created'; |
||||||
|
$handler->display->display_options['sorts']['created']['table'] = 'node'; |
||||||
|
$handler->display->display_options['sorts']['created']['field'] = 'created'; |
||||||
|
$handler->display->display_options['sorts']['created']['order'] = 'DESC'; |
||||||
|
/* Contextual filter: Content: Node UUID */ |
||||||
|
$handler->display->display_options['arguments']['uuid']['id'] = 'uuid'; |
||||||
|
$handler->display->display_options['arguments']['uuid']['table'] = 'node'; |
||||||
|
$handler->display->display_options['arguments']['uuid']['field'] = 'uuid'; |
||||||
|
$handler->display->display_options['arguments']['uuid']['relationship'] = 'relation_pcdm_hasmember_node'; |
||||||
|
$handler->display->display_options['arguments']['uuid']['default_argument_type'] = 'fixed'; |
||||||
|
$handler->display->display_options['arguments']['uuid']['summary']['number_of_records'] = '0'; |
||||||
|
$handler->display->display_options['arguments']['uuid']['summary']['format'] = 'default_summary'; |
||||||
|
$handler->display->display_options['arguments']['uuid']['summary_options']['items_per_page'] = '25'; |
||||||
|
$handler->display->display_options['arguments']['uuid']['limit'] = '0'; |
||||||
|
/* Filter criterion: Content: Published */ |
||||||
|
$handler->display->display_options['filters']['status']['id'] = 'status'; |
||||||
|
$handler->display->display_options['filters']['status']['table'] = 'node'; |
||||||
|
$handler->display->display_options['filters']['status']['field'] = 'status'; |
||||||
|
$handler->display->display_options['filters']['status']['value'] = 1; |
||||||
|
$handler->display->display_options['filters']['status']['group'] = 1; |
||||||
|
$handler->display->display_options['filters']['status']['expose']['operator'] = FALSE; |
||||||
|
|
||||||
|
/* Display: Page */ |
||||||
|
$handler = $view->new_display('page', 'Page', 'page'); |
||||||
|
$handler->display->display_options['path'] = 'collection-view/%'; |
||||||
|
|
||||||
|
$views[$view->name] = $view; |
||||||
|
return $views; |
||||||
|
} |
@ -0,0 +1,35 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
/** |
||||||
|
* @file |
||||||
|
* Utility functions for defining collection relationships. |
||||||
|
*/ |
||||||
|
|
||||||
|
/** |
||||||
|
* Utility function to define relation types using CTools export code. |
||||||
|
*/ |
||||||
|
function islandora_collection_define_relations() { |
||||||
|
$relation_type = new stdClass(); |
||||||
|
$relation_type->disabled = FALSE; /* Edit this to true to make a default relation_type disabled initially */ |
||||||
|
$relation_type->api_version = 1; |
||||||
|
$relation_type->relation_type = 'pcdm_hasmember'; |
||||||
|
$relation_type->label = 'pcdm:hasMember'; |
||||||
|
$relation_type->reverse_label = 'fedora:hasParent'; |
||||||
|
$relation_type->directional = 1; |
||||||
|
$relation_type->transitive = 0; |
||||||
|
$relation_type->r_unique = 0; |
||||||
|
$relation_type->min_arity = 2; |
||||||
|
$relation_type->max_arity = 2; |
||||||
|
$relation_type->source_bundles = array( |
||||||
|
0 => 'node:basic_image', |
||||||
|
1 => 'node:collection', |
||||||
|
); |
||||||
|
$relation_type->target_bundles = array( |
||||||
|
0 => 'node:basic_image', |
||||||
|
1 => 'node:collection', |
||||||
|
); |
||||||
|
// Relation type create adds default keys. It also handles casting to array. |
||||||
|
$relation_type = relation_type_create($relation_type); |
||||||
|
// Yes, in relation.module 'save' is a distinct step from 'create'. |
||||||
|
relation_type_save($relation_type); |
||||||
|
} |
Loading…
Reference in new issue