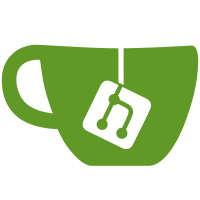
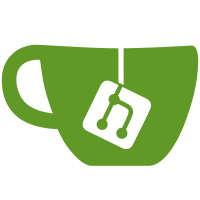
3 changed files with 240 additions and 0 deletions
@ -0,0 +1,136 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\islandora\Plugin\Field\FieldFormatter; |
||||
|
||||
use Drupal\Core\Entity\EntityStorageInterface; |
||||
use Drupal\Core\Field\FieldDefinitionInterface; |
||||
use Drupal\Core\Field\FieldItemListInterface; |
||||
use Drupal\Core\Session\AccountInterface; |
||||
use Drupal\image\Plugin\Field\FieldFormatter\ImageFormatter; |
||||
use Drupal\islandora\IslandoraUtils; |
||||
use Symfony\Component\DependencyInjection\ContainerInterface; |
||||
|
||||
/** |
||||
* Plugin implementation of the 'image' formatter for a media. |
||||
* |
||||
* @FieldFormatter( |
||||
* id = "islandora_image", |
||||
* label = @Translation("Islandora Image"), |
||||
* field_types = { |
||||
* "image" |
||||
* }, |
||||
* quickedit = { |
||||
* "editor" = "image" |
||||
* } |
||||
* ) |
||||
*/ |
||||
class IslandoraImageFormatter extends ImageFormatter { |
||||
|
||||
/** |
||||
* Islandora utility functions. |
||||
* |
||||
* @var \Drupal\islandora\IslandoraUtils |
||||
*/ |
||||
protected $utils; |
||||
|
||||
/** |
||||
* Constructs an IslandoraImageFormatter object. |
||||
* |
||||
* @param string $plugin_id |
||||
* The plugin_id for the formatter. |
||||
* @param mixed $plugin_definition |
||||
* The plugin implementation definition. |
||||
* @param \Drupal\Core\Field\FieldDefinitionInterface $field_definition |
||||
* The definition of the field to which the formatter is associated. |
||||
* @param array $settings |
||||
* The formatter settings. |
||||
* @param string $label |
||||
* The formatter label display setting. |
||||
* @param string $view_mode |
||||
* The view mode. |
||||
* @param array $third_party_settings |
||||
* Any third party settings settings. |
||||
* @param \Drupal\Core\Session\AccountInterface $current_user |
||||
* The current user. |
||||
* @param \Drupal\Core\Entity\EntityStorageInterface $image_style_storage |
||||
* The image style storage. |
||||
* @param \Drupal\islandora\IslandoraUtils $utils |
||||
* Islandora utils. |
||||
*/ |
||||
public function __construct( |
||||
$plugin_id, |
||||
$plugin_definition, |
||||
FieldDefinitionInterface $field_definition, |
||||
array $settings, |
||||
$label, |
||||
$view_mode, |
||||
array $third_party_settings, |
||||
AccountInterface $current_user, |
||||
EntityStorageInterface $image_style_storage, |
||||
IslandoraUtils $utils |
||||
) { |
||||
parent::__construct( |
||||
$plugin_id, |
||||
$plugin_definition, |
||||
$field_definition, |
||||
$settings, |
||||
$label, |
||||
$view_mode, |
||||
$third_party_settings, |
||||
$current_user, |
||||
$image_style_storage |
||||
); |
||||
$this->utils = $utils; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public static function create(ContainerInterface $container, array $configuration, $plugin_id, $plugin_definition) { |
||||
return new static( |
||||
$plugin_id, |
||||
$plugin_definition, |
||||
$configuration['field_definition'], |
||||
$configuration['settings'], |
||||
$configuration['label'], |
||||
$configuration['view_mode'], |
||||
$configuration['third_party_settings'], |
||||
$container->get('current_user'), |
||||
$container->get('entity.manager')->getStorage('image_style'), |
||||
$container->get('islandora.utils') |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function viewElements(FieldItemListInterface $items, $langcode) { |
||||
$elements = parent::viewElements($items, $langcode); |
||||
|
||||
$image_link_setting = $this->getSetting('image_link'); |
||||
// Check if the formatter involves a link. |
||||
if ($image_link_setting != 'content') { |
||||
return $elements; |
||||
} |
||||
|
||||
$entity = $items->getEntity(); |
||||
if ($entity->isNew() || $entity->getEntityTypeId() != 'media') { |
||||
return $elements; |
||||
} |
||||
|
||||
$node = $this->utils->getParentNode($entity); |
||||
|
||||
if ($node === NULL) { |
||||
return $elements; |
||||
} |
||||
|
||||
$url = $node->urlInfo(); |
||||
|
||||
foreach ($elements as &$element) { |
||||
$element['#url'] = $url; |
||||
} |
||||
|
||||
return $elements; |
||||
} |
||||
|
||||
} |
@ -0,0 +1,93 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\Tests\islandora\Functional; |
||||
|
||||
/** |
||||
* Tests the links for image fields with the islandora_image field formatter. |
||||
* |
||||
* @group islandora |
||||
* @coversDefaultClass \Drupal\islandora\Plugin\Field\FieldFormatter\IslandoraImageFormatter |
||||
*/ |
||||
class IslandoraImageFormatterTest extends IslandoraFunctionalTestBase { |
||||
|
||||
/** |
||||
* @covers \Drupal\islandora\Plugin\Field\FieldFormatter\IslandoraImageFormatter::viewElements |
||||
*/ |
||||
public function testIslandoraImageFormatter() { |
||||
|
||||
// Log in. |
||||
$account = $this->drupalCreateUser([ |
||||
'bypass node access', |
||||
'view media', |
||||
'create media', |
||||
]); |
||||
$this->drupalLogin($account); |
||||
|
||||
// Create an image media type. |
||||
$testImageMediaType = $this->createMediaType('image', ['id' => 'test_image_media_type']); |
||||
$testImageMediaType->save(); |
||||
$this->createEntityReferenceField('media', $testImageMediaType->id(), 'field_media_of', 'Media Of', 'node', 'default', [], 2); |
||||
|
||||
// Set the display mode to use the islandora_image formatter. |
||||
// Also, only show the image on display to remove clutter. |
||||
$display_options = [ |
||||
'type' => 'islandora_image', |
||||
'settings' => ['image_style' => NULL, 'image_link' => 'content'], |
||||
]; |
||||
$display = entity_get_display('media', $testImageMediaType->id(), 'default'); |
||||
$display->setComponent('field_media_image', $display_options) |
||||
->removeComponent('created') |
||||
->removeComponent('uid') |
||||
->removeComponent('thumbnail') |
||||
->save(); |
||||
|
||||
// Make a node. |
||||
$node = $this->container->get('entity_type.manager')->getStorage('node')->create([ |
||||
'type' => $this->testType->id(), |
||||
'title' => 'Test Node', |
||||
]); |
||||
$node->save(); |
||||
|
||||
// Make a image for the Media. |
||||
$file = $this->container->get('entity_type.manager')->getStorage('file')->create([ |
||||
'uid' => $account->id(), |
||||
'uri' => "public://test.jpeg", |
||||
'filename' => "test.jpeg", |
||||
'filemime' => "image/jpeg", |
||||
'status' => FILE_STATUS_PERMANENT, |
||||
]); |
||||
$file->save(); |
||||
|
||||
// Make the media, and associate it with the image and node. |
||||
$media = $this->container->get('entity_type.manager')->getStorage('media')->create([ |
||||
'bundle' => $testImageMediaType->id(), |
||||
'name' => 'Media', |
||||
'field_media_image' => |
||||
[ |
||||
'target_id' => $file->id(), |
||||
'alt' => 'Some Alt', |
||||
'title' => 'Some Title', |
||||
], |
||||
'field_media_of' => ['target_id' => $node->id()], |
||||
]); |
||||
$media->save(); |
||||
|
||||
// View the media. |
||||
$this->drupalGet("media/1"); |
||||
|
||||
// Assert that the image is rendered into html as a link pointing |
||||
// to the Node, not the Media (that's what the islandora_image |
||||
// formatter does). |
||||
$elements = $this->xpath( |
||||
'//a[@href=:path]/img[@src=:url and @alt=:alt and @title=:title]', |
||||
[ |
||||
':path' => $node->url(), |
||||
':url' => file_url_transform_relative(file_create_url($file->getFileUri())), |
||||
':alt' => 'Some Alt', |
||||
':title' => 'Some Title', |
||||
] |
||||
); |
||||
$this->assertEqual(count($elements), 1, 'Image linked to content formatter displaying points to Node and not Media.'); |
||||
} |
||||
|
||||
} |
Loading…
Reference in new issue