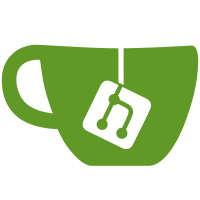
7 changed files with 179 additions and 2 deletions
@ -0,0 +1,52 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Drupal\twig_tweak; |
||||||
|
|
||||||
|
use Drupal\Core\Cache\CacheableDependencyInterface; |
||||||
|
use Drupal\Core\Cache\CacheableMetadata; |
||||||
|
use Drupal\Core\Render\Element; |
||||||
|
|
||||||
|
/** |
||||||
|
* Cache metadata extractor service. |
||||||
|
*/ |
||||||
|
class CacheMetadataExtractor { |
||||||
|
|
||||||
|
/** |
||||||
|
* Extracts cache metadata from object or render array. |
||||||
|
* |
||||||
|
* @param \Drupal\Core\Cache\CacheableDependencyInterface|array $input |
||||||
|
* The cacheable object or render array. |
||||||
|
* |
||||||
|
* @return array |
||||||
|
* A render array with extracted cache metadata. |
||||||
|
*/ |
||||||
|
public function extractCacheMetadata($input): array { |
||||||
|
if ($input instanceof CacheableDependencyInterface) { |
||||||
|
$cache_metadata = CacheableMetadata::createFromObject($input); |
||||||
|
} |
||||||
|
elseif (is_array($input)) { |
||||||
|
$cache_metadata = self::extractFromArray($input); |
||||||
|
} |
||||||
|
else { |
||||||
|
$message = sprintf('The input should be either instance of %s or array. %s was given.', CacheableDependencyInterface::class, \get_debug_type($input)); |
||||||
|
throw new \InvalidArgumentException($message); |
||||||
|
} |
||||||
|
|
||||||
|
$build = []; |
||||||
|
$cache_metadata->applyTo($build); |
||||||
|
return $build; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* Extracts cache metadata from renders array. |
||||||
|
*/ |
||||||
|
private function extractFromArray(array $build): CacheableMetadata { |
||||||
|
$cache_metadata = CacheableMetadata::createFromRenderArray($build); |
||||||
|
$keys = Element::children($build); |
||||||
|
foreach (array_intersect_key($build, array_flip($keys)) as $item) { |
||||||
|
$cache_metadata->addCacheableDependency(self::extractFromArray($item)); |
||||||
|
} |
||||||
|
return $cache_metadata; |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,91 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Drupal\Tests\twig_tweak\Kernel; |
||||||
|
|
||||||
|
use Drupal\Core\Cache\CacheableMetadata; |
||||||
|
|
||||||
|
/** |
||||||
|
* A test for Cache Metadata Extractor service. |
||||||
|
* |
||||||
|
* @group twig_tweak |
||||||
|
*/ |
||||||
|
final class CacheMetadataExtractorTest extends AbstractExtractorTestCase { |
||||||
|
|
||||||
|
/** |
||||||
|
* Test callback. |
||||||
|
*/ |
||||||
|
public function testCacheMetadataExtractor(): void { |
||||||
|
|
||||||
|
$extractor = $this->container->get('twig_tweak.cache_metadata_extractor'); |
||||||
|
|
||||||
|
// -- Object. |
||||||
|
$input = new CacheableMetadata(); |
||||||
|
$input->setCacheMaxAge(5); |
||||||
|
$input->setCacheContexts(['url', 'user.permissions']); |
||||||
|
$input->setCacheTags(['node', 'node.view']); |
||||||
|
|
||||||
|
$build = $extractor->extractCacheMetadata($input); |
||||||
|
$expected_build['#cache'] = [ |
||||||
|
'contexts' => ['url', 'user.permissions'], |
||||||
|
'tags' => ['node', 'node.view'], |
||||||
|
'max-age' => 5, |
||||||
|
]; |
||||||
|
self::assertSame($expected_build, $build); |
||||||
|
|
||||||
|
// -- Render array. |
||||||
|
$input = [ |
||||||
|
'foo' => [ |
||||||
|
'#cache' => [ |
||||||
|
'tags' => ['foo', 'foo.view'], |
||||||
|
], |
||||||
|
'bar' => [ |
||||||
|
0 => [ |
||||||
|
'#cache' => [ |
||||||
|
'tags' => ['bar-0'], |
||||||
|
], |
||||||
|
], |
||||||
|
1 => [ |
||||||
|
'#cache' => [ |
||||||
|
'tags' => ['bar-1'], |
||||||
|
], |
||||||
|
], |
||||||
|
'#cache' => [ |
||||||
|
'tags' => ['bar', 'bar.view'], |
||||||
|
'contexts' => ['url.path'], |
||||||
|
'max-age' => 10, |
||||||
|
], |
||||||
|
], |
||||||
|
], |
||||||
|
'#cache' => [ |
||||||
|
'contexts' => ['url', 'user.permissions'], |
||||||
|
'tags' => ['node', 'node.view'], |
||||||
|
'max-age' => 20, |
||||||
|
], |
||||||
|
]; |
||||||
|
$build = $extractor->extractCacheMetadata($input); |
||||||
|
|
||||||
|
$expected_build = [ |
||||||
|
'#cache' => [ |
||||||
|
'contexts' => ['url', 'url.path', 'user.permissions'], |
||||||
|
'tags' => [ |
||||||
|
'bar', |
||||||
|
'bar-0', |
||||||
|
'bar-1', |
||||||
|
'bar.view', |
||||||
|
'foo', |
||||||
|
'foo.view', |
||||||
|
'node', |
||||||
|
'node.view', |
||||||
|
], |
||||||
|
'max-age' => 10, |
||||||
|
], |
||||||
|
]; |
||||||
|
self::assertSame($expected_build, $build); |
||||||
|
|
||||||
|
// -- Wrong type. |
||||||
|
self::expectErrorMessage('The input should be either instance of Drupal\Core\Cache\CacheableDependencyInterface or array. stdClass was given.'); |
||||||
|
/** @noinspection PhpParamsInspection */ |
||||||
|
$extractor->extractCacheMetadata(new \stdClass()); |
||||||
|
} |
||||||
|
|
||||||
|
} |
Loading…
Reference in new issue