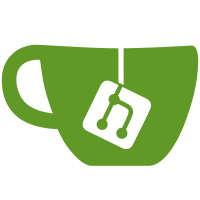
2 changed files with 0 additions and 125 deletions
@ -1,113 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace Drupal\reserve; |
|
||||||
|
|
||||||
use Drupal\Component\Utility\Unicode; |
|
||||||
use Drupal\Core\Entity\EntityInterface; |
|
||||||
use Drupal\Core\Form\FormStateInterface; |
|
||||||
use Drupal\field\Entity\FieldConfig; |
|
||||||
|
|
||||||
/** |
|
||||||
* Helper for reserve_form_alter(). |
|
||||||
*/ |
|
||||||
class BundleFormAlter { |
|
||||||
|
|
||||||
/** |
|
||||||
* Entity type definition. |
|
||||||
* |
|
||||||
* @var \Drupal\Core\Entity\ContentEntityTypeInterface |
|
||||||
*/ |
|
||||||
protected $definition; |
|
||||||
|
|
||||||
/** |
|
||||||
* The entity bundle. |
|
||||||
* |
|
||||||
* @var string |
|
||||||
*/ |
|
||||||
protected $bundle; |
|
||||||
|
|
||||||
/** |
|
||||||
* The bundle label. |
|
||||||
* |
|
||||||
* @var string |
|
||||||
*/ |
|
||||||
protected $bundleLabel; |
|
||||||
|
|
||||||
/** |
|
||||||
* The entity type ID. |
|
||||||
* |
|
||||||
* @var string |
|
||||||
*/ |
|
||||||
protected $entityTypeId; |
|
||||||
|
|
||||||
/** |
|
||||||
* The form entity which has been used for populating form element defaults. |
|
||||||
* |
|
||||||
* @var \Drupal\Core\Entity\EntityInterface |
|
||||||
*/ |
|
||||||
protected $entity; |
|
||||||
|
|
||||||
/** |
|
||||||
* Construct a BundleFormAlter object. |
|
||||||
* |
|
||||||
* @param \Drupal\Core\Entity\EntityInterface $entity |
|
||||||
* The entity object. |
|
||||||
*/ |
|
||||||
public function __construct(EntityInterface $entity) { |
|
||||||
$this->entity = $entity; |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* This is a helper for og_ui_form_alter(). |
|
||||||
* |
|
||||||
* @param array $form |
|
||||||
* The form variable. |
|
||||||
* @param \Drupal\Core\Form\FormStateInterface $form_state |
|
||||||
* The form state object. |
|
||||||
*/ |
|
||||||
public function formAlter(array &$form, FormStateInterface $form_state) { |
|
||||||
$this->prepare($form, $form_state); |
|
||||||
$this->addReserveCategories($form, $form_state); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* Prepares object properties and adds the og details element. |
|
||||||
* |
|
||||||
* @param array $form |
|
||||||
* The form variable. |
|
||||||
* @param \Drupal\Core\Form\FormStateInterface $form_state |
|
||||||
* The form state object. |
|
||||||
*/ |
|
||||||
protected function prepare(array &$form, FormStateInterface $form_state) { |
|
||||||
// Example: article. |
|
||||||
$this->bundle = $this->entity->id(); |
|
||||||
// Example: Article. |
|
||||||
$this->bundleLabel = Unicode::lcfirst($this->entity->label()); |
|
||||||
$this->definition = $this->entity->getEntityType(); |
|
||||||
// Example: node. |
|
||||||
$this->entityTypeId = $this->definition->getBundleOf(); |
|
||||||
|
|
||||||
$form['reserve'] = [ |
|
||||||
'#type' => 'details', |
|
||||||
'#title' => t('Reserve'), |
|
||||||
'#collapsible' => TRUE, |
|
||||||
'#group' => 'additional_settings', |
|
||||||
'#description' => t('If any Reserve Categories are selected here; this bundle will be reservable.'), |
|
||||||
]; |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* Adds the "is group?" checkbox. |
|
||||||
*/ |
|
||||||
protected function addReserveCategories(array &$form, FormStateInterface $form_state) { |
|
||||||
$options = array('big', 'small'); |
|
||||||
$form['reserve']['reserve_categories'] = [ |
|
||||||
'#type' => 'checkboxes', |
|
||||||
'#title' => t('Categories'), |
|
||||||
'#options' => array_combine($options, $options), |
|
||||||
//'#default_value' => Og::isGroup($this->entityTypeId, $this->bundle), |
|
||||||
|
|
||||||
]; |
|
||||||
} |
|
||||||
|
|
||||||
} |
|
Loading…
Reference in new issue