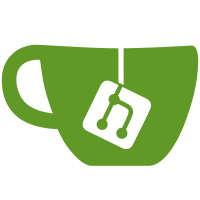
19 changed files with 222 additions and 321 deletions
@ -0,0 +1,107 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
/** |
||||||
|
* @file |
||||||
|
* Utility functions for working with DC fields. |
||||||
|
*/ |
||||||
|
|
||||||
|
/** |
||||||
|
* Adds all the DC fields to a bundle. |
||||||
|
* |
||||||
|
* @param string $bundle_name |
||||||
|
* The name of the bundle to give the fields. |
||||||
|
*/ |
||||||
|
function islandora_dc_add_fields_to_bundle($bundle_name) { |
||||||
|
|
||||||
|
// If this gets called from an install hook, we can't guarantee the t's |
||||||
|
// existance. |
||||||
|
$t = get_t(); |
||||||
|
|
||||||
|
// Big list of field names/labels/descriptions. |
||||||
|
$field_data = array( |
||||||
|
ISLANDORA_DC_CONTRIBUTOR_FIELD => array( |
||||||
|
'label' => 'DC Contributor', |
||||||
|
'description' => 'Dublin Core 1.1 Contributor Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_COVERAGE_FIELD => array( |
||||||
|
'label' => 'DC Coverage', |
||||||
|
'description' => 'Dublin Core 1.1 Coverage Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_CREATOR_FIELD => array( |
||||||
|
'label' => 'DC Creator', |
||||||
|
'description' => 'Dublin Core 1.1 Creator Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_DATE_FIELD => array( |
||||||
|
'label' => 'DC Date', |
||||||
|
'description' => 'Dublin Core 1.1 Date Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_DESCRIPTION_FIELD => array( |
||||||
|
'label' => 'DC Description', |
||||||
|
'description' => 'Dublin Core 1.1 Description Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_FORMAT_FIELD => array( |
||||||
|
'label' => 'DC Format', |
||||||
|
'description' => 'Dublin Core 1.1 Format Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_IDENTIFIER_FIELD => array( |
||||||
|
'label' => 'DC Identifier', |
||||||
|
'description' => 'Dublin Core 1.1 Identifier Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_LANGUAGE_FIELD => array( |
||||||
|
'label' => 'DC Language', |
||||||
|
'description' => 'Dublin Core 1.1 Language Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_PUBLISHER_FIELD => array( |
||||||
|
'label' => 'DC Publisher', |
||||||
|
'description' => 'Dublin Core 1.1 Publisher Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_RELATION_FIELD => array( |
||||||
|
'label' => 'DC Relation', |
||||||
|
'description' => 'Dublin Core 1.1 Relation Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_RIGHTS_FIELD => array( |
||||||
|
'label' => 'DC Rights', |
||||||
|
'description' => 'Dublin Core 1.1 Rights Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_SOURCE_FIELD => array( |
||||||
|
'label' => 'DC Source', |
||||||
|
'description' => 'Dublin Core 1.1 Source Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_SUBJECT_FIELD => array( |
||||||
|
'label' => 'DC Subject', |
||||||
|
'description' => 'Dublin Core 1.1 Subject Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_TITLE_FIELD => array( |
||||||
|
'label' => 'DC Title', |
||||||
|
'description' => 'Dublin Core 1.1 Title Metadata', |
||||||
|
), |
||||||
|
ISLANDORA_DC_TYPE_FIELD => array( |
||||||
|
'label' => 'DC Type', |
||||||
|
'description' => 'Dublin Core 1.1 Type Metadata', |
||||||
|
), |
||||||
|
); |
||||||
|
|
||||||
|
// Iterate over fields and add each to the specified bundle. |
||||||
|
foreach ($field_data as $field_name => $data) { |
||||||
|
$field_label = $data['label']; |
||||||
|
$field_description = $data['description']; |
||||||
|
|
||||||
|
if (field_info_field($field_name)) { |
||||||
|
$field_instance = array( |
||||||
|
'field_name' => $field_name, |
||||||
|
'entity_type' => 'node', |
||||||
|
'bundle' => $bundle_name, |
||||||
|
'label' => $t("%label", array("%description" => $field_label)), |
||||||
|
'description' => $t("%description", array("%description" => $field_description)), |
||||||
|
'required' => FALSE, |
||||||
|
); |
||||||
|
field_create_instance($field_instance); |
||||||
|
$message = $t('Field %name was added to %bundle successfully', |
||||||
|
array( |
||||||
|
'%name' => $field_name, |
||||||
|
'%bundle' => $bundle, |
||||||
|
)); |
||||||
|
drupal_set_message($message); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,5 @@ |
|||||||
|
name = Islandora DC |
||||||
|
description = "Fields and utilities for managing DC 1.1 metadata in Islandora" |
||||||
|
package = Islandora |
||||||
|
version = 7.x-dev |
||||||
|
core = 7.x |
@ -0,0 +1,40 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
/** |
||||||
|
* @file |
||||||
|
* Install hooks for this module. |
||||||
|
*/ |
||||||
|
|
||||||
|
/** |
||||||
|
* Implements hook_install(). |
||||||
|
* |
||||||
|
* Creates shared fields for content types using DC metadata. |
||||||
|
*/ |
||||||
|
function islandora_dc_install() { |
||||||
|
$field_names = array( |
||||||
|
ISLANDORA_DC_CONTRIBUTOR_FIELD, |
||||||
|
ISLANDORA_DC_COVERAGE_FIELD, |
||||||
|
ISLANDORA_DC_CREATOR_FIELD, |
||||||
|
ISLANDORA_DC_DATE_FIELD, |
||||||
|
ISLANDORA_DC_DESCRIPTION_FIELD, |
||||||
|
ISLANDORA_DC_FORMAT_FIELD, |
||||||
|
ISLANDORA_DC_IDENTIFIER_FIELD, |
||||||
|
ISLANDORA_DC_LANGUAGE_FIELD, |
||||||
|
ISLANDORA_DC_PUBLISHER_FIELD, |
||||||
|
ISLANDORA_DC_RELATION_FIELD, |
||||||
|
ISLANDORA_DC_RIGHTS_FIELD, |
||||||
|
ISLANDORA_DC_SOURCE_FIELD, |
||||||
|
ISLANDORA_DC_SUBJECT_FIELD, |
||||||
|
ISLANDORA_DC_TITLE_FIELD, |
||||||
|
ISLANDORA_DC_TYPE_FIELD, |
||||||
|
); |
||||||
|
|
||||||
|
foreach ($field_names as $field_name) { |
||||||
|
$field = array( |
||||||
|
'field_name' => $field_name, |
||||||
|
'type' => 'text_long', |
||||||
|
'cardinality' => FIELD_CARDINALITY_UNLIMITED, |
||||||
|
); |
||||||
|
field_create_field($field); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,24 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
/** |
||||||
|
* @file |
||||||
|
* Islandora module for working with DC Metadata. |
||||||
|
*/ |
||||||
|
|
||||||
|
define('ISLANDORA_DC_NAMESPACE', 'http://purl.org/dc/elements/1.1/'); |
||||||
|
define('ISLANDORA_DC_NAMESPACE_PREFIX', 'dc11'); |
||||||
|
define('ISLANDORA_DC_CONTRIBUTOR_FIELD', 'field_dc_contributor'); |
||||||
|
define('ISLANDORA_DC_COVERAGE_FIELD', 'field_dc_coverage'); |
||||||
|
define('ISLANDORA_DC_CREATOR_FIELD', 'field_dc_creator'); |
||||||
|
define('ISLANDORA_DC_DATE_FIELD', 'field_dc_date'); |
||||||
|
define('ISLANDORA_DC_DESCRIPTION_FIELD', 'field_dc_description'); |
||||||
|
define('ISLANDORA_DC_FORMAT_FIELD', 'field_dc_format'); |
||||||
|
define('ISLANDORA_DC_IDENTIFIER_FIELD', 'field_dc_identifier'); |
||||||
|
define('ISLANDORA_DC_LANGUAGE_FIELD', 'field_dc_language'); |
||||||
|
define('ISLANDORA_DC_PUBLISHER_FIELD', 'field_dc_publisher'); |
||||||
|
define('ISLANDORA_DC_RELATION_FIELD', 'field_dc_relation'); |
||||||
|
define('ISLANDORA_DC_RIGHTS_FIELD', 'field_dc_rights'); |
||||||
|
define('ISLANDORA_DC_SOURCE_FIELD', 'field_dc_source'); |
||||||
|
define('ISLANDORA_DC_SUBJECT_FIELD', 'field_dc_subject'); |
||||||
|
define('ISLANDORA_DC_TITLE_FIELD', 'field_dc_title'); |
||||||
|
define('ISLANDORA_DC_TYPE_FIELD', 'field_dc_type'); |
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
@ -1,212 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
/** |
|
||||||
* @file |
|
||||||
* Utility functions for working with dcterms fields. |
|
||||||
*/ |
|
||||||
|
|
||||||
/** |
|
||||||
* Attaches all the dcterms fields to a bundle. |
|
||||||
* |
|
||||||
* @param string $bundle_name |
|
||||||
* The name of the bundle to give the fields. |
|
||||||
*/ |
|
||||||
function islandora_dcterms_attach_fields_to_bundle($bundle_name) { |
|
||||||
|
|
||||||
$t = get_t(); |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_CONTRIBUTOR_FIELD)) { |
|
||||||
$dcterms_contributor_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_CONTRIBUTOR_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Contributor"), |
|
||||||
'description' => $t("Dublin Core Contributor Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_contributor_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_CONTRIBUTOR_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_COVERAGE_FIELD)) { |
|
||||||
$dcterms_coverage_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_COVERAGE_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Coverage"), |
|
||||||
'description' => $t("Dublin Core Coverage Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_coverage_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_COVERAGE_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_CREATOR_FIELD)) { |
|
||||||
$dcterms_creator_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_CREATOR_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Creator"), |
|
||||||
'description' => $t("Dublin Core Creator Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_creator_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_CREATOR_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_DATE_FIELD)) { |
|
||||||
$dcterms_date_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_DATE_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Date"), |
|
||||||
'description' => $t("Dublin Core Date Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_date_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_DATE_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_DESCRIPTION_FIELD)) { |
|
||||||
$dcterms_description_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_DESCRIPTION_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Description"), |
|
||||||
'description' => $t("Dublin Core Description Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_description_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_DESCRIPTION_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_FORMAT_FIELD)) { |
|
||||||
$dcterms_format_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_FORMAT_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Format"), |
|
||||||
'description' => $t("Dublin Core Format Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_format_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_FORMAT_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_IDENTIFIER_FIELD)) { |
|
||||||
$dcterms_identifier_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_IDENTIFIER_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Identfier"), |
|
||||||
'description' => $t("Dublin Core Identifier Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_identifier_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_IDENTIFIER_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_LANGUAGE_FIELD)) { |
|
||||||
$dcterms_language_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_LANGUAGE_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Language"), |
|
||||||
'description' => $t("Dublin Core Language Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_language_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_LANGUAGE_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_PUBLISHER_FIELD)) { |
|
||||||
$dcterms_publisher_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_PUBLISHER_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Publisher"), |
|
||||||
'description' => $t("Dublin Core Publisher Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_publisher_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_PUBLISHER_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_RELATION_FIELD)) { |
|
||||||
$dcterms_relation_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_RELATION_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Relation"), |
|
||||||
'description' => $t("Dublin Core Relation Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_relation_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_RELATION_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_RIGHTS_FIELD)) { |
|
||||||
$dcterms_rights_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_RIGHTS_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Rights"), |
|
||||||
'description' => $t("Dublin Core Rights Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_rights_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_RIGHTS_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_SOURCE_FIELD)) { |
|
||||||
$dcterms_source_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_SOURCE_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Source"), |
|
||||||
'description' => $t("Dublin Core Source Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_source_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_SOURCE_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_SUBJECT_FIELD)) { |
|
||||||
$dcterms_subject_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_SUBJECT_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Subject"), |
|
||||||
'description' => $t("Dublin Core Subject Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_subject_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_SUBJECT_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_TITLE_FIELD)) { |
|
||||||
$dcterms_title_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_TITLE_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Title"), |
|
||||||
'description' => $t("Dublin Core Title Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_title_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_TITLE_FIELD))); |
|
||||||
} |
|
||||||
|
|
||||||
if (field_info_field(ISLANDORA_DCTERMS_TYPE_FIELD)) { |
|
||||||
$dcterms_type_field_instance = array( |
|
||||||
'field_name' => ISLANDORA_DCTERMS_TYPE_FIELD, |
|
||||||
'entity_type' => 'node', |
|
||||||
'bundle' => $bundle_name, |
|
||||||
'label' => $t("DC Terms Type"), |
|
||||||
'description' => $t("Dublin Core Type Metadata"), |
|
||||||
'required' => FALSE, |
|
||||||
); |
|
||||||
field_create_instance($dcterms_type_field_instance); |
|
||||||
drupal_set_message($t('Field %name was created successfully', array('%name' => ISLANDORA_DCTERMS_TYPE_FIELD))); |
|
||||||
} |
|
||||||
} |
|
@ -1,5 +0,0 @@ |
|||||||
name = Islandora DC Terms |
|
||||||
description = "Fields and utilities for managing dcterms metadata in Islandora" |
|
||||||
package = Islandora |
|
||||||
version = 7.x-dev |
|
||||||
core = 7.x |
|
@ -1,40 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
/** |
|
||||||
* @file |
|
||||||
* Install hook for dcterms metadata. |
|
||||||
*/ |
|
||||||
|
|
||||||
/** |
|
||||||
* Implements hook_install(). |
|
||||||
* |
|
||||||
* Creates shared fields for all content types using dcterms metadata. |
|
||||||
*/ |
|
||||||
function islandora_dcterms_install() { |
|
||||||
$dcterms_field_names = array( |
|
||||||
ISLANDORA_DCTERMS_CONTRIBUTOR_FIELD, |
|
||||||
ISLANDORA_DCTERMS_COVERAGE_FIELD, |
|
||||||
ISLANDORA_DCTERMS_CREATOR_FIELD, |
|
||||||
ISLANDORA_DCTERMS_DATE_FIELD, |
|
||||||
ISLANDORA_DCTERMS_DESCRIPTION_FIELD, |
|
||||||
ISLANDORA_DCTERMS_FORMAT_FIELD, |
|
||||||
ISLANDORA_DCTERMS_IDENTIFIER_FIELD, |
|
||||||
ISLANDORA_DCTERMS_LANGUAGE_FIELD, |
|
||||||
ISLANDORA_DCTERMS_PUBLISHER_FIELD, |
|
||||||
ISLANDORA_DCTERMS_RELATION_FIELD, |
|
||||||
ISLANDORA_DCTERMS_RIGHTS_FIELD, |
|
||||||
ISLANDORA_DCTERMS_SOURCE_FIELD, |
|
||||||
ISLANDORA_DCTERMS_SUBJECT_FIELD, |
|
||||||
ISLANDORA_DCTERMS_TITLE_FIELD, |
|
||||||
ISLANDORA_DCTERMS_TYPE_FIELD, |
|
||||||
); |
|
||||||
|
|
||||||
foreach ($dcterms_field_names as $field_name) { |
|
||||||
$field = array( |
|
||||||
'field_name' => $field_name, |
|
||||||
'type' => 'text_long', |
|
||||||
'cardinality' => FIELD_CARDINALITY_UNLIMITED, |
|
||||||
); |
|
||||||
field_create_field($field); |
|
||||||
} |
|
||||||
} |
|
@ -1,22 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
/** |
|
||||||
* @file |
|
||||||
* Collection of fields and utility functions for working with DC Metadata. |
|
||||||
*/ |
|
||||||
|
|
||||||
define('ISLANDORA_DCTERMS_CONTRIBUTOR_FIELD', 'field_dcterms_contributor'); |
|
||||||
define('ISLANDORA_DCTERMS_COVERAGE_FIELD', 'field_dcterms_coverage'); |
|
||||||
define('ISLANDORA_DCTERMS_CREATOR_FIELD', 'field_dcterms_creator'); |
|
||||||
define('ISLANDORA_DCTERMS_DATE_FIELD', 'field_dcterms_date'); |
|
||||||
define('ISLANDORA_DCTERMS_DESCRIPTION_FIELD', 'field_dcterms_description'); |
|
||||||
define('ISLANDORA_DCTERMS_FORMAT_FIELD', 'field_dcterms_format'); |
|
||||||
define('ISLANDORA_DCTERMS_IDENTIFIER_FIELD', 'field_dcterms_identifier'); |
|
||||||
define('ISLANDORA_DCTERMS_LANGUAGE_FIELD', 'field_dcterms_language'); |
|
||||||
define('ISLANDORA_DCTERMS_PUBLISHER_FIELD', 'field_dcterms_publisher'); |
|
||||||
define('ISLANDORA_DCTERMS_RELATION_FIELD', 'field_dcterms_relation'); |
|
||||||
define('ISLANDORA_DCTERMS_RIGHTS_FIELD', 'field_dcterms_rights'); |
|
||||||
define('ISLANDORA_DCTERMS_SOURCE_FIELD', 'field_dcterms_source'); |
|
||||||
define('ISLANDORA_DCTERMS_SUBJECT_FIELD', 'field_dcterms_subject'); |
|
||||||
define('ISLANDORA_DCTERMS_TITLE_FIELD', 'field_dcterms_title'); |
|
||||||
define('ISLANDORA_DCTERMS_TYPE_FIELD', 'field_dcterms_type'); |
|
Loading…
Reference in new issue