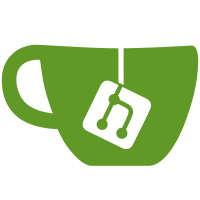
6 changed files with 205 additions and 0 deletions
@ -0,0 +1,50 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Provides the callback implementations for the service. |
||||
*/ |
||||
|
||||
/** |
||||
* The POST callback for the MEDIUM_SIZE service. |
||||
* |
||||
* @param array $args |
||||
* JSON encoded arguments. |
||||
* |
||||
* @return string |
||||
* Response message. |
||||
*/ |
||||
function islandora_medium_size_service_create($args) { |
||||
$uuid = $args['uuid']; |
||||
$tn_b64 = $args['file']; |
||||
$mimetype = $args['mimetype']; |
||||
$exploded = explode("/", $mimetype); |
||||
$extension = $exploded[1]; |
||||
|
||||
$entities = entity_uuid_load('node', array($uuid)); |
||||
|
||||
if (empty($entities)) { |
||||
return "Could not add medium sized image because there is no entity identified by $uuid"; |
||||
} |
||||
|
||||
$node = array_pop($entities); |
||||
|
||||
$file = file_save_data(base64_decode($tn_b64), "public://" . $uuid . "_MEDIUM_SIZE." . $extension, FILE_EXISTS_REPLACE); |
||||
|
||||
$node->field_medium_size[LANGUAGE_NONE][] = array( |
||||
'fid' => $file->fid, |
||||
'alt' => "Medium sized image for node $uuid", |
||||
'title' => "Medium sized image for node $uuid", |
||||
); |
||||
$node->field_tn[LANGUAGE_NONE][] = array( |
||||
'fid' => $file->fid, |
||||
'width' => 100, |
||||
'height' => 100, |
||||
'alt' => "Thumbnail for node $uuid", |
||||
'title' => "Thumbnail for node $uuid", |
||||
); |
||||
|
||||
node_save($node); |
||||
|
||||
return "Successfully added medium sized image to node $uuid"; |
||||
} |
@ -0,0 +1,6 @@
|
||||
name = Islandora Medium Size Service |
||||
description = "Adds medium size images to nodes" |
||||
package = Islandora |
||||
version = 7.x-dev |
||||
core = 7.x |
||||
dependencies[] = rest_server |
@ -0,0 +1,48 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Provides a service for adding medium size images to nodes. |
||||
*/ |
||||
|
||||
/** |
||||
* Implements hook_permission(). |
||||
*/ |
||||
function islandora_medium_size_service_permission() { |
||||
return array( |
||||
'islandora medium size service create' => array( |
||||
'title' => t('Add Medium Size'), |
||||
'description' => t('Allows external sources to add medium size images to nodes.'), |
||||
), |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Implements hook_services_resources(). |
||||
*/ |
||||
function islandora_medium_size_service_services_resources() { |
||||
return array( |
||||
'medium_size' => array( |
||||
'create' => array( |
||||
'help' => t('Adds a medium sized image to the node identified by UUID.'), |
||||
'file' => array( |
||||
'type' => 'inc', |
||||
'module' => 'islandora_medium_size_service', |
||||
'name' => 'include/islandora_medium_size_service', |
||||
), |
||||
'callback' => 'islandora_medium_size_service_create', |
||||
'access callback' => 'user_access', |
||||
'access arguments' => array('islandora medium size service create'), |
||||
'args' => array( |
||||
array( |
||||
'name' => 'args', |
||||
'type' => 'array', |
||||
'description' => t("JSON data containing arguments to add the medium sized image."), |
||||
'source' => 'data', |
||||
'optional' => FALSE, |
||||
), |
||||
), |
||||
), |
||||
), |
||||
); |
||||
} |
@ -0,0 +1,47 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Provides the callback implementations for the service. |
||||
*/ |
||||
|
||||
/** |
||||
* The POST callback for the TN service. |
||||
* |
||||
* @param string $type |
||||
* Entity type to which the RDF mapping belongs. |
||||
* @param string $bundle |
||||
* Bundle to which the RDF mapping belongs. |
||||
* |
||||
* @return array |
||||
* The RDF mapping. |
||||
*/ |
||||
function islandora_tn_service_create($args) { |
||||
$uuid = $args['uuid']; |
||||
$tn_b64 = $args['file']; |
||||
$mimetype = $args['mimetype']; |
||||
$exploded = explode("/", $mimetype); |
||||
$extension = $exploded[1]; |
||||
|
||||
$entities = entity_uuid_load('node', array($uuid)); |
||||
|
||||
if (empty($entities)) { |
||||
return "Could not add thumbnail because there is no entity identified by $uuid"; |
||||
} |
||||
|
||||
$node = array_pop($entities); |
||||
|
||||
$file = file_save_data(base64_decode($tn_b64), "public://" . $uuid . "_TN." . $extension, FILE_EXISTS_REPLACE); |
||||
|
||||
$node->field_tn[LANGUAGE_NONE][] = array( |
||||
'fid' => $file->fid, |
||||
'width' => 100, |
||||
'height' => 100, |
||||
'alt' => "Thumbnail for node $uuid", |
||||
'title' => "Thumbnail for node $uuid", |
||||
); |
||||
|
||||
node_save($node); |
||||
|
||||
return "Successfully added thumbnail to node $uuid"; |
||||
} |
@ -0,0 +1,6 @@
|
||||
name = Islandora TN Service |
||||
description = "Adds thumbnail images to nodes" |
||||
package = Islandora |
||||
version = 7.x-dev |
||||
core = 7.x |
||||
dependencies[] = rest_server |
@ -0,0 +1,48 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Provides a service for adding thumbnails to Drupal. |
||||
*/ |
||||
|
||||
/** |
||||
* Implements hook_permission(). |
||||
*/ |
||||
function islandora_tn_service_permission() { |
||||
return array( |
||||
'islandora tn service create' => array( |
||||
'title' => t('Add Thumbnails'), |
||||
'description' => t('Allows external sources to add thumbnails to nodes.'), |
||||
), |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* Implements hook_services_resources(). |
||||
*/ |
||||
function islandora_tn_service_services_resources() { |
||||
return array( |
||||
'tn' => array( |
||||
'create' => array( |
||||
'help' => t('Adds a thumbnail to the node identified by UUID.'), |
||||
'file' => array( |
||||
'type' => 'inc', |
||||
'module' => 'islandora_tn_service', |
||||
'name' => 'include/islandora_tn_service', |
||||
), |
||||
'callback' => 'islandora_tn_service_create', |
||||
'access callback' => 'user_access', |
||||
'access arguments' => array('islandora tn service create'), |
||||
'args' => array( |
||||
array( |
||||
'name' => 'args', |
||||
'type' => 'array', |
||||
'description' => t("JSON data containing arguments to add the thumbnail."), |
||||
'source' => 'data', |
||||
'optional' => FALSE, |
||||
), |
||||
), |
||||
), |
||||
), |
||||
); |
||||
} |
Loading…
Reference in new issue