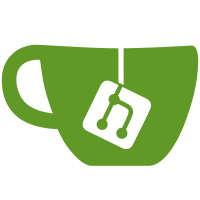
16 changed files with 158 additions and 811 deletions
@ -1,264 +0,0 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* batch creation form submit |
||||
* @global type $user |
||||
* @param array $form |
||||
* @param array $form_state |
||||
* @param array $content_models |
||||
*/ |
||||
function batch_creation_form(&$form_state, $collection_pid, $content_models) { |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_utils'); |
||||
module_load_include('inc', 'fedora_repository', 'CollectionPolicy'); |
||||
$cm_options = array(); |
||||
$name_mappings = array(); |
||||
foreach ($content_models as $content_model) { |
||||
if ($content_model->pid != "islandora:collectionCModel") { |
||||
$cm_options[$content_model->pid] = $content_model->name; |
||||
$name_mappings[] = $content_model->pid . '^' . $content_model->pid_namespace; |
||||
} |
||||
} |
||||
$mappings = implode('~~~', $name_mappings); |
||||
$form['#attributes']['enctype'] = 'multipart/form-data'; |
||||
|
||||
$form['titlebox'] = array( |
||||
'#type' => 'item', |
||||
'#value' => t("Batch ingest into $collection_pid"), |
||||
); |
||||
|
||||
$form['collection_pid'] = array( |
||||
'#type' => 'hidden', |
||||
'#value' => $collection_pid, |
||||
); |
||||
$form['namespace_mappings'] = array( |
||||
'#type' => 'hidden', |
||||
'#value' => $mappings, |
||||
); |
||||
$form['content_model'] = array( |
||||
'#title' => "Choose content model to be associated with objects ingested", |
||||
'#type' => 'select', |
||||
'#options' => $cm_options, |
||||
'#required' => TRUE, |
||||
'#description' => t("Content models describe the behaviours of objects with which they are associated."), |
||||
); |
||||
$form['indicator']['file-location'] = array( |
||||
'#type' => 'file', |
||||
'#title' => t('Upload zipped folder'), |
||||
'#size' => 48, |
||||
'#description' => t('The zipped folder should contain all files necessary to build objects.<br .> |
||||
Related files must have the same filename, but with differing extensions to indicate mimetypes.<br /> |
||||
ie. <em>myFile.xml</em> and <em>myFile.jpg</em>'), |
||||
); |
||||
|
||||
$form['submit'] = array( |
||||
'#type' => 'submit', |
||||
'#value' => t('Create Objects ') |
||||
); |
||||
|
||||
|
||||
return($form); |
||||
} |
||||
|
||||
/** |
||||
* @param array $form |
||||
* @param array $form_state |
||||
*/ |
||||
function batch_creation_form_validate($form, &$form_state) { |
||||
|
||||
$field_name = 'file-location'; |
||||
if (isset($_FILES['files']) && is_uploaded_file($_FILES['files']['tmp_name'][$field_name])) { |
||||
$file = file_save_upload($field_name); |
||||
if ($file->filemime != 'application/zip') { |
||||
form_set_error($field_name, 'Input file must be a .zip file'); |
||||
return; |
||||
} |
||||
if (!$file) { |
||||
form_set_error($field_name, 'Error uploading file.'); |
||||
return; |
||||
} |
||||
$form_state['values']['file'] = $file; |
||||
} |
||||
else { |
||||
// set error |
||||
form_set_error($field_name, 'Error uploading file.'); |
||||
return; |
||||
} |
||||
} |
||||
|
||||
function batch_creation_form_submit($form, &$form_state) { |
||||
module_load_include('inc', 'fedora_repository', 'ContentModel'); |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_item'); |
||||
global $user; |
||||
$namespace_mappings = array(); |
||||
$content_model = $form_state['values']['content_model']; |
||||
$metadata = $form_state['values']['metadata_type']; |
||||
$collection_pid = $form_state['values']['collection_pid']; |
||||
$namespace_process = explode('~~~', $form_state['values']['namespace_mappings']); |
||||
foreach ($namespace_process as $line) { |
||||
$line_parts = explode('^', $line); |
||||
$namespace_mappings[$line_parts[0]] = $line_parts[1]; |
||||
} |
||||
$namespace = $namespace_mappings[$content_model]; |
||||
$namespace = preg_replace('/:.*/', '', $namespace); |
||||
$dir_name = "temp" . $user->uid; |
||||
$tmp_dir = file_directory_path() . '/' . $dir_name . '/'; |
||||
mkdir($tmp_dir); |
||||
$file = $form_state['values']['file']; |
||||
$fileName = $file->filepath; |
||||
$file_list = array(); |
||||
$cmdString = "unzip -q -o -d $tmp_dir \"$fileName\""; |
||||
system($cmdString, $retVal); |
||||
$dirs = array(); |
||||
$do_not_add = array('.', '..', '__MACOSX'); |
||||
array_push($dirs, $tmp_dir); |
||||
$files = scandir($tmp_dir); |
||||
foreach ($files as $file) { |
||||
if (is_dir("$tmp_dir/$file") & !in_array($file, $do_not_add)) { |
||||
array_push($dirs, $tmp_dir . $file); |
||||
} |
||||
} |
||||
foreach ($dirs as $directory) { |
||||
if ($inputs = opendir($directory)) { |
||||
while (FALSE !== ($file_name = readdir($inputs))) { |
||||
if (!in_array($file_name, $do_not_add) && is_dir($file_name) == FALSE) { |
||||
$ext = strrchr($file_name, '.'); |
||||
$base = preg_replace("/$ext$/", '', $file_name); |
||||
$ext = substr($ext, 1); |
||||
if ($ext) { |
||||
if ($directory[strlen($directory)-1] != '/'){ |
||||
$directory .= '/'; |
||||
} |
||||
$file_list[$base][$ext] = "$directory" . $file_name; |
||||
} |
||||
} |
||||
} |
||||
closedir($inputs); |
||||
} |
||||
} |
||||
|
||||
if (($cm = ContentModel::loadFromModel($content_model, 'ISLANDORACM')) === FALSE) { |
||||
drupal_set_message("$content_model not found", "error"); |
||||
return; |
||||
} |
||||
|
||||
$batch = array( |
||||
'title' => 'Ingesting Objects', |
||||
'operations' => array(), |
||||
'file' => drupal_get_path('module', 'fedora_repository') . '/BatchIngest.inc', |
||||
); |
||||
|
||||
|
||||
foreach ($file_list as $label => $object_files) { |
||||
$batch['operations'][] = array('create_batch_objects', array($label, $content_model, $object_files, $collection_pid, $namespace, $metadata)); |
||||
} |
||||
$batch['operations'][] = array('recursive_directory_delete', array($tmp_dir)); |
||||
batch_set($batch); |
||||
batch_process(); |
||||
} |
||||
|
||||
/** |
||||
* |
||||
* @param <string> $label |
||||
* @param <string> $content_model |
||||
* @param <array> $object_files |
||||
* @param <string> $collection_pid |
||||
* @param <string> $namespace |
||||
* @param <string> $metadata |
||||
*/ |
||||
function create_batch_objects($label, $content_model, $object_files, $collection_pid, $namespace, $metadata) { |
||||
module_load_include('inc', 'fedora_repository', 'ContentModel'); |
||||
module_load_include('inc', 'fedora_repository', 'MimeClass'); |
||||
module_load_include('inc', 'fedora_reppository', 'api/fedora_item'); |
||||
|
||||
$cm = ContentModel::loadFromModel($content_model, 'ISLANDORACM'); |
||||
$allowed_mime_types = $cm->getMimetypes(); |
||||
$mime_helper = new MimeClass(); |
||||
$pid = fedora_item::get_next_PID_in_namespace($namespace); |
||||
|
||||
$item = Fedora_item::ingest_new_item($pid, 'A', $label, $owner); |
||||
$item->add_relationship('hasModel', $content_model, FEDORA_MODEL_URI); |
||||
$item->add_relationship('isMemberOfCollection', $collection_pid); |
||||
if ($object_files['xml']) { |
||||
$data = file_get_contents($object_files['xml']); |
||||
$xml = simplexml_load_string($data); |
||||
$identifier = $xml->getName(); |
||||
if ($identifier == 'dc') { |
||||
$item->modify_datastream_by_value($data, 'DC', "Dublin Core", 'text/xml'); |
||||
} |
||||
if ($identifier == 'mods') { |
||||
$item->add_datastream_from_string($mods_xml, 'MODS'); |
||||
$dc_xml = batch_create_dc_from_mods($mods_xml); |
||||
$item->modify_datastream_by_value($dc_xml, 'DC', "Dublin Core", 'text/xml'); |
||||
} |
||||
} |
||||
|
||||
unset($object_files['xml']); |
||||
foreach ($object_files as $ext => $filename) { |
||||
$file_mimetype = $mime_helper->get_mimetype($filename); |
||||
if (in_array($file_mimetype, $allowed_mime_types)) { |
||||
$added = $cm->execIngestRules($filename, $file_mimetype); |
||||
} |
||||
else { |
||||
$item->purge("$pid $label not ingested. $file_mimetype not permitted in objects associated with $content_model"); |
||||
continue; |
||||
} |
||||
$item->add_datastream_from_file($filename, "Source_File"); |
||||
|
||||
if (!empty($_SESSION['fedora_ingest_files'])) { |
||||
foreach ($_SESSION['fedora_ingest_files'] as $dsid => $datastream_file) { |
||||
$item->add_datastream_from_file($datastream_file, $dsid); |
||||
} |
||||
} |
||||
} |
||||
} |
||||
|
||||
/** |
||||
* transforms mods to dc |
||||
* @param <string> $mods_xml |
||||
* @return <string> |
||||
*/ |
||||
function batch_create_dc_from_mods($mods_xml) { |
||||
$path = drupal_get_path('module', 'fedora_repository'); |
||||
module_load_include('inc', 'fedora_repository', 'ObjectHelper'); |
||||
module_load_include('inc', 'fedora_repository', 'CollectionClass'); |
||||
|
||||
if ($xmlstr == NULL || strlen($xmlstr) < 5) { |
||||
return " "; |
||||
} |
||||
|
||||
try { |
||||
$proc = new XsltProcessor(); |
||||
} catch (Exception $e) { |
||||
drupal_set_message(t('@e', array('@e' => check_plain($e->getMessage()))), 'error'); |
||||
return " "; |
||||
} |
||||
|
||||
$xsl = new DomDocument(); |
||||
$xsl->load($path . '/xsl/mods_to_dc.xsl'); |
||||
$input = new DomDocument(); |
||||
$input->loadXML(trim($xmlstr)); |
||||
$xsl = $proc->importStylesheet($xsl); |
||||
$newdom = $proc->transformToDoc($input); |
||||
$dc_xml = $newdom->saveXML(); |
||||
|
||||
return $dc_xml; |
||||
} |
||||
|
||||
/** |
||||
* |
||||
* @param <string> $dir |
||||
* @return <boolean> |
||||
*/ |
||||
function recursive_directory_delete($dir) { |
||||
if (!file_exists($dir)) |
||||
return TRUE; |
||||
if (!is_dir($dir)) |
||||
return unlink($dir); |
||||
foreach (scandir($dir) as $item) { |
||||
if ($item == '.' || $item == '..') |
||||
continue; |
||||
if (!recursive_directory_delete($dir . DIRECTORY_SEPARATOR . $item)) |
||||
return FALSE; |
||||
} |
||||
return rmdir($dir); |
||||
} |
@ -1,401 +0,0 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* collection creation form |
||||
* @param array $form_state |
||||
* @param string $parent_collection_pid |
||||
* @param string $content_models |
||||
* @return array |
||||
*/ |
||||
function collection_management_form(&$form_state, $this_collection_pid, $content_models) { |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_utils'); |
||||
$restricted = FALSE; |
||||
if (variable_get('fedora_namespace_restriction_enforced', TRUE)) { |
||||
$restricted = TRUE; |
||||
$allowed_string = variable_get('fedora_pids_allowed', 'default: demo: changeme: islandora:'); |
||||
$namespaces = explode(':', $allowed_string); |
||||
foreach ($namespaces as $namespace) { |
||||
if ($namespace) { |
||||
$allowed[trim($namespace)] = trim($namespace); |
||||
} |
||||
} |
||||
} |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_utils'); |
||||
module_load_include('inc', 'fedora_repository', 'CollectionPolicy'); |
||||
$item = new Fedora_Item($this_collection_pid); |
||||
$collection_name = $item->objectProfile->objLabel; |
||||
$new_content_models = get_content_models_as_option_array(); |
||||
$cm_options = array(); |
||||
$name_mappings = array(); |
||||
foreach ($content_models as $content_model) { |
||||
if ($content_model->pid != "islandora:collectionCModel") { |
||||
$item = new fedora_item($content_model->pid); |
||||
$cm_name = $item->objectProfile->objLabel; |
||||
$cm_options[$content_model->pid] = $cm_name; |
||||
} |
||||
} |
||||
if (!empty($cm_options)) { |
||||
$show_delete = TRUE; |
||||
} |
||||
|
||||
|
||||
$content_models = get_content_models_as_option_array(); |
||||
$form['child_creation'] = array( |
||||
'#title' => "Create Child Collection", |
||||
'#type' => 'fieldset', |
||||
'#collapsible' => TRUE, |
||||
'#collapsed' => TRUE, |
||||
); |
||||
|
||||
$form['child_creation']['titlebox'] = array( |
||||
'#type' => 'item', |
||||
'#value' => t("Create New Child Collection within $this_collection_pid"), |
||||
); |
||||
|
||||
$form['child_creation']['collection_name'] = array( |
||||
'#title' => "Collection Name", |
||||
'#type' => 'textfield', |
||||
'#description' => t("Human readable name for this collection"), |
||||
); |
||||
|
||||
$form['child_creation']['new_collection_pid'] = array( |
||||
'#title' => "Collection PID", |
||||
'#type' => 'textfield', |
||||
'#size' => 15, |
||||
'#description' => t("Unique PID for this collection. <br />Pids take the general form of namespace:collection eg islandora:pamphlets"), |
||||
); |
||||
if (!$restricted) { |
||||
$form['child_creation']['collection_namespace'] = array( |
||||
'#title' => "Collection Namespace", |
||||
'#type' => 'textfield', |
||||
'#size' => 15, |
||||
'#default_value' => 'default', |
||||
'#description' => t("Namespace for objects in this collection."), |
||||
); |
||||
} |
||||
else { |
||||
$form['child_creation']['collection_namespace'] = array( |
||||
'#title' => "Collection Namespace", |
||||
'#type' => 'select', |
||||
'#options' => $allowed, |
||||
'#default_value' => 'default', |
||||
'#description' => t("Namespace for objects in this collection."), |
||||
); |
||||
} |
||||
$form['parent_collection'] = array( |
||||
'#type' => 'hidden', |
||||
'#value' => $this_collection_pid, |
||||
); |
||||
|
||||
$form['collection_pid'] = array( |
||||
'#type' => 'hidden', |
||||
'#value' => $this_collection_pid, |
||||
); |
||||
$form['child_creation']['content_models'] = array( |
||||
'#title' => "Choose allowable content models for this collection", |
||||
'#type' => 'checkboxes', |
||||
'#options' => $content_models, |
||||
'#description' => t("Content models describe the behaviours of objects with which they are associated."), |
||||
); |
||||
|
||||
|
||||
$form['child_creation']['submit'] = array( |
||||
'#type' => 'submit', |
||||
'#value' => t('Create Collection'), |
||||
'#id' => 'create_class' |
||||
); |
||||
$form['manage_collection_policy'] = array( |
||||
'#title' => "Manage Collection Policies", |
||||
'#type' => 'fieldset', |
||||
'#collapsible' => TRUE, |
||||
'#collapsed' => TRUE, |
||||
); |
||||
|
||||
$form['manage_collection_policy']['add'] = array( |
||||
'#title' => "Add Content Model", |
||||
'#type' => 'fieldset', |
||||
'#collapsible' => TRUE, |
||||
'#collapsed' => $show_delete, |
||||
); |
||||
|
||||
|
||||
$form ['manage_collection_policy']['add']['content_model_to_add'] = array( |
||||
'#title' => "Choose Content Model", |
||||
'#type' => 'select', |
||||
'#options' => array_diff_key($content_models, $cm_options), |
||||
'#description' => t("Choose content model to add to this collection policy."), |
||||
); |
||||
|
||||
$form ['manage_collection_policy']['add']['new_cp_namespace'] = array( |
||||
'#title' => "Choose Namespace", |
||||
'#type' => 'textfield', |
||||
'#size' => 15, |
||||
'#description' => t("Choose namespace for objects in this collection associated with this content model"), |
||||
); |
||||
$form['manage_collection_policy']['add']['submit'] = array( |
||||
'#type' => 'submit', |
||||
'#value' => t('Add Content Model to Collection Policy'), |
||||
'#id' => 'add_cm' |
||||
); |
||||
|
||||
if ($show_delete) { |
||||
$form['manage_collection_policy']['remove'] = array( |
||||
'#title' => "Delete Content Model", |
||||
'#type' => 'fieldset', |
||||
'#collapsible' => TRUE, |
||||
'#collapsed' => TRUE, |
||||
); |
||||
|
||||
$form ['manage_collection_policy']['remove']['content_models_to_remove'] = array( |
||||
'#title' => "Choose Content Model to Remove", |
||||
'#type' => 'checkboxes', |
||||
'#options' => $cm_options, |
||||
'#description' => t("Choose content models to remove from this collection policy."), |
||||
); |
||||
|
||||
|
||||
$form['manage_collection_policy']['remove']['submit'] = array( |
||||
'#type' => 'submit', |
||||
'#value' => t('Remove Content Collection Policy'), |
||||
'#id' => 'remove_cm' |
||||
); |
||||
} |
||||
$form['change_cmodel'] = array( |
||||
'#title' => "Change Content Models", |
||||
'#type' => 'fieldset', |
||||
'#collapsible' => TRUE, |
||||
'#collapsed' => TRUE, |
||||
); |
||||
$form['change_cmodel']['titlebox'] = array( |
||||
'#type' => 'item', |
||||
'#value' => t("Change Content Models within $this_collection_pid"), |
||||
); |
||||
|
||||
$form['change_cmodel']['current_content_model'] = array( |
||||
'#title' => "Choose content model to be changed", |
||||
'#type' => 'select', |
||||
'#options' => $cm_options, |
||||
'#description' => t("All objects in this collection with the selected content model will be changed."), |
||||
); |
||||
$form['change_cmodel']['new_content_model'] = array( |
||||
'#title' => "Choose new content model", |
||||
'#type' => 'select', |
||||
'#options' => $new_content_models, |
||||
'#description' => t("The new content model to be assigned to selected objects."), |
||||
); |
||||
$form['change_cmodel']['collection_pid'] = array( |
||||
'#type' => 'hidden', |
||||
'#value' => $this_collection_pid, |
||||
); |
||||
$form['change_cmodel']['submit'] = array( |
||||
'#type' => 'submit', |
||||
'#value' => t('Change Content Model Associations'), |
||||
'#id' => 'change_model', |
||||
); |
||||
if (user_access('delete entire collections')) { |
||||
$form['delete_collection'] = array( |
||||
'#title' => "Permanently Delete $collection_name", |
||||
'#type' => 'fieldset', |
||||
'#description' => t("Clicking this button will delete all objects within $collection_name. <br /> <strong>This action cannot be undone.</strong>"), |
||||
'#collapsible' => TRUE, |
||||
'#collapsed' => TRUE, |
||||
); |
||||
$form['delete_collection']['confirm'] = array( |
||||
'#title' => "Are you sure?", |
||||
'#type' => 'fieldset', |
||||
'#description' => t('<strong>Clicking the delete button will permanantly remove all objects from this collection. <br /> <strong>This action cannot be undone.</strong> '), |
||||
'#collapsible' => TRUE, |
||||
'#collapsed' => TRUE, |
||||
); |
||||
|
||||
$form['delete_collection']['confirm']['submit'] = array( |
||||
'#type' => 'submit', |
||||
'#value' => t('Delete this collection'), |
||||
'#id' => 'delete_collection', |
||||
); |
||||
} |
||||
return($form); |
||||
} |
||||
|
||||
/** |
||||
* collection creation form validate |
||||
* @param array $form |
||||
* @param array $form_state |
||||
*/ |
||||
function collection_management_form_validate($form, &$form_state) { |
||||
if ($form_state['clicked_button']['#id'] == 'create_class') { |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_item'); |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_utils'); |
||||
$pid = $form_state['values']['new_collection_pid']; |
||||
$item = new fedora_item($pid); |
||||
if ($item->exists()) { |
||||
form_set_error('new_collection_pid', t("$pid already exists within your repository. the PID must be unique")); |
||||
return; |
||||
} |
||||
if (!valid_pid($pid)) { |
||||
form_set_error('new_collection_pid', t("$pid is not a valid identifier")); |
||||
return; |
||||
} |
||||
} |
||||
if ($form_state['clicked_button']['#id'] == 'add_cm') { |
||||
if (!valid_pid($form_state['values']['new_cp_namespace'])) { |
||||
form_set_error('new_cp_namespace', t("Namespace must be a valid PID")); |
||||
return; |
||||
} |
||||
} |
||||
} |
||||
|
||||
/** |
||||
* collection creation form submit |
||||
* @global type $user |
||||
* @param type $form |
||||
* @param type $form_state |
||||
*/ |
||||
function collection_management_form_submit($form, &$form_state) { |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_collection'); |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_item'); |
||||
module_load_include('inc', 'fedora_repository', 'api/dublin_core'); |
||||
global $user; |
||||
$collection_pid = $form_state['values']['parent_collection']; |
||||
$policy = CollectionPolicy::loadFromCollection($collection_pid, TRUE); |
||||
if ($form_state['clicked_button']['#id'] == 'create_class') { |
||||
$module_path = drupal_get_path('module', 'fedora_repository'); |
||||
$thumbnail = drupal_get_path('module', 'Fedora_Repository') . '/images/Crystal_Clear_filesystem_folder_grey.png'; |
||||
$new_collection_pid = $form_state['values']['new_collection_pid']; |
||||
$new_collection_label = $form_state['values']['collection_name']; |
||||
$pid_namespace = $form_state['values']['collection_namespace']; |
||||
$all_cModels = get_content_models_as_option_array(); |
||||
$collection_policy = '<?xml version="1.0" encoding="UTF-8"?> |
||||
<collection_policy xmlns="http://www.islandora.ca" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" name="" xsi:schemaLocation="http://www.islandora.ca http://syn.lib.umanitoba.ca/collection_policy.xsd"> |
||||
<content_models> |
||||
</content_models> |
||||
<search_terms> |
||||
</search_terms> |
||||
<staging_area></staging_area> |
||||
<relationship>isMemberOfCollection</relationship> |
||||
</collection_policy>'; |
||||
$content_models = $form_state['values']['content_models']; |
||||
$collection_policy_xml = simplexml_load_string($collection_policy); |
||||
foreach ($content_models as $content_model) { |
||||
if ($content_model) { |
||||
$node = $collection_policy_xml->content_models->addChild('content_model'); |
||||
$node->addAttribute('dsid', 'ISLANDORACM'); |
||||
$node->addAttribute('name', $all_cModels[$content_model]); |
||||
$node->addAttribute('namespace', $pid_namespace . ':1'); |
||||
$node->addAttribute('pid', $content_model); |
||||
} |
||||
} |
||||
$item = fedora_item::ingest_new_item($new_collection_pid, 'A', $new_collection_label, $user->name); |
||||
$item->add_relationship('isMemberOfCollection', $collection_pid, RELS_EXT_URI); |
||||
$item->add_relationship('hasModel', 'islandora:collectionCModel', FEDORA_MODEL_URI); |
||||
$item->add_datastream_from_string($collection_policy_xml->saveXML(), 'COLLECTION_POLICY'); |
||||
$item->add_datastream_from_file($thumbnail, 'TN'); |
||||
drupal_goto("/fedora/repository/$new_collection_pid"); |
||||
} |
||||
|
||||
if ($form_state['clicked_button']['#id'] == 'add_cm') { |
||||
|
||||
$cp_namespace = $form_state['values']['new_cp_namespace']; |
||||
$cp_content_model = $form_state['values']['content_model_to_add']; |
||||
$policy->addModel(ContentModel::loadFromModel($cp_content_model), $cp_namespace); |
||||
$policy->saveToFedora(); |
||||
drupal_set_message("Collection model successfully added"); |
||||
} |
||||
|
||||
if ($form_state['clicked_button']['#id'] == 'remove_cm') { |
||||
$candidates = $form_state['values']['content_models_to_remove']; |
||||
$count = 0; |
||||
foreach ($candidates as $candidate) { |
||||
if (is_string($candidate)) { |
||||
$policy->removeModel(ContentModel::loadFromModel($candidate)); |
||||
$count++; |
||||
} |
||||
} |
||||
if ($count > 0) { |
||||
$policy->saveToFedora(); |
||||
if ($count > 1) { |
||||
$s = 's'; |
||||
} |
||||
drupal_set_message("$count collection model$s removed"); |
||||
} |
||||
} |
||||
|
||||
if ($form_state['clicked_button']['#id'] == 'change_model') { |
||||
$current_content_model = $form_state['values']['current_content_model']; |
||||
$new_content_model = $form_state['values']['new_content_model']; |
||||
|
||||
$add_to_policy = TRUE; |
||||
$policy_cms = $policy->getContentModels(); |
||||
foreach ($policy_cms as $policy_cm) { |
||||
if ($policy_cm->pid == $current_content_model) { |
||||
$namespace = $policy_cm->pid_namespace; |
||||
} |
||||
if ($policy_cm->pid == $new_content_model) { |
||||
$add_to_policy = FALSE; |
||||
} |
||||
} |
||||
if ($add_to_policy) { |
||||
$policy->addModel(ContentModel::loadFromModel($new_content_model), $namespace); |
||||
$policy->saveToFedora(); |
||||
} |
||||
$query = "select \$object from <#ri> |
||||
where (\$object <info:fedora/fedora-system:def/model#hasModel> <info:fedora/$current_content_model> |
||||
and \$object <info:fedora/fedora-system:def/relations-external#isMemberOfCollection> <info:fedora/$collection_pid> |
||||
and \$object <fedora-model:state> <info:fedora/fedora-system:def/model#Active>)"; |
||||
$query = htmlentities(urlencode($query)); |
||||
$content = ''; |
||||
|
||||
$url = variable_get('fedora_repository_url', 'http://localhost:8080/fedora/risearch'); |
||||
$url .= "?type=tuples&flush=TRUE&format=csv&limit=$limit&offset=$offset&lang=itql&stream=on&query=" . $query; |
||||
$content .= do_curl($url); |
||||
$results = explode("\n", $content); |
||||
$object_pids = preg_replace('/^info:fedora\/|"object"/', '', $results); |
||||
$count = 0; |
||||
foreach ($object_pids as $object_pid) { |
||||
if (!$object_pid) { |
||||
continue; |
||||
} |
||||
$item = new fedora_item($object_pid); |
||||
$item->purge_relationship('hasModel', $current_content_model); |
||||
$item->add_relationship('hasModel', $new_content_model, FEDORA_MODEL_URI); |
||||
$count++; |
||||
} |
||||
drupal_set_message("$current_content_model changed to $new_content_model on $count objects"); |
||||
} |
||||
|
||||
if ($form_state['clicked_button']['#id'] == 'delete_collection') { |
||||
$collection_name = $form_state['values']['collection_name']; |
||||
$pids = get_related_items_as_array($collection_pid, 'isMemberOfCollection'); |
||||
|
||||
$batch = array( |
||||
'title' => "Deleting Objects from $name", |
||||
'operations' => array(), |
||||
'file' => drupal_get_path('module', 'fedora_repository') . '/CollectionManagement.inc', |
||||
); |
||||
|
||||
|
||||
foreach ($pids as $pid) { |
||||
|
||||
$batch['operations'][] = array('delete_objects_as_batch', array($pid)); |
||||
} |
||||
|
||||
batch_set($batch); |
||||
batch_process('/fedora/repository'); |
||||
} |
||||
} |
||||
|
||||
/** |
||||
* deletes PID if pid is not collection |
||||
* @param <type> $pid |
||||
*/ |
||||
function delete_objects_as_batch($pid) { |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_item'); |
||||
$name = $user->name; |
||||
$item_to_delete = new Fedora_Item($pid); |
||||
$models = $item_to_delete->get_models(); |
||||
foreach ($models as $model) { |
||||
if ($model['object'] != 'islandora:collectionCModel') { |
||||
$item_to_delete->purge("$object purged by $name"); |
||||
} |
||||
} |
||||
} |
@ -1,9 +1,8 @@
|
||||
; $Id$ |
||||
name = Islandora Repository |
||||
dependencies[] = imageapi |
||||
dependencies[] = tabs |
||||
dependencies[] = islandora_content_model_forms |
||||
description = Shows a list of items in a fedora collection. |
||||
package = Islandora |
||||
version = 11.2.0 |
||||
version = 11.3.1 |
||||
core = 6.x |
||||
|
Loading…
Reference in new issue