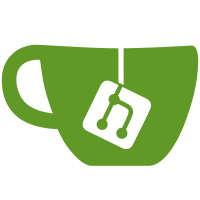
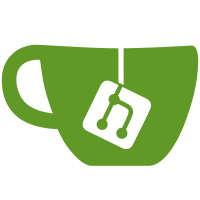
4 changed files with 1 additions and 234 deletions
@ -1,90 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace Drupal\islandora\Controller; |
|
||||||
|
|
||||||
use Drupal\Core\Cache\CacheableJsonResponse; |
|
||||||
use Drupal\Core\Controller\ControllerBase; |
|
||||||
use Drupal\jsonld\ContextGenerator\JsonldContextGeneratorInterface; |
|
||||||
use Symfony\Component\DependencyInjection\ContainerInterface; |
|
||||||
use Symfony\Component\HttpFoundation\Request; |
|
||||||
use Symfony\Component\HttpFoundation\Response; |
|
||||||
use Drupal\rdf\Entity\RdfMapping; |
|
||||||
use Drupal\Core\Cache\Cache; |
|
||||||
use Drupal\Core\Cache\CacheableMetadata; |
|
||||||
|
|
||||||
/** |
|
||||||
* Class JsonLdContextController. |
|
||||||
* |
|
||||||
* @package Drupal\islandora\Controller |
|
||||||
*/ |
|
||||||
class JsonLdContextController extends ControllerBase { |
|
||||||
|
|
||||||
/** |
|
||||||
* Injected JsonldContextGenerator. |
|
||||||
* |
|
||||||
* @var \Drupal\jsonld\ContextGenerator\JsonldContextGeneratorInterface |
|
||||||
*/ |
|
||||||
private $jsonldContextGenerator; |
|
||||||
|
|
||||||
/** |
|
||||||
* JsonLdContextController constructor. |
|
||||||
* |
|
||||||
* @param \Drupal\jsonld\ContextGenerator\JsonldContextGeneratorInterface $jsonld_context_generator |
|
||||||
* Injected JsonldContextGenerator. |
|
||||||
*/ |
|
||||||
public function __construct(JsonldContextGeneratorInterface $jsonld_context_generator) { |
|
||||||
$this->jsonldContextGenerator = $jsonld_context_generator; |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* Controller's create method for dependecy injection. |
|
||||||
* |
|
||||||
* @param \Symfony\Component\DependencyInjection\ContainerInterface $container |
|
||||||
* The App Container. |
|
||||||
* |
|
||||||
* @return static |
|
||||||
* An instance of our jsonld.contextgenerator service. |
|
||||||
*/ |
|
||||||
public static function create(ContainerInterface $container) { |
|
||||||
return new static($container->get('jsonld.contextgenerator')); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* Returns an JSON-LD Context for a entity bundle. |
|
||||||
* |
|
||||||
* @param string $entity_type |
|
||||||
* Route argument, an entity type. |
|
||||||
* @param string $bundle |
|
||||||
* Route argument, a bundle. |
|
||||||
* @param \Symfony\Component\HttpFoundation\Request $request |
|
||||||
* The Symfony Http Request. |
|
||||||
* |
|
||||||
* @return \Symfony\Component\HttpFoundation\Response |
|
||||||
* An Http response. |
|
||||||
*/ |
|
||||||
public function content($entity_type, $bundle, Request $request) { |
|
||||||
|
|
||||||
// TODO: expose cached/not cached through |
|
||||||
// more varied HTTP response codes. |
|
||||||
try { |
|
||||||
$context = $this->jsonldContextGenerator->getContext("$entity_type.$bundle"); |
|
||||||
$response = new CacheableJsonResponse(json_decode($context), 200); |
|
||||||
$response->setEncodingOptions(JSON_UNESCAPED_UNICODE | JSON_UNESCAPED_SLASHES | JSON_NUMERIC_CHECK); |
|
||||||
$response->headers->set('X-Powered-By', 'Islandora CLAW API'); |
|
||||||
$response->headers->set('Content-Type', 'application/ld+json'); |
|
||||||
|
|
||||||
// For now deal with Cache dependencies manually. |
|
||||||
$meta = new CacheableMetadata(); |
|
||||||
$meta->setCacheContexts(['user.permissions', 'ip', 'url']); |
|
||||||
$meta->setCacheTags(RdfMapping::load("$entity_type.$bundle")->getCacheTags()); |
|
||||||
$meta->setCacheMaxAge(Cache::PERMANENT); |
|
||||||
$response->addCacheableDependency($meta); |
|
||||||
} |
|
||||||
catch (\Exception $e) { |
|
||||||
$response = new Response($e->getMessage(), 400); |
|
||||||
} |
|
||||||
|
|
||||||
return $response; |
|
||||||
} |
|
||||||
|
|
||||||
} |
|
@ -1,41 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace Drupal\islandora\Tests\Web; |
|
||||||
|
|
||||||
use Drupal\simpletest\WebTestBase; |
|
||||||
|
|
||||||
/** |
|
||||||
* Abstract base class for Islandora Web tests. |
|
||||||
*/ |
|
||||||
abstract class IslandoraWebTestBase extends WebTestBase { |
|
||||||
|
|
||||||
/** |
|
||||||
* {@inheritdoc} |
|
||||||
*/ |
|
||||||
public static $modules = [ |
|
||||||
'system', |
|
||||||
'user', |
|
||||||
'field', |
|
||||||
'filter', |
|
||||||
'block', |
|
||||||
'node', |
|
||||||
'path', |
|
||||||
'text', |
|
||||||
'options', |
|
||||||
'inline_entity_form', |
|
||||||
'serialization', |
|
||||||
'rest', |
|
||||||
'rdf', |
|
||||||
'action', |
|
||||||
'context', |
|
||||||
'context_ui', |
|
||||||
'jsonld', |
|
||||||
'views', |
|
||||||
'key', |
|
||||||
'jwt', |
|
||||||
'basic_auth', |
|
||||||
'filehash', |
|
||||||
'islandora', |
|
||||||
]; |
|
||||||
|
|
||||||
} |
|
@ -1,94 +0,0 @@ |
|||||||
<?php |
|
||||||
|
|
||||||
namespace Drupal\islandora\Tests\Web; |
|
||||||
|
|
||||||
use Drupal\Core\Url; |
|
||||||
use Drupal\node\Entity\NodeType; |
|
||||||
use Drupal\rdf\Entity\RdfMapping; |
|
||||||
|
|
||||||
/** |
|
||||||
* Implements WEB tests for Context routing response in various scenarios. |
|
||||||
* |
|
||||||
* @group islandora |
|
||||||
*/ |
|
||||||
class JsonldContextGeneratorWebTest extends IslandoraWebTestBase { |
|
||||||
|
|
||||||
/** |
|
||||||
* A user entity. |
|
||||||
* |
|
||||||
* @var \Drupal\Core\Session\AccountInterface |
|
||||||
*/ |
|
||||||
private $user; |
|
||||||
|
|
||||||
/** |
|
||||||
* Jwt does not define a config schema breaking this tests. |
|
||||||
* |
|
||||||
* @var bool |
|
||||||
*/ |
|
||||||
protected $strictConfigSchema = FALSE; |
|
||||||
|
|
||||||
/** |
|
||||||
* Initial setup tasks that for every method method. |
|
||||||
*/ |
|
||||||
public function setUp() { |
|
||||||
parent::setUp(); |
|
||||||
|
|
||||||
$test_type = NodeType::create([ |
|
||||||
'type' => 'test_type', |
|
||||||
'label' => 'Test Type', |
|
||||||
]); |
|
||||||
$test_type->save(); |
|
||||||
|
|
||||||
// Give it a basic rdf mapping. |
|
||||||
$mapping = RdfMapping::create([ |
|
||||||
'id' => 'node.test_type', |
|
||||||
'targetEntityType' => 'node', |
|
||||||
'bundle' => 'test_type', |
|
||||||
'types' => ['schema:Thing'], |
|
||||||
'fieldMappings' => [ |
|
||||||
'title' => [ |
|
||||||
'properties' => ['dc11:title'], |
|
||||||
], |
|
||||||
], |
|
||||||
]); |
|
||||||
$mapping->save(); |
|
||||||
|
|
||||||
$this->user = $this->drupalCreateUser([ |
|
||||||
'administer site configuration', |
|
||||||
'administer nodes', |
|
||||||
]); |
|
||||||
|
|
||||||
// Login. |
|
||||||
$this->drupalLogin($this->user); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* Tests that the Context Response Page can be reached. |
|
||||||
*/ |
|
||||||
public function testJsonldcontextPageExists() { |
|
||||||
$url = Url::fromRoute('islandora.jsonldcontext', ['entity_type' => 'node', 'bundle' => 'test_type']); |
|
||||||
$this->drupalGet($url); |
|
||||||
$this->assertResponse(200); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* Tests that the response is in fact application/ld+json. |
|
||||||
*/ |
|
||||||
public function testJsonldcontextContentypeheaderResponseIsValid() { |
|
||||||
$url = Url::fromRoute('islandora.jsonldcontext', ['entity_type' => 'node', 'bundle' => 'test_type']); |
|
||||||
$this->drupalGet($url); |
|
||||||
$this->assertEqual($this->drupalGetHeader('Content-Type'), 'application/ld+json', 'Correct JSON-LD mime type was returned'); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* Tests that the Context received has the basic structural needs. |
|
||||||
*/ |
|
||||||
public function testJsonldcontextResponseIsValid() { |
|
||||||
$url = Url::fromRoute('islandora.jsonldcontext', ['entity_type' => 'node', 'bundle' => 'test_type']); |
|
||||||
$this->drupalGet($url); |
|
||||||
$jsonldarray = json_decode($this->getRawContent(), TRUE); |
|
||||||
// Check if the only key is "@context". |
|
||||||
$this->assertTrue(count(array_keys($jsonldarray)) == 1 && (key($jsonldarray) == '@context'), "JSON-LD to array encoded response has just one key and that key is @context"); |
|
||||||
} |
|
||||||
|
|
||||||
} |
|
Loading…
Reference in new issue