Browse Source
* Add remove fedora_has_parent function Remove fedora_has_parent if the parent is deleted * Coding style * What the WHAT * Change variables to use less node-tastic terminology * Adding tests Fixing setParent type hint Fixing hasParent logic * Coder * Fix parent clean up test. * Coderpull/756/head
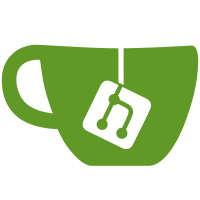
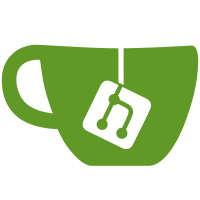
4 changed files with 277 additions and 6 deletions
@ -0,0 +1,92 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\Tests\islandora\Kernel; |
||||
|
||||
use Drupal\islandora\Entity\FedoraResource; |
||||
use Drupal\simpletest\UserCreationTrait; |
||||
|
||||
/** |
||||
* Tests the clean up of deleted parent referenced from children. |
||||
* |
||||
* @group islandora |
||||
* @coversDefaultClass \Drupal\islandora\Entity\FedoraResource |
||||
*/ |
||||
class DeleteFedoraResourceWithParentsTest extends IslandoraKernelTestBase { |
||||
|
||||
use UserCreationTrait; |
||||
|
||||
/** |
||||
* Parent Fedora resource entity. |
||||
* |
||||
* @var \Drupal\islandora\FedoraResourceInterface |
||||
*/ |
||||
protected $parentEntity; |
||||
|
||||
/** |
||||
* Child Fedora resource entity. |
||||
* |
||||
* @var \Drupal\islandora\FedoraResourceInterface |
||||
*/ |
||||
protected $childEntity; |
||||
|
||||
/** |
||||
* User entity. |
||||
* |
||||
* @var \Drupal\user\UserInterface |
||||
*/ |
||||
protected $user; |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function setUp() { |
||||
parent::setUp(); |
||||
$permissions = [ |
||||
'add fedora resource entities', |
||||
'edit fedora resource entities', |
||||
'delete fedora resource entities', |
||||
]; |
||||
$this->assertTrue($this->checkPermissions($permissions), 'Permissions are invalid'); |
||||
|
||||
$this->user = $this->createUser($permissions); |
||||
|
||||
// Create a test entity. |
||||
$this->parentEntity = FedoraResource::create([ |
||||
"type" => "rdf_source", |
||||
"uid" => $this->user->get('uid'), |
||||
"name" => "Test Parent", |
||||
"langcode" => "und", |
||||
"status" => 1, |
||||
]); |
||||
$this->parentEntity->save(); |
||||
|
||||
$this->childEntity = FedoraResource::create([ |
||||
"type" => "rdf_source", |
||||
"uid" => $this->user->get('uid'), |
||||
"name" => "Test Child", |
||||
"langcode" => "und", |
||||
"fedora_has_parent" => $this->parentEntity, |
||||
"status" => 1, |
||||
]); |
||||
$this->childEntity->save(); |
||||
} |
||||
|
||||
/** |
||||
* Tests cleaning up child to parent references when parent is deleted. |
||||
* |
||||
* @covers \Drupal\islandora\Entity\FedoraResource::postDelete |
||||
*/ |
||||
public function testCleanUpParents() { |
||||
$child_id = $this->childEntity->id(); |
||||
// Load the child entity. |
||||
$new_child = FedoraResource::load($child_id); |
||||
// Verify it has a parent. |
||||
$this->assertFalse($new_child->get('fedora_has_parent')->isEmpty(), "Should have a parent."); |
||||
// Delete the parent entity. |
||||
$this->parentEntity->delete(); |
||||
// Verify we don't have a parent anymore. |
||||
$this->assertTrue($new_child->get('fedora_has_parent')->isEmpty(), "Should not have a parent."); |
||||
|
||||
} |
||||
|
||||
} |
@ -0,0 +1,134 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\Tests\islandora\Kernel; |
||||
|
||||
use Drupal\islandora\Entity\FedoraResource; |
||||
use Drupal\simpletest\UserCreationTrait; |
||||
|
||||
/** |
||||
* Tests for adding, removing and testing for parents. |
||||
* |
||||
* @group islandora |
||||
* @coversDefaultClass \Drupal\islandora\Entity\FedoraResource |
||||
*/ |
||||
class FedoraResourceParentTest extends IslandoraKernelTestBase { |
||||
|
||||
use UserCreationTrait; |
||||
|
||||
/** |
||||
* A Drupal user. |
||||
* |
||||
* @var \Drupal\user\UserInterface |
||||
*/ |
||||
protected $user; |
||||
|
||||
/** |
||||
* A Fedora Resource. |
||||
* |
||||
* @var \Drupal\islandora\Entity\FedoraResource |
||||
*/ |
||||
protected $entity; |
||||
|
||||
/** |
||||
* Another Fedora Resource. |
||||
* |
||||
* @var \Drupal\islandora\Entity\FedoraResource |
||||
*/ |
||||
protected $parentEntity; |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function setUp() { |
||||
parent::setUp(); |
||||
|
||||
$permissions = [ |
||||
'add fedora resource entities', |
||||
'edit fedora resource entities', |
||||
'delete fedora resource entities', |
||||
]; |
||||
$this->assertTrue($this->checkPermissions($permissions), 'Permissions are invalid'); |
||||
|
||||
$this->user = $this->createUser($permissions); |
||||
|
||||
$this->entity = FedoraResource::create([ |
||||
'type' => 'rdf_source', |
||||
'uid' => $this->user->get('uid'), |
||||
'name' => 'Test Entity', |
||||
'langcode' => 'und', |
||||
'status' => 1, |
||||
]); |
||||
$this->entity->save(); |
||||
|
||||
$this->parentEntity = FedoraResource::create([ |
||||
'type' => 'rdf_source', |
||||
'uid' => $this->user->get('uid'), |
||||
'name' => 'Parent Entity', |
||||
'langcode' => 'und', |
||||
'status' => 1, |
||||
]); |
||||
$this->parentEntity->save(); |
||||
} |
||||
|
||||
/** |
||||
* @covers \Drupal\islandora\Entity\FedoraResource::setParent |
||||
*/ |
||||
public function testSetParent() { |
||||
$this->assertTrue($this->entity->get('fedora_has_parent')->isEmpty(), "Entity has an unexpected parent."); |
||||
|
||||
$this->entity->setParent($this->parentEntity); |
||||
$this->entity->save(); |
||||
|
||||
$this->assertFalse($this->entity->get('fedora_has_parent')->isEmpty(), "Entity has no parent."); |
||||
} |
||||
|
||||
/** |
||||
* @covers \Drupal\islandora\Entity\FedoraResource::removeParent |
||||
*/ |
||||
public function testRemoveParent() { |
||||
$this->assertTrue($this->entity->get('fedora_has_parent')->isEmpty(), "Entity has an unexpected parent."); |
||||
|
||||
$this->entity->set('fedora_has_parent', $this->parentEntity); |
||||
$this->entity->save(); |
||||
|
||||
$this->assertFalse($this->entity->get('fedora_has_parent')->isEmpty(), "Entity has no parent."); |
||||
|
||||
$this->entity->removeParent(); |
||||
$this->entity->save(); |
||||
|
||||
$this->assertTrue($this->entity->get('fedora_has_parent')->isEmpty(), "Entity has an unexpected parent."); |
||||
} |
||||
|
||||
/** |
||||
* @covers \Drupal\islandora\Entity\FedoraResource::hasParent |
||||
*/ |
||||
public function testHasParent() { |
||||
$this->assertTrue($this->entity->get('fedora_has_parent')->isEmpty(), "Entity has an unexpected parent."); |
||||
$this->assertFalse($this->entity->hasParent(), "hasParent is reporting a parent incorrectly."); |
||||
|
||||
$this->entity->set('fedora_has_parent', $this->parentEntity); |
||||
$this->entity->save(); |
||||
|
||||
$this->assertFalse($this->entity->get('fedora_has_parent')->isEmpty(), "Entity has no parent."); |
||||
$this->assertTrue($this->entity->hasParent(), "hasParent is reporting NO parent incorrectly."); |
||||
|
||||
$this->entity->set('fedora_has_parent', NULL); |
||||
$this->entity->save(); |
||||
|
||||
$this->assertTrue($this->entity->get('fedora_has_parent')->isEmpty(), "Entity still has a parent."); |
||||
$this->assertFalse($this->entity->hasParent(), "hasParent is reporting a parent incorrectly."); |
||||
} |
||||
|
||||
/** |
||||
* @covers \Drupal\islandora\Entity\FedoraResource::getParentId |
||||
*/ |
||||
public function testGetParentId() { |
||||
$id = $this->parentEntity->id(); |
||||
|
||||
$this->entity->set('fedora_has_parent', $this->parentEntity); |
||||
$this->entity->save(); |
||||
|
||||
$this->assertEquals($id, $this->entity->getParentId(), "Did not get correct parent id."); |
||||
} |
||||
|
||||
} |
Loading…
Reference in new issue