Browse Source
* initial commit * break looped chains * add depthLimit and includeSelf configuration * clean up configuration * breadcrumb tests * enable tests in travis * fix travis testspull/729/head
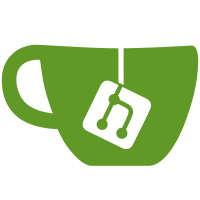
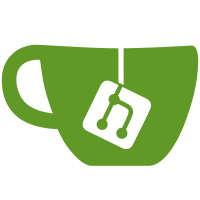
7 changed files with 214 additions and 0 deletions
@ -0,0 +1,3 @@ |
|||||||
|
maxDepth: -1 |
||||||
|
includeSelf: FALSE |
||||||
|
referenceField: field_member_of |
@ -0,0 +1,12 @@ |
|||||||
|
islandora.breadcrumbs: |
||||||
|
type: config_object |
||||||
|
mapping: |
||||||
|
maxDepth: |
||||||
|
type: integer |
||||||
|
label: 'Max Depth' |
||||||
|
includeSelf: |
||||||
|
type: boolean |
||||||
|
label: 'Include Self' |
||||||
|
referenceField: |
||||||
|
type: string |
||||||
|
label: 'Reference Field' |
@ -0,0 +1,7 @@ |
|||||||
|
name: 'Islandora Breadcrumbs' |
||||||
|
type: module |
||||||
|
description: 'Builds breadcrumbs based on field_member_of relationships.' |
||||||
|
core: 8.x |
||||||
|
package: Islandora |
||||||
|
dependencies: |
||||||
|
- islandora |
@ -0,0 +1,6 @@ |
|||||||
|
services: |
||||||
|
islandora_breadcrumbs.breadcrumb: |
||||||
|
class: Drupal\islandora_breadcrumbs\IslandoraBreadcrumbBuilder |
||||||
|
arguments: ['@config.factory'] |
||||||
|
tags: |
||||||
|
- { name: breadcrumb_builder, priority: 100 } |
@ -0,0 +1,96 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Drupal\islandora_breadcrumbs; |
||||||
|
|
||||||
|
use Drupal\Core\Config\ConfigFactoryInterface; |
||||||
|
use Drupal\Core\Entity\EntityInterface; |
||||||
|
use Drupal\Core\Breadcrumb\Breadcrumb; |
||||||
|
use Drupal\Core\Breadcrumb\BreadcrumbBuilderInterface; |
||||||
|
use Drupal\Core\Routing\RouteMatchInterface; |
||||||
|
|
||||||
|
/** |
||||||
|
* Provides breadcrumbs for nodes using a configured entity reference field. |
||||||
|
*/ |
||||||
|
class IslandoraBreadcrumbBuilder implements BreadcrumbBuilderInterface { |
||||||
|
|
||||||
|
/** |
||||||
|
* The configuration. |
||||||
|
* |
||||||
|
* @var \Drupal\Core\Config\ImmutableConfig |
||||||
|
*/ |
||||||
|
protected $config; |
||||||
|
|
||||||
|
/** |
||||||
|
* Constructs a breadcrumb builder. |
||||||
|
* |
||||||
|
* @param \Drupal\Core\Config\ConfigFactoryInterface $config_factory |
||||||
|
* The configuration factory. |
||||||
|
*/ |
||||||
|
public function __construct(ConfigFactoryInterface $config_factory) { |
||||||
|
$this->config = $config_factory->get('islandora.breadcrumbs'); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function applies(RouteMatchInterface $attributes) { |
||||||
|
$parameters = $attributes->getParameters()->all(); |
||||||
|
if (!empty($parameters['node'])) { |
||||||
|
return ($parameters['node']->hasField($this->config->get('referenceField')) && |
||||||
|
!$parameters['node']->get($this->config->get('referenceField'))->isEmpty()); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function build(RouteMatchInterface $route_match) { |
||||||
|
|
||||||
|
$node = $route_match->getParameter('node'); |
||||||
|
$breadcrumb = new Breadcrumb(); |
||||||
|
|
||||||
|
$chain = []; |
||||||
|
$this->walkMembership($node, $chain); |
||||||
|
|
||||||
|
if (!$this->config->get('includeSelf')) { |
||||||
|
array_pop($chain); |
||||||
|
} |
||||||
|
$breadcrumb->addCacheableDependency($node); |
||||||
|
|
||||||
|
// Add membership chain to the breadcrumb. |
||||||
|
foreach ($chain as $chainlink) { |
||||||
|
$breadcrumb->addCacheableDependency($chainlink); |
||||||
|
$breadcrumb->addLink($chainlink->toLink()); |
||||||
|
} |
||||||
|
$breadcrumb->addCacheContexts(['route']); |
||||||
|
return $breadcrumb; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* Follows chain of field_member_of links. |
||||||
|
* |
||||||
|
* We pass crumbs by reference to enable checking for looped chains. |
||||||
|
*/ |
||||||
|
protected function walkMembership(EntityInterface $entity, &$crumbs) { |
||||||
|
// Avoid infinate loops, return if we've seen this before. |
||||||
|
foreach ($crumbs as $crumb) { |
||||||
|
if ($crumb->uuid == $entity->uuid) { |
||||||
|
return; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
// Add this item onto the pile. |
||||||
|
array_unshift($crumbs, $entity); |
||||||
|
|
||||||
|
if ($this->config->get('maxDepth') > 0 && count($crumbs) >= $this->config->get('maxDepth')) { |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
// Find the next in the chain, if there are any. |
||||||
|
if ($entity->hasField($this->config->get('referenceField')) && |
||||||
|
!$entity->get($this->config->get('referenceField'))->isEmpty()) { |
||||||
|
$this->walkMembership($entity->get($this->config->get('referenceField'))->entity, $crumbs); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,89 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace Drupal\Tests\islandora_breadcrumbs\Functional; |
||||||
|
|
||||||
|
use Drupal\Tests\islandora\Functional\IslandoraFunctionalTestBase; |
||||||
|
use Drupal\Tests\system\Functional\Menu\AssertBreadcrumbTrait; |
||||||
|
|
||||||
|
/** |
||||||
|
* Tests the Islandora Breadcrumbs Builder. |
||||||
|
* |
||||||
|
* @group islandora_breadcrumbs |
||||||
|
*/ |
||||||
|
class BreadcrumbsTest extends IslandoraFunctionalTestBase { |
||||||
|
|
||||||
|
use AssertBreadcrumbTrait; |
||||||
|
|
||||||
|
/** |
||||||
|
* Modules to enable. |
||||||
|
* |
||||||
|
* @var array |
||||||
|
*/ |
||||||
|
public static $modules = [ |
||||||
|
'islandora_breadcrumbs', |
||||||
|
]; |
||||||
|
|
||||||
|
|
||||||
|
protected $nodeA; |
||||||
|
|
||||||
|
protected $nodeB; |
||||||
|
|
||||||
|
protected $nodeC; |
||||||
|
|
||||||
|
protected $nodeD; |
||||||
|
|
||||||
|
/** |
||||||
|
* {@inheritdoc} |
||||||
|
*/ |
||||||
|
public function setUp() { |
||||||
|
parent::setUp(); |
||||||
|
|
||||||
|
// Create some nodes. |
||||||
|
$this->nodeA = $this->container->get('entity_type.manager')->getStorage('node')->create([ |
||||||
|
'type' => $this->testType->id(), |
||||||
|
'title' => 'Node A', |
||||||
|
]); |
||||||
|
$this->nodeA->save(); |
||||||
|
|
||||||
|
$this->nodeB = $this->container->get('entity_type.manager')->getStorage('node')->create([ |
||||||
|
'type' => $this->testType->id(), |
||||||
|
'title' => 'Node B', |
||||||
|
]); |
||||||
|
$this->nodeB->set('field_member_of', [$this->nodeA->id()]); |
||||||
|
$this->nodeB->save(); |
||||||
|
|
||||||
|
$this->nodeC = $this->container->get('entity_type.manager')->getStorage('node')->create([ |
||||||
|
'type' => $this->testType->id(), |
||||||
|
'title' => 'Node C', |
||||||
|
]); |
||||||
|
$this->nodeC->set('field_member_of', [$this->nodeB->id()]); |
||||||
|
$this->nodeC->save(); |
||||||
|
|
||||||
|
$this->nodeD = $this->container->get('entity_type.manager')->getStorage('node')->create([ |
||||||
|
'type' => $this->testType->id(), |
||||||
|
'title' => 'Node D', |
||||||
|
]); |
||||||
|
$this->nodeD->set('field_member_of', [$this->nodeC->id()]); |
||||||
|
$this->nodeD->save(); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* @covers \Drupal\islandora_breadcrumbs\IslandoraBreadcrumbBuilder::applies |
||||||
|
*/ |
||||||
|
public function testDefaults() { |
||||||
|
$breadcrumbs = [ |
||||||
|
$this->nodeC->toUrl()->toString() => $this->nodeC->label(), |
||||||
|
$this->nodeB->toUrl()->toString() => $this->nodeB->label(), |
||||||
|
$this->nodeA->toUrl()->toString() => $this->nodeA->label(), |
||||||
|
]; |
||||||
|
$this->assertBreadcrumb($this->nodeD->toUrl()->toString(), $breadcrumbs); |
||||||
|
|
||||||
|
// Create a reference loop. |
||||||
|
$this->nodeA->set('field_member_of', [$this->nodeD->id()]); |
||||||
|
$this->nodeA->save(); |
||||||
|
|
||||||
|
// We should still escape it and have the same trail as before. |
||||||
|
$this->assertBreadcrumb($this->nodeD->toUrl()->toString(), $breadcrumbs); |
||||||
|
} |
||||||
|
|
||||||
|
} |
Loading…
Reference in new issue