Browse Source
* new EntityBundle condition and JsonldTypeAlterReaction * coding standards and removed logger notice * add description to formbuilder * support referenced entities that include a field_external_uripull/729/head
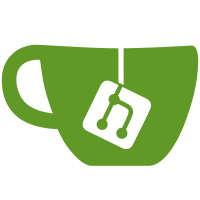
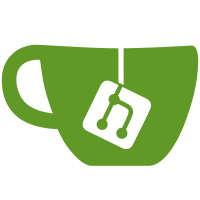
2 changed files with 188 additions and 0 deletions
@ -0,0 +1,96 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\islandora\Plugin\Condition; |
||||
|
||||
use Drupal\Core\Condition\ConditionPluginBase; |
||||
use Drupal\Core\Form\FormStateInterface; |
||||
|
||||
/** |
||||
* Provides a 'Entity Bundle' condition. |
||||
* |
||||
* @Condition( |
||||
* id = "entity_bundle", |
||||
* label = @Translation("Entity Bundle"), |
||||
* context = { |
||||
* "node" = @ContextDefinition("entity:node", label = @Translation("Node")), |
||||
* "taxonomy_term" = @ContextDefinition("entity:taxonomy_term", label = @Translation("Term")) |
||||
* } |
||||
* ) |
||||
*/ |
||||
class EntityBundle extends ConditionPluginBase { |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function buildConfigurationForm(array $form, FormStateInterface $form_state) { |
||||
$options = []; |
||||
foreach (['node', 'taxonomy_term'] as $content_entity) { |
||||
$bundles = \Drupal::service('entity_type.bundle.info')->getBundleInfo($content_entity); |
||||
foreach ($bundles as $bundle => $bundle_properties) { |
||||
$options[$bundle] = $this->t('@bundle (@type)', [ |
||||
'@bundle' => $bundle_properties['label'], |
||||
'@type' => $content_entity, |
||||
]); |
||||
} |
||||
} |
||||
|
||||
$form['bundles'] = [ |
||||
'#title' => $this->t('Bundles'), |
||||
'#type' => 'checkboxes', |
||||
'#options' => $options, |
||||
'#default_value' => $this->configuration['bundles'], |
||||
]; |
||||
|
||||
return parent::buildConfigurationForm($form, $form_state);; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function submitConfigurationForm(array &$form, FormStateInterface $form_state) { |
||||
$this->configuration['bundles'] = array_filter($form_state->getValue('bundles')); |
||||
parent::submitConfigurationForm($form, $form_state); |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function evaluate() { |
||||
foreach (\Drupal::routeMatch()->getParameters()->keys() as $type) { |
||||
if ($this->getContext($type)->hasContextValue()) { |
||||
$entity = $this->getContextValue($type); |
||||
if (!empty($this->configuration['bundles'][$entity->bundle()])) { |
||||
return !$this->isNegated(); |
||||
} |
||||
} |
||||
} |
||||
return $this->isNegated(); |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function summary() { |
||||
if (empty($this->configuration['bundles'])) { |
||||
return $this->t('No bundles are selected.'); |
||||
} |
||||
|
||||
return $this->t( |
||||
'Entity bundle in the list: @bundles', |
||||
[ |
||||
'@bundles' => implode(', ', $this->configuration['field']), |
||||
] |
||||
); |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function defaultConfiguration() { |
||||
return array_merge( |
||||
['bundles' => []], |
||||
parent::defaultConfiguration() |
||||
); |
||||
} |
||||
|
||||
} |
@ -0,0 +1,92 @@
|
||||
<?php |
||||
|
||||
namespace Drupal\islandora\Plugin\ContextReaction; |
||||
|
||||
use Drupal\Core\Entity\EntityInterface; |
||||
use Drupal\Core\Form\FormStateInterface; |
||||
use Drupal\islandora\ContextReaction\NormalizerAlterReaction; |
||||
use Drupal\jsonld\Normalizer\NormalizerBase; |
||||
|
||||
/** |
||||
* Alter JSON-LD Type context reaction. |
||||
* |
||||
* @ContextReaction( |
||||
* id = "alter_jsonld_type", |
||||
* label = @Translation("Alter JSON-LD Type") |
||||
* ) |
||||
*/ |
||||
class JsonldTypeAlterReaction extends NormalizerAlterReaction { |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function summary() { |
||||
return $this->t('Alter JSON-LD Type context reaction.'); |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function execute(EntityInterface $entity = NULL, array &$normalized = NULL, array $context = NULL) { |
||||
$config = $this->getConfiguration(); |
||||
// Use a pre-configured field as the source of the additional @type. |
||||
if (($entity->hasField($config['source_field'])) && |
||||
(!empty($entity->get($config['source_field'])->getValue()))) { |
||||
if (isset($normalized['@graph']) && is_array($normalized['@graph'])) { |
||||
foreach ($normalized['@graph'] as &$graph) { |
||||
foreach ($entity->get($config['source_field'])->getValue() as $type) { |
||||
// If the configured field is using an entity reference, |
||||
// we will see if it uses the core config's field_external_uri. |
||||
if (array_key_exists('target_id', $type)) { |
||||
$target_type = $entity->get($config['source_field'])->getFieldDefinition()->getSetting('target_type'); |
||||
$referenced_entity = \Drupal::entityTypeManager()->getStorage($target_type)->load($type['target_id']); |
||||
if ($referenced_entity->hasField('field_external_uri') && |
||||
!empty($referenced_entity->get('field_external_uri')->getValue())) { |
||||
foreach ($referenced_entity->get('field_external_uri')->getValue() as $value) { |
||||
$graph['@type'][] = $value['uri']; |
||||
} |
||||
} |
||||
} |
||||
else { |
||||
$graph['@type'][] = NormalizerBase::escapePrefix($type['value'], $context['namespaces']); |
||||
} |
||||
} |
||||
} |
||||
} |
||||
} |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function buildConfigurationForm(array $form, FormStateInterface $form_state) { |
||||
$options = []; |
||||
$fieldsArray = \Drupal::service('entity_field.manager')->getFieldMap(); |
||||
foreach ($fieldsArray as $entity_type => $entity_fields) { |
||||
foreach ($entity_fields as $field => $field_properties) { |
||||
$options[$field] = $this->t('@field (@bundles)', [ |
||||
'@field' => $field, |
||||
'@bundles' => implode(', ', array_keys($field_properties['bundles'])), |
||||
]); |
||||
} |
||||
} |
||||
|
||||
$config = $this->getConfiguration(); |
||||
$form['source_field'] = [ |
||||
'#type' => 'select', |
||||
'#title' => $this->t('Source Field'), |
||||
'#options' => $options, |
||||
'#description' => $this->t("Select the field containing the type predicates."), |
||||
'#default_value' => isset($config['source_field']) ? $config['source_field'] : '', |
||||
]; |
||||
return $form; |
||||
} |
||||
|
||||
/** |
||||
* {@inheritdoc} |
||||
*/ |
||||
public function submitConfigurationForm(array &$form, FormStateInterface $form_state) { |
||||
$this->setConfiguration(['source_field' => $form_state->getValue('source_field')]); |
||||
} |
||||
|
||||
} |
Loading…
Reference in new issue