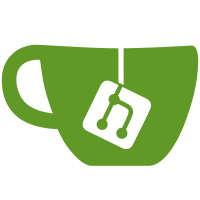
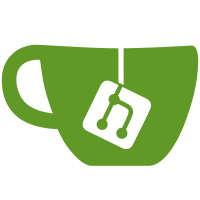
7 changed files with 267 additions and 6 deletions
@ -0,0 +1,61 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Admin form. |
||||
*/ |
||||
|
||||
/** |
||||
* Form function for module config. |
||||
* |
||||
* @param array $form |
||||
* Drupal renderable array. |
||||
* @param array $form_state |
||||
* Drupal form state. |
||||
* |
||||
* @return array |
||||
* Final form to render. |
||||
*/ |
||||
function islandora_config_form(array $form, array &$form_state) { |
||||
$form = array( |
||||
'islandora_stomp_url' => array( |
||||
'#type' => 'textfield', |
||||
'#title' => t('Stomp URL'), |
||||
'#default_value' => variable_get('islandora_stomp_url', 'tcp://localhost:61613'), |
||||
'#description' => t('URL to the Stomp broker for async messaging'), |
||||
'#element_validate' => array('islandora_config_form_validate_stomp_url'), |
||||
'#required' => TRUE, |
||||
), |
||||
'islandora_triplestore_index_queue' => array( |
||||
'#type' => 'textfield', |
||||
'#title' => t('Triplestore Index Queue'), |
||||
'#default_value' => variable_get('islandora_triplestore_index_queue', 'islandora/triplestore/index'), |
||||
'#description' => t('Queue to send triplestore indexing messages'), |
||||
'#required' => TRUE, |
||||
), |
||||
); |
||||
|
||||
return system_settings_form($form); |
||||
} |
||||
|
||||
/** |
||||
* Stomp broker url validator. |
||||
* |
||||
* @param array $element |
||||
* Form element for stomp broker url. |
||||
* @param array $form_state |
||||
* Drupal form state. |
||||
* @param array $form |
||||
* Drupal renderable array. |
||||
*/ |
||||
function islandora_config_form_validate_stomp_url(array $element, array &$form_state, array $form) { |
||||
// Try to connect to the server. |
||||
try { |
||||
$url = $element['#value']; |
||||
$stomp = new Stomp($url); |
||||
unset($stomp); |
||||
} |
||||
catch (StompException $e) { |
||||
form_error($element, t('Connection failed for @url: @message', array('@url' => $url, '@message' => $e->getMessage()))); |
||||
} |
||||
} |
@ -0,0 +1,21 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Utility functions for working with RDF. |
||||
*/ |
||||
|
||||
/** |
||||
* Serializes a Drupal node to N-Triples. |
||||
* |
||||
* @param object $node |
||||
* Node to serialize. |
||||
* |
||||
* @return string |
||||
* RDF serialized as N-Triples. |
||||
*/ |
||||
function islandora_serialize_node_to_triples($node) { |
||||
$rdf_model = rdfx_get_rdf_model('node', $node); |
||||
$serializer = ARC2::getSer('NTriples', array('ns' => $rdf_model->ns)); |
||||
return $serializer->getSerializedIndex($rdf_model->index); |
||||
} |
@ -0,0 +1,70 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Triplestore indexing functionality. |
||||
*/ |
||||
|
||||
/** |
||||
* Enqueues a triplestore upsert action. |
||||
* |
||||
* @param object $node |
||||
* Node to upsert in index. |
||||
* |
||||
* @return bool |
||||
* True if publishing the message was successful. |
||||
*/ |
||||
function islandora_triplestore_upsert($node) { |
||||
return islandora_triplestore_enqueue_action($node, 'upsert'); |
||||
} |
||||
|
||||
/** |
||||
* Enqueues a triplestore delete action. |
||||
* |
||||
* @param object $node |
||||
* Node to delete from index. |
||||
* |
||||
* @return bool |
||||
* True if publishing the message was successful. |
||||
*/ |
||||
function islandora_triplestore_delete($node) { |
||||
return islandora_triplestore_enqueue_action($node, 'delete'); |
||||
} |
||||
|
||||
/** |
||||
* Enqueues a triplestore index action. |
||||
* |
||||
* @param object $node |
||||
* Node to index. |
||||
* @param string $action |
||||
* Action to take. Either 'upsert' or 'delete' at the moment. |
||||
* |
||||
* @return bool |
||||
* True if publishing the message was successful. |
||||
*/ |
||||
function islandora_triplestore_enqueue_action($node, $action) { |
||||
module_load_include('inc', 'islandora', 'include/rdf'); |
||||
$broker_url = variable_get("islandora_stomp_url", "tcp://localhost:61613"); |
||||
$queue = variable_get("islandora_triplestore_index_queue", "islandora/triplestore/index"); |
||||
$msg = islandora_serialize_node_to_triples($node); |
||||
|
||||
// Add enough headers to fool the FcrepoCamel code we're re-using to do this. |
||||
global $base_url; |
||||
$headers = array( |
||||
'action' => $action, |
||||
'Content-Type' => 'application/n-triples', |
||||
'CamelFcrepoBaseUrl' => $base_url, |
||||
'CamelFcrepoIdentifier' => "/node/{$node->nid}", |
||||
); |
||||
|
||||
try { |
||||
$stomp = new Stomp($broker_url); |
||||
$stomp->send($queue, $msg, $headers); |
||||
unset($stomp); |
||||
return TRUE; |
||||
} |
||||
catch (StompException $e) { |
||||
watchdog('islandora', 'Error publishing triplestore index action to Stomp broker: @err', array('@err' => $e->getMessage()), WATCHDOG_ERROR); |
||||
return FALSE; |
||||
} |
||||
} |
@ -0,0 +1,24 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Miscellaneous utility functions for the islandora module. |
||||
*/ |
||||
|
||||
/** |
||||
* Predicate to determine if we should ignore hooks that talk to Fedora. |
||||
* |
||||
* @return bool |
||||
* True if we shouldn't talk to Fedora (breaks the cycle). |
||||
*/ |
||||
function islandora_ignore_hooks() { |
||||
$header_is_set = isset($_SERVER['HTTP_IGNORE_HOOKS']); |
||||
|
||||
if (!$header_is_set) { |
||||
return FALSE; |
||||
} |
||||
|
||||
$header_is_true = strcmp(strtolower($_SERVER['HTTP_IGNORE_HOOKS']), "true") == 0; |
||||
|
||||
return $header_is_true; |
||||
} |
Loading…
Reference in new issue