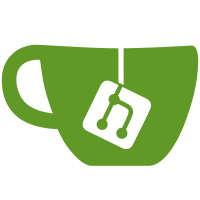
6 changed files with 0 additions and 2665 deletions
@ -1,9 +0,0 @@
|
||||
; $Id$ |
||||
name = Digital Repository |
||||
dependencies[] = imageapi |
||||
dependencies[] = tabs |
||||
dependencies[] = islandora_content_model_forms |
||||
description = Shows a list of items in a fedora collection. |
||||
package = Islandora |
||||
version = 11.2.beta1 |
||||
core = 6.x |
@ -1,107 +0,0 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file fedora_repository.install |
||||
*/ |
||||
|
||||
/** |
||||
* Implementation of hook_enable(). |
||||
*/ |
||||
function fedora_collections_enable() { |
||||
//nothing to do as we do not currently touch the drupal database. |
||||
//other than the variables table |
||||
} |
||||
|
||||
/** |
||||
* Implementation of hook_requirements(). |
||||
* |
||||
* @return |
||||
* An array describing the status of the site regarding available updates. |
||||
* If there is no update data, only one record will be returned, indicating |
||||
* that the status of core can't be determined. If data is available, there |
||||
* will be two records: one for core, and another for all of contrib |
||||
* (assuming there are any contributed modules or themes enabled on the |
||||
* site). In addition to the fields expected by hook_requirements ('value', |
||||
* 'severity', and optionally 'description'), this array will contain a |
||||
* 'reason' attribute, which is an integer constant to indicate why the |
||||
* given status is being returned (UPDATE_NOT_SECURE, UPDATE_NOT_CURRENT, or |
||||
* UPDATE_UNKNOWN). This is used for generating the appropriate e-mail |
||||
* notification messages during update_cron(), and might be useful for other |
||||
* modules that invoke update_requirements() to find out if the site is up |
||||
* to date or not. |
||||
* |
||||
* @see _update_message_text() |
||||
* @see _update_cron_notify() |
||||
*/ |
||||
function fedora_repository_requirements($phase) { |
||||
global $base_url; |
||||
|
||||
$requirements = array(); |
||||
|
||||
if ($phase == 'install') { |
||||
|
||||
// Test for SOAP |
||||
$requirements['fedora-soap']['title'] = t("PHP SOAP extension library"); |
||||
if (!class_exists('SoapClient')) { |
||||
$requirements['fedora-soap']['value'] = t("Not installed"); |
||||
$requirements['fedora-soap']['severity'] = REQUIREMENT_ERROR; |
||||
$requirements['fedora-soap']['description'] = t('Ensure that the PHP SOAP extension is installed.'); |
||||
} |
||||
else { |
||||
$requirements['fedora-soap']['value'] = t("Installed"); |
||||
$requirements['fedora-soap']['severity'] = REQUIREMENT_OK; |
||||
} |
||||
|
||||
// Test for Curl |
||||
$requirements['curl']['title'] = "PHP Curl extension library"; |
||||
if (!function_exists('curl_init')) { |
||||
$requirements['curl']['value'] = t("Not installed"); |
||||
$requirements['curl']['severity'] = REQUIREMENT_ERROR; |
||||
$requirements['curl']['description'] = t("Ensure that the PHP Curl extension is installed."); |
||||
} |
||||
else { |
||||
$requirements['curl']['value'] = t("Installed"); |
||||
$requirements['curl']['severity'] = REQUIREMENT_OK; |
||||
} |
||||
|
||||
// Test for DOM |
||||
$requirements['dom']['title'] = "PHP DOM XML extension library"; |
||||
if (!method_exists('DOMDocument', 'loadHTML')) { |
||||
$requirements['dom']['value'] = t("Not installed"); |
||||
$requirements['dom']['severity'] = REQUIREMENT_ERROR; |
||||
$requirements['dom']['description'] = t("Ensure that the PHP DOM XML extension is installed."); |
||||
} |
||||
else { |
||||
$requirements['dom']['value'] = t("Installed"); |
||||
$requirements['dom']['severity'] = REQUIREMENT_OK; |
||||
} |
||||
|
||||
// Test for XSLT |
||||
$requirements['xsl']['title'] = "PHP XSL extension library"; |
||||
if (!class_exists('XSLTProcessor')) { |
||||
$requirements['xslt']['value'] = t("Not installed"); |
||||
$requirements['xslt']['severity'] = REQUIREMENT_ERROR; |
||||
$requirements['xslt']['description'] = t("Ensure that the PHP XSL extension is installed."); |
||||
} |
||||
else { |
||||
$requirements['xslt']['value'] = t("Installed"); |
||||
$requirements['xslt']['severity'] = REQUIREMENT_OK; |
||||
} |
||||
} |
||||
elseif ($phase == 'runtime') { |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_utils'); |
||||
|
||||
$requirements['fedora-repository']['title'] = t("Fedora server"); |
||||
if (!fedora_available()) { |
||||
$requirements['fedora-repository']['value'] = t("Not available"); |
||||
$requirements['fedora-repository']['severity'] = REQUIREMENT_ERROR; |
||||
$requirements['fedora-repository']['description'] = t('Ensure that Fedora is running and that the <a href="@collection-settings">collection settings</a> are correct.', array('@collection-settings' => $base_url . '/admin/settings/fedora_repository')); |
||||
} |
||||
else { |
||||
$requirements['fedora-repository']['value'] = t("Available"); |
||||
$requirements['fedora-repository']['severity'] = REQUIREMENT_OK; |
||||
} |
||||
} |
||||
|
||||
return $requirements; |
||||
} |
@ -1,175 +0,0 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file |
||||
* Invokes a hook to any dependent modules asking them if their installations require |
||||
* any fedora objects to be present. Modules implementing this hook should return an array |
||||
* of arrays of the form: |
||||
* |
||||
* array( 'pid', 'path-to-foxml-file', 'dsid', 'path-to-datastream-file', int dsversion) |
||||
* |
||||
* where the last three options are optional. A module can either point to a simple |
||||
* foxml file to install, or can specify a datastreamstream to check for, with a |
||||
* path to load the datastream from if it isn't there. Optionally a version number |
||||
* can be included, to enable updating of content model or collection policy streams |
||||
* that may have been updated. THis is a simple whole number that should be incremented |
||||
* when changed. This value appears in as an attribute of the topmost element of the stream, |
||||
* e.g.,: |
||||
* |
||||
* <?xml version="1.0" encoding="utf-8"?> <content_model name="Collection" version="2" ...
|
||||
* |
||||
* Datastreams which don't have this element are assumed to be at version 0. |
||||
*/ |
||||
function fedora_repository_solution_packs_page() { |
||||
$enabled_solution_packs = module_invoke_all('required_fedora_objects'); |
||||
$output = ''; |
||||
foreach ($enabled_solution_packs as $solution_pack_module => $solution_pack_info) { |
||||
$objects = array(); |
||||
foreach ($solution_pack_info as $field => $value) { |
||||
switch ($field) { |
||||
case 'title': |
||||
$solution_pack_name = $value; |
||||
break; |
||||
case 'objects': |
||||
$objects = $value; |
||||
break; |
||||
} |
||||
} |
||||
$output .= drupal_get_form('fedora_repository_solution_pack_form_' . $solution_pack_module, $solution_pack_module, $solution_pack_name, $objects); |
||||
} |
||||
|
||||
return $output; |
||||
} |
||||
|
||||
/** |
||||
* Check for installed objects and add a 'Update' or 'Install' button if some objects are missing. |
||||
* @param array $solution_pack |
||||
*/ |
||||
function fedora_repository_solution_pack_form(&$form_state, $solution_pack_module, $solution_pack_name, $objects = array()) { |
||||
// Check each object to see if it is in the repository. |
||||
module_load_include('inc', 'fedora_repository', 'api/fedora_item'); |
||||
global $base_path; |
||||
$needs_update = FALSE; |
||||
$needs_install = FALSE; |
||||
$form = array(); |
||||
$form['solution_pack_module'] = array( |
||||
'#type' => 'hidden', |
||||
'#value' => $solution_pack_module, |
||||
); |
||||
|
||||
if (!$form_state['submitted']) { |
||||
$form['soluction_pack_name'] = array( |
||||
'#type' => 'markup', |
||||
'#value' => t($solution_pack_name), |
||||
'#prefix' => '<h3>', |
||||
'#suffix' => '</h3>', |
||||
); |
||||
$form['objects'] = array( |
||||
'#type' => 'fieldset', |
||||
'#title' => "Objects", |
||||
'#weight' => 10, |
||||
'#attributes' => array('class' => 'collapsed'), |
||||
'#collapsible' => TRUE, |
||||
'#collapsed' => TRUE, |
||||
); |
||||
$table_header = array('PID', 'Status'); |
||||
$table_rows = array(); |
||||
|
||||
|
||||
foreach ($objects as $object) { |
||||
$datastreams = NULL; |
||||
if (isset($object['pid'])) { |
||||
$pid = $object['pid']; |
||||
|
||||
$item = new Fedora_Item($pid); |
||||
$table_row = array($object['pid']); |
||||
$object_status = t('Up-to-date'); |
||||
if (!$item->exists()) { |
||||
$object_status = 'Missing'; |
||||
$needs_install = TRUE; |
||||
} |
||||
else { |
||||
if (isset($object['dsid']) && isset($object['datastream_file']) && isset($object['dsversion'])) { |
||||
$datastreams = array( |
||||
array( |
||||
'dsid' => $object['dsid'], |
||||
'datastream_file' => $object['datastream_file'], |
||||
'dsversion' => $object['dsversion'], |
||||
), |
||||
); |
||||
} |
||||
elseif (!empty($object['datastreams'])) { |
||||
$datastreams = $object['datastreams']; |
||||
} |
||||
if (!empty($datastreams) && is_array($datastreams)) { |
||||
foreach ($datastreams as $ds) { |
||||
$ds_list = $item->get_datastreams_list_as_array(); |
||||
if (!array_key_exists($ds['dsid'], $ds_list)) { |
||||
$needs_update = TRUE; |
||||
$object_status = 'Missing datastream'; |
||||
break; |
||||
} |
||||
if (isset($ds['dsversion'])) { |
||||
// Check if the datastream is versioned and needs updating. |
||||
$installed_version = fedora_repository_get_islandora_datastream_version($item, $ds['dsid']); |
||||
$available_version = fedora_repository_get_islandora_datastream_version(NULL, NULL, $ds['datastream_file']); |
||||
if ($available_version > $installed_version) { |
||||
$needs_update = TRUE; |
||||
$object_status = 'Out of date'; |
||||
break; |
||||
} |
||||
} |
||||
} |
||||
} |
||||
} |
||||
array_push($table_row, $object_status); |
||||
$table_rows[] = $table_row; |
||||
} |
||||
} |
||||
$form['objects']['table'] = array( |
||||
'#type' => 'markup', |
||||
'#value' => theme_table($table_header, $table_rows), |
||||
); |
||||
} |
||||
|
||||
$form['install_status'] = array( |
||||
'#type' => 'markup', |
||||
'#prefix' => '<strong>' . t('Object status:') . ' </strong>', |
||||
'#suffix' => ' ', |
||||
); |
||||
if (!$needs_install && !$needs_update) { |
||||
$form['install_status']['#value'] = theme_image('misc/watchdog-ok.png') . t('All required objects are installed and up-to-date.'); |
||||
} |
||||
else { |
||||
$form['install_status']['#value'] = theme_image('misc/watchdog-warning.png') . t('Some objects must be re-ingested. See Objects list for details.'); |
||||
} |
||||
$form['submit'] = array( |
||||
'#value' => t('Install'), |
||||
'#disabled' => !$needs_install && !$needs_update, |
||||
'#type' => 'submit', |
||||
'#name' => $solution_pack_module, |
||||
); |
||||
|
||||
$form['#submit'] = array( |
||||
'fedora_repository_solution_pack_form_submit', |
||||
); |
||||
return $form; |
||||
} |
||||
|
||||
function fedora_repository_solution_pack_form_submit($form, &$form_state) { |
||||
$what = $form_state; |
||||
$module_name = $form_state['values']['solution_pack_module']; |
||||
$solution_pack_info = call_user_func($module_name . '_required_fedora_objects'); |
||||
$batch = array( |
||||
'title' => t('Installing / updating solution pack objects'), |
||||
'file' => drupal_get_path('module', 'fedora_repository') . '/fedora_repository.module', |
||||
'operations' => array(), |
||||
); |
||||
|
||||
|
||||
foreach ($solution_pack_info[$module_name]['objects'] as $object) { |
||||
// Add this object to the batch job queue. |
||||
$batch['operations'][] = array('fedora_repository_batch_reingest_object', array($object)); |
||||
} |
||||
batch_set($batch); |
||||
} |
@ -1,7 +0,0 @@
|
||||
name = Fedora ImageAPI |
||||
description = Adds image manipulation support through a REST interface |
||||
package = Islandora Dependencies |
||||
dependencies[] = fedora_repository |
||||
dependencies[] = imageapi |
||||
version = 11.2.beta1 |
||||
core = 6.x |
@ -1,116 +0,0 @@
|
||||
<?php |
||||
|
||||
|
||||
|
||||
function fedora_imageapi_menu() { |
||||
$items = array(); |
||||
$items['fedora/imageapi'] = array( |
||||
'title' => t('Image manipulation functions'), |
||||
'page callback' => 'fedora_repository_image_manip', |
||||
'type' => MENU_CALLBACK, |
||||
'access arguments' => array('view fedora collection'), |
||||
); |
||||
return $items; |
||||
} |
||||
|
||||
/** |
||||
* Call out to the Drupal ImageAPI module and return the resulting image as a stream. |
||||
* |
||||
* @param string $pid |
||||
* @param string $dsid |
||||
* @param string $op |
||||
* @param string $params |
||||
*/ |
||||
function fedora_repository_image_manip($pid = '', $dsid = '', $op = '', $params = '') { |
||||
module_load_include('inc', 'Fedora_Repository', 'ObjectHelper'); |
||||
module_load_include('module', 'imageapi'); |
||||
$obj = new ObjectHelper(); |
||||
$mimetype = $obj->getMimeType($pid, $dsid); |
||||
$ext = substr(strstr($mimetype, '/'), 1); |
||||
$op = (!empty($_GET['op']) ? $_GET['op'] : ''); |
||||
$safe_pid = str_replace(':', '_', $pid); |
||||
|
||||
$cache_key = 'fedora_repository_image_manip_' . md5($safe_pid . '_' . $dsid . '_' . $ext . '_' . $op . (isset($_GET['width']) ? '_' . $_GET['width'] : '') . (isset($_GET['height']) ? '_' . $_GET['height'] : '')); |
||||
if (($file = cache_get($cache_key)) === 0) { |
||||
//added the slash as sys_get_temp_dir in linux does not seem to include the slash |
||||
$tmp_file_name = sys_get_temp_dir() . '/' . $safe_pid . '_' . $dsid . '.' . $ext; |
||||
$handle = fopen($tmp_file_name, "w"); |
||||
$numbytes = fwrite($handle, $obj->getStream($pid, $dsid)); |
||||
fclose($handle); |
||||
if ($numbytes == 0) { |
||||
return; |
||||
} |
||||
|
||||
|
||||
$image = imageapi_image_open($tmp_file_name); |
||||
|
||||
switch ($op) { |
||||
case 'scale': |
||||
if (!empty($_GET['height']) || !empty($_GET['width'])) { |
||||
imageapi_image_scale($image, $_GET['width'], $_GET['height']); |
||||
} |
||||
case 'centerscale': |
||||
if (!empty($_GET['height']) && !empty($_GET['width'])) { |
||||
imageapi_image_scale_and_crop($image, $_GET['width'], $_GET['height']); |
||||
} |
||||
} |
||||
imageapi_image_close($image); |
||||
$file = file_get_contents($tmp_file_name); |
||||
cache_set($cache_key, $file, 'cache', time() + variable_get('fedora_image_blocks_cache_time', 3600)); |
||||
file_delete($tmp_file_name); |
||||
} |
||||
else { |
||||
$file = $file->data; |
||||
} |
||||
|
||||
|
||||
header("Content-type: $mimetype"); |
||||
header('Content-Disposition: attachment; filename="' . $dsid . '.' . $ext . '"'); |
||||
echo $file; |
||||
|
||||
|
||||
// return "$numbytes bytes written to ".sys_get_temp_dir()."$pid_$dsid.$ext\n"; |
||||
} |
||||
|
||||
/** |
||||
* Implementation of hook_form_alter |
||||
* |
||||
* @param unknown_type $form |
||||
* @param unknown_type $form_state |
||||
* @param unknown_type $form_id |
||||
*/ |
||||
/* |
||||
function fedora_imageapi_form_alter( &$form, $form_state, $form_id) { |
||||
|
||||
switch ( $form_id ) { |
||||
case 'fedora_repository_admin': |
||||
|
||||
$fedora_base_url = $form['fedora_base_url']['#default_value']; |
||||
|
||||
$fedora_server_url = substr($fedora_base_url,0,strpos($fedora_base_url,'/',7)); |
||||
// Add the Djatoka server location. Set it to default to the same server as fedora. |
||||
$form['djatoka_server_url'] = array ( |
||||
'#type' => 'textfield', |
||||
'#title' => '<h3>'.t('Fedora Image API Module').'</h3><br />'.t('aDORe Djatoka image server resolver URL'), |
||||
'#default_value' => variable_get('djatoka_server_url', $fedora_server_url.'/adore-djatoka/resolver' ), |
||||
'#description' => t('The location of your <a href="http://african.lanl.gov/aDORe/projects/djatoka/" title="aDORe Djatoka Home Page">aDORe Djatoka</a> image server, if you have one installed.'), |
||||
'#weight' => 1, |
||||
); |
||||
$form['openlayers_server_url'] = array( |
||||
'#type' => 'textfield', |
||||
'#title' => t('OpenLayers servlet URL'), |
||||
'#default_value' => variable_get('openlayers_server_url', $fedora_server_url.'/islandora/OpenLayers'), |
||||
'#description' => t('URL of your installation of the <a href="http://openlayers.org/">OpenLayers</a> servlet, if you have one.'), |
||||
'#weight' => 1, |
||||
); |
||||
$form['buttons']['#weight'] = 2; |
||||
break; |
||||
} |
||||
} |
||||
|
||||
function show_openlayers_viewer() { |
||||
$output = 'Hi.'; |
||||
|
||||
return $output; |
||||
} |
||||
*/ |
Loading…
Reference in new issue