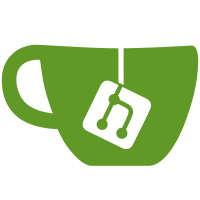
4 changed files with 221 additions and 0 deletions
After Width: | Height: | Size: 5.0 KiB |
@ -0,0 +1,95 @@
|
||||
<?php |
||||
|
||||
/** |
||||
* @file islandora_basic_collection.install |
||||
*/ |
||||
|
||||
function islandora_basic_collection_install() { |
||||
module_load_include('inc', 'islandora', 'RestConnection'); |
||||
global $user; |
||||
global $base_root; |
||||
|
||||
try { |
||||
$restConnection = new RestConnection($user); |
||||
} catch (Exception $e) { |
||||
drupal_set_message(t('Unable to connect to the repository %e', array('%e' => $e)), 'error'); |
||||
return; |
||||
} |
||||
|
||||
$content_model_query = $restConnection->api->a->findObjects('query', 'pid=islandora:collectionCModel'); |
||||
if (empty($content_model_query['results'])) { |
||||
try { |
||||
$xml = file_get_contents(drupal_get_path('module', 'islandora_basic_collection') . '/xml/islandora_collection_CModel.xml'); |
||||
$restConnection->api->m->ingest(array('string' => $xml)); |
||||
} catch (Exception $e) { |
||||
drupal_set_message(t('Unable to install content models %e', array('%e' => $e)), 'error'); |
||||
return; |
||||
} |
||||
drupal_set_message(t('Content models installed!')); |
||||
} |
||||
else { |
||||
drupal_set_message(t('Content models already exist!'), 'warning'); |
||||
} |
||||
|
||||
$collection_query = $restConnection->api->a->findObjects('query', 'pid=islandora:root'); |
||||
if (empty($collection_query['results'])) { |
||||
try { |
||||
$xml = file_get_contents(drupal_get_path('module', 'islandora_basic_collection') . '/xml/islandora_root_collection.xml'); |
||||
$restConnection->api->m->ingest(array('string' => $xml)); |
||||
$fedora_object = new FedoraObject('islandora:root', $restConnection->repository); |
||||
$datastream = new NewFedoraDatastream('TN', 'M', $fedora_object, $restConnection->repository); |
||||
$file_path = $base_root . '/' . drupal_get_path('module', 'islandora_basic_collection') . '/Crystal_Clear_filesystem_folder_grey.png'; |
||||
$datastream->label = 'Thumbnail'; |
||||
$datastream->mimetype = 'image/png'; |
||||
$datastream->setContentFromUrl($file_path); |
||||
$fedora_object->ingestDatastream($datastream); |
||||
|
||||
} catch (Exception $e) { |
||||
drupal_set_message(t('Unable to install collections %e', array('%e' => $e)), 'error'); |
||||
return; |
||||
} |
||||
drupal_set_message(t('Collections installed!')); |
||||
} |
||||
else { |
||||
drupal_set_message(t('Collections already exist!'), 'warning'); |
||||
} |
||||
} |
||||
|
||||
function islandora_basic_collection_uninstall() { |
||||
module_load_include('inc', 'islandora', 'RestConnection'); |
||||
global $user; |
||||
try { |
||||
$restConnection = new RestConnection($user); |
||||
} catch (Exception $e) { |
||||
drupal_set_message(t('Unable to connect to the repository %e', array('%e' => $e)), 'error'); |
||||
return; |
||||
} |
||||
|
||||
$content_model_query = $restConnection->api->a->findObjects('query', 'pid=islandora:collectionCModel'); |
||||
if (!empty($content_model_query['results'])) { |
||||
try { |
||||
$restConnection->repository->purgeObject('islandora:collectionCModel'); |
||||
} catch (Exception $e) { |
||||
drupal_set_message(t('Unable to purge content models %e', array('%e' => $e)), 'error'); |
||||
return; |
||||
} |
||||
drupal_set_message(t('Content models purged!')); |
||||
} |
||||
else { |
||||
drupal_set_message(t('Content models don\'t exist!'), 'warning'); |
||||
} |
||||
|
||||
$collection_query = $restConnection->api->a->findObjects('query', 'pid=islandora:root'); |
||||
if (!empty($collection_query['results'])) { |
||||
try { |
||||
$restConnection->repository->purgeObject('islandora:root'); |
||||
} catch (Exception $e) { |
||||
drupal_set_message(t('Unable to purge collections %e', array('%e' => $e)), 'error'); |
||||
return; |
||||
} |
||||
drupal_set_message(t('Collections purged!')); |
||||
} |
||||
else { |
||||
drupal_set_message(t('Collections don\'t exist!'), 'warning'); |
||||
} |
||||
} |
@ -0,0 +1,58 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?> |
||||
<foxml:digitalObject VERSION="1.1" PID="islandora:collectionCModel" |
||||
xmlns:foxml="info:fedora/fedora-system:def/foxml#" |
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" |
||||
xsi:schemaLocation="info:fedora/fedora-system:def/foxml# http://www.fedora.info/definitions/1/0/foxml1-1.xsd"> |
||||
<foxml:objectProperties> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/model#state" VALUE="Active"/> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/model#label" VALUE="Islandora Collection Content Model"/> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/model#ownerId" VALUE="fedoraAdmin"/> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/model#createdDate" VALUE="2012-05-14T13:13:59.507Z"/> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/view#lastModifiedDate" VALUE="2012-05-14T13:13:59.964Z"/> |
||||
</foxml:objectProperties> |
||||
<foxml:datastream ID="DC" STATE="A" CONTROL_GROUP="X" VERSIONABLE="true"> |
||||
<foxml:datastreamVersion ID="DC1.0" LABEL="Dublin Core Record for this object" CREATED="2012-05-14T13:13:59.507Z" MIMETYPE="text/xml" FORMAT_URI="http://www.openarchives.org/OAI/2.0/oai_dc/" SIZE="413"> |
||||
<foxml:xmlContent> |
||||
<oai_dc:dc xmlns:oai_dc="http://www.openarchives.org/OAI/2.0/oai_dc/" xmlns:dc="http://purl.org/dc/elements/1.1/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.openarchives.org/OAI/2.0/oai_dc/ http://www.openarchives.org/OAI/2.0/oai_dc.xsd"> |
||||
<dc:title>Islandora Collection Content Model</dc:title> |
||||
<dc:identifier>islandora:collectionCModel</dc:identifier> |
||||
</oai_dc:dc> |
||||
</foxml:xmlContent> |
||||
</foxml:datastreamVersion> |
||||
</foxml:datastream> |
||||
<foxml:datastream ID="DS-COMPOSITE-MODEL" STATE="A" CONTROL_GROUP="X" VERSIONABLE="false"> |
||||
<foxml:datastreamVersion ID="DS-COMPOSITE-MODEL1.0" LABEL="Datastream Composite Model" CREATED="2012-04-26T13:37:58.308Z" MIMETYPE="text/xml" FORMAT_URI="info:fedora/fedora-system:FedoraDSCompositeModel-1.0"> |
||||
<foxml:xmlContent> |
||||
<dsCompositeModel xmlns="info:fedora/fedora-system:def/dsCompositeModel#"> <!-- using this as a guide --> |
||||
<dsTypeModel ID="DC" > |
||||
<form FORMAT_URI="http://www.openarchives.org/OAI/2.0/oai_dc/" MIME="text/xml"></form> |
||||
</dsTypeModel> |
||||
<dsTypeModel ID="RELS-EXT" optional="true"> |
||||
<form FORMAT_URI="info:fedora/fedora-system:FedoraRELSExt-1.0" MIME="application/rdf+xml"></form> |
||||
</dsTypeModel> |
||||
<dsTypeModel ID="RELS-INT" optional="true"> |
||||
<form FORMAT_URI="info:fedora/fedora-system:FedoraRELSInt-1.0" MIME="application/rdf+xml"></form> |
||||
</dsTypeModel> |
||||
</dsCompositeModel> |
||||
</foxml:xmlContent> |
||||
</foxml:datastreamVersion> |
||||
</foxml:datastream> |
||||
<foxml:datastream ID="RELS-EXT" STATE="A" CONTROL_GROUP="X" VERSIONABLE="true"> |
||||
<foxml:datastreamVersion ID="RELS-EXT.0" LABEL="Fedora object-to-object relationship metadata" CREATED="2012-05-14T13:13:59.665Z" MIMETYPE="application/rdf+xml" SIZE="167"> |
||||
<foxml:xmlContent> |
||||
<rdf:RDF xmlns:rdf="http://www.w3.org/1999/02/22-rdf-syntax-ns#"> |
||||
<rdf:Description rdf:about="info:fedora/islandora:collectionCModel"></rdf:Description> |
||||
</rdf:RDF> |
||||
</foxml:xmlContent> |
||||
</foxml:datastreamVersion> |
||||
<foxml:datastreamVersion ID="RELS-EXT.1" LABEL="Fedora Object-to-Object Relationship Metadata" CREATED="2012-05-14T13:13:59.879Z" MIMETYPE="application/rdf+xml" SIZE="299"> |
||||
<foxml:xmlContent> |
||||
<rdf:RDF xmlns:rdf="http://www.w3.org/1999/02/22-rdf-syntax-ns#"> |
||||
<rdf:Description rdf:about="info:fedora/islandora:collectionCModel"> |
||||
<hasModel xmlns="info:fedora/fedora-system:def/model#" rdf:resource="info:fedora/fedora-system:ContentModel-3.0"></hasModel> |
||||
</rdf:Description> |
||||
</rdf:RDF> |
||||
</foxml:xmlContent> |
||||
</foxml:datastreamVersion> |
||||
</foxml:datastream> |
||||
</foxml:digitalObject> |
@ -0,0 +1,68 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?> |
||||
<foxml:digitalObject VERSION="1.1" PID="islandora:root" |
||||
xmlns:foxml="info:fedora/fedora-system:def/foxml#" |
||||
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" |
||||
xsi:schemaLocation="info:fedora/fedora-system:def/foxml# http://www.fedora.info/definitions/1/0/foxml1-1.xsd"> |
||||
<foxml:objectProperties> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/model#state" VALUE="Active"/> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/model#label" VALUE="Islandora Top-level Collection"/> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/model#ownerId" VALUE="fedoraAdmin"/> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/model#createdDate" VALUE="2012-05-14T13:14:01.118Z"/> |
||||
<foxml:property NAME="info:fedora/fedora-system:def/view#lastModifiedDate" VALUE="2012-05-14T13:14:01.769Z"/> |
||||
</foxml:objectProperties> |
||||
<foxml:datastream ID="DC" STATE="A" CONTROL_GROUP="X" VERSIONABLE="true"> |
||||
<foxml:datastreamVersion ID="DC1.0" LABEL="Dublin Core Record for this object" CREATED="2012-05-14T13:14:01.118Z" MIMETYPE="text/xml" FORMAT_URI="http://www.openarchives.org/OAI/2.0/oai_dc/" SIZE="397"> |
||||
<foxml:xmlContent> |
||||
<oai_dc:dc xmlns:oai_dc="http://www.openarchives.org/OAI/2.0/oai_dc/" xmlns:dc="http://purl.org/dc/elements/1.1/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.openarchives.org/OAI/2.0/oai_dc/ http://www.openarchives.org/OAI/2.0/oai_dc.xsd"> |
||||
<dc:title>Islandora Top-level Collection</dc:title> |
||||
<dc:identifier>islandora:root</dc:identifier> |
||||
</oai_dc:dc> |
||||
</foxml:xmlContent> |
||||
</foxml:datastreamVersion> |
||||
</foxml:datastream> |
||||
<foxml:datastream ID="RELS-EXT" STATE="A" CONTROL_GROUP="X" VERSIONABLE="true"> |
||||
<foxml:datastreamVersion ID="RELS-EXT.0" LABEL="Fedora object-to-object relationship metadata" CREATED="2012-05-14T13:14:01.258Z" MIMETYPE="application/rdf+xml" SIZE="155"> |
||||
<foxml:xmlContent> |
||||
<rdf:RDF xmlns:rdf="http://www.w3.org/1999/02/22-rdf-syntax-ns#"> |
||||
<rdf:Description rdf:about="info:fedora/islandora:root"></rdf:Description> |
||||
</rdf:RDF> |
||||
</foxml:xmlContent> |
||||
</foxml:datastreamVersion> |
||||
<foxml:datastreamVersion ID="RELS-EXT.1" LABEL="Fedora Object-to-Object Relationship Metadata" CREATED="2012-05-14T13:14:01.471Z" MIMETYPE="application/rdf+xml" SIZE="283"> |
||||
<foxml:xmlContent> |
||||
<rdf:RDF xmlns:rdf="http://www.w3.org/1999/02/22-rdf-syntax-ns#"> |
||||
<rdf:Description rdf:about="info:fedora/islandora:root"> |
||||
<hasModel xmlns="info:fedora/fedora-system:def/model#" rdf:resource="info:fedora/islandora:collectionCModel"></hasModel> |
||||
</rdf:Description> |
||||
</rdf:RDF> |
||||
</foxml:xmlContent> |
||||
</foxml:datastreamVersion> |
||||
</foxml:datastream> |
||||
<foxml:datastream ID="COLLECTION_POLICY" STATE="A" CONTROL_GROUP="X" VERSIONABLE="true"> |
||||
<foxml:datastreamVersion ID="COLLECTION_POLICY.0" LABEL="COLLECTION_POLICY" CREATED="2012-05-14T13:14:01.607Z" MIMETYPE="text/xml" SIZE="1211"> |
||||
<foxml:xmlContent> |
||||
<collection_policy name="" xmlns="http://www.islandora.ca" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.islandora.ca http://syn.lib.umanitoba.ca/collection_policy.xsd"> |
||||
<content_models> |
||||
<content_model dsid="ISLANDORACM" name="Collection" namespace="islandora:collection" pid="islandora:collectionCModel"/> |
||||
</content_models> |
||||
<search_terms> |
||||
<term field="dc.title">dc.title</term> |
||||
<term field="dc.creator">dc.creator</term> |
||||
<term default="true" field="dc.description">dc.description</term> |
||||
<term field="dc.date">dc.date</term> |
||||
<term field="dc.identifier">dc.identifier</term> |
||||
<term field="dc.language">dc.language</term> |
||||
<term field="dc.publisher">dc.publisher</term> |
||||
<term field="dc.rights">dc.rights</term> |
||||
<term field="dc.subject">dc.subject</term> |
||||
<term field="dc.relation">dc.relation</term> |
||||
<term field="dcterms.temporal">dcterms.temporal</term> |
||||
<term field="dcterms.spatial">dcterms.spatial</term> |
||||
<term field="fgs.DS.first.text">Full Text</term> |
||||
</search_terms> |
||||
<relationship>isMemberOfCollection</relationship> |
||||
</collection_policy> |
||||
</foxml:xmlContent> |
||||
</foxml:datastreamVersion> |
||||
</foxml:datastream> |
||||
</foxml:digitalObject> |
Loading…
Reference in new issue